UWP - Add a Hyperlink to a TextBlock
The TextBlock is the primary control for displaying read-only text in
UWP apps. The UWP app developers can use it to display single-line or
multi-line text, inline hyperlinks, and text with formatting like bold,
italic, or underlined. The TextBlock is designed to display a single paragraph
and it does not support text indentation.
The following Universal Windows Platform application development tutorial demonstrates how we can add a Hyperlink to a TextBlock. Here we will display a Hyperlink inside two TextBlock texts. That means we will put a Hyperlink within the TextBlock text.
For the first TextBlock control, we will add a Hyperlink inside the text using the XAML language. And for the second TextBlock control, we will put a Hyperlink within its text programmatically.
For the first TextBlock control, we will use inline Run and Hyperlink elements to add a Hyperlink in the text. And for the second TextBlock control, we will create and apply the Run and Hyperlink elements programmatically.
The Run class represents a discrete section of formatted or unformatted text. The UWP developers can use a Run instance in a TextBlock or RichTextBlock. The UWP app developers can even place multiple Run elements inside a Span. The UWP developers should typically use the Run element only when they want to format a discrete section of text within the TextBlock.
The Run class Text property gets or sets the text contents of the Run.
The Hyperlink class provides an inline-level content element that provides facilities for hosting hyperlinks.
The Hyperlink class NavigateUri property gets or sets the Uniform Resource Identifier (URI) to navigate when the Hyperlink is activated. This property value is a Uri which is the Uniform Resource Identifier (URI) to navigate to when the Hyperlink is activated. The default value is null. By default, a Hyperlink appears as a text hyperlink.
The Hyperlink class Inlines property gets an InlineCollection containing the top-level inline elements that include the contents of Span. This property is inherited from Span.
The TextBlock class Inlines property gets the collection of inline text elements within a TextBlock. This property value is an InlineCollectionWhich is a collection that holds all inline text elements from the TextBlock. The default value of this property is an empty collection.
The following Universal Windows Platform application development tutorial demonstrates how we can add a Hyperlink to a TextBlock. Here we will display a Hyperlink inside two TextBlock texts. That means we will put a Hyperlink within the TextBlock text.
For the first TextBlock control, we will add a Hyperlink inside the text using the XAML language. And for the second TextBlock control, we will put a Hyperlink within its text programmatically.
For the first TextBlock control, we will use inline Run and Hyperlink elements to add a Hyperlink in the text. And for the second TextBlock control, we will create and apply the Run and Hyperlink elements programmatically.
The Run class represents a discrete section of formatted or unformatted text. The UWP developers can use a Run instance in a TextBlock or RichTextBlock. The UWP app developers can even place multiple Run elements inside a Span. The UWP developers should typically use the Run element only when they want to format a discrete section of text within the TextBlock.
The Run class Text property gets or sets the text contents of the Run.
The Hyperlink class provides an inline-level content element that provides facilities for hosting hyperlinks.
The Hyperlink class NavigateUri property gets or sets the Uniform Resource Identifier (URI) to navigate when the Hyperlink is activated. This property value is a Uri which is the Uniform Resource Identifier (URI) to navigate to when the Hyperlink is activated. The default value is null. By default, a Hyperlink appears as a text hyperlink.
The Hyperlink class Inlines property gets an InlineCollection containing the top-level inline elements that include the contents of Span. This property is inherited from Span.
The TextBlock class Inlines property gets the collection of inline text elements within a TextBlock. This property value is an InlineCollectionWhich is a collection that holds all inline text elements from the TextBlock. The default value of this property is an empty collection.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Padding="50" Background="AliceBlue">
<TextBlock
x:Name="TextBlock1"
TextWrapping="Wrap"
Margin="5"
FontSize="30"
>
<Run>This is a sample TextBlock which diaplay a clickable Hyperlink,</Run>
<Hyperlink
NavigateUri="https://asp-net-example.blogspot.com/"
>
.NET C# Examples Blog
</Hyperlink>
<Run>is my personal blog.</Run>
</TextBlock>
<TextBlock
x:Name="TextBlock2"
FontSize="30"
Foreground="BlueViolet"
TextWrapping="Wrap"
Margin="5"
FontFamily="MV Boli"
/>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Documents;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
UpdateSecondTextBlock();
}
void UpdateSecondTextBlock() {
// Initialize a new Run instance
Run run = new Run();
run.Text = "Programmatically put a Hyperlink on TextBlock. ";
// Initialize a new Run instance for Hyperlink text
Run run2 = new Run();
run2.Text = "Google";
// Initialize another Run instance
Run run3 = new Run();
run3.Text = " is my favorite search engine.";
// Initialize a new Hyperlink instance
Hyperlink hyperlink = new Hyperlink();
// Specify the Hyperlink navigate Uri
hyperlink.NavigateUri=new System.Uri("https://www.google.com");
// Specify the Hyperlink text
hyperlink.Inlines.Add(run2);
// Clear the TextBlock
TextBlock2.Inlines.Clear();
// Add the text to the TextBlock
TextBlock2.Inlines.Add(run);
// Programmatically put a clickable Hyperlink on TextBlock
TextBlock2.Inlines.Add(hyperlink);
// Add the text to the TextBlock
TextBlock2.Inlines.Add(run3);
}
}
}
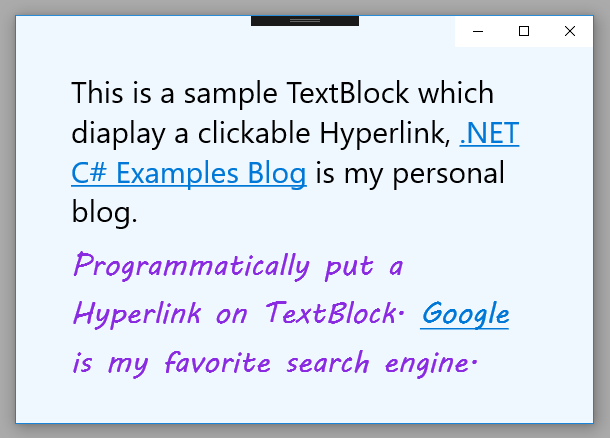