UWP - Change TextBox background color
The TextBox class represents a control that can be used to display and
edit plain text. A TextBox can be single or multi-line. The TextBox control
enables the UWP app user to enter text into a UWP app. The TextBox is
typically used to capture a single line of text but the UWP developers can
configure it to capture multiple lines of text. The text displays on the
screen in a simple uniform plaintext format.
The following Universal Windows Platform application development tutorial demonstrates how we can set or change the TextBox background color. Here we will wrap the TextBox control with a Border control. Then We will set the Border control’s background color. This color will be displayed as the TextBox control’s background color. We will also set the TextBox background color programmatically when it gets focused.
The Border class draws a border, background, or both, around another object. The Border class Background property gets or sets the Brush that fills the background of the border which means the inner area of the border.
The Border is a container control that draws a border, background, or both, around another object. So we can draw a background color to a TextBox control by wrapping the TextBox control with a Border and setting a background color to this Border control.
The TextBox GotFocus event occurs when the TextBox UIElement receives focus. The GotFocus event is raised asynchronously, so the focus can move again before bubbling is complete. The TextBox GotFocus event is inherited from UIElement.
On the TextBox GotFocus event, we will set a background color for the TextBox. The TextBox Background property gets or sets a brush that provides the background of the control. Using this property we will set a background color for the TextBox control when it gets focused.
The following Universal Windows Platform application development tutorial demonstrates how we can set or change the TextBox background color. Here we will wrap the TextBox control with a Border control. Then We will set the Border control’s background color. This color will be displayed as the TextBox control’s background color. We will also set the TextBox background color programmatically when it gets focused.
The Border class draws a border, background, or both, around another object. The Border class Background property gets or sets the Brush that fills the background of the border which means the inner area of the border.
The Border is a container control that draws a border, background, or both, around another object. So we can draw a background color to a TextBox control by wrapping the TextBox control with a Border and setting a background color to this Border control.
The TextBox GotFocus event occurs when the TextBox UIElement receives focus. The GotFocus event is raised asynchronously, so the focus can move again before bubbling is complete. The TextBox GotFocus event is inherited from UIElement.
On the TextBox GotFocus event, we will set a background color for the TextBox. The TextBox Background property gets or sets a brush that provides the background of the control. Using this property we will set a background color for the TextBox control when it gets focused.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Padding="50" Background="AliceBlue">
<!--
Put TextBox inside a Border and set a background color
for Border to display it as TextBox background color.
-->
<Border Background="Yellow">
<TextBox
x:Name="TextBox1"
PlaceholderText="Input your name here."
GotFocus="TextBox_GetFocus"
/>
</Border>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Media;
using Windows.UI;
using Windows.UI.Xaml;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
private void TextBox_GetFocus(object sender, RoutedEventArgs e) {
// Set the TexBox focus background color
TextBox1.Background = new SolidColorBrush(Colors.Yellow);
}
}
}
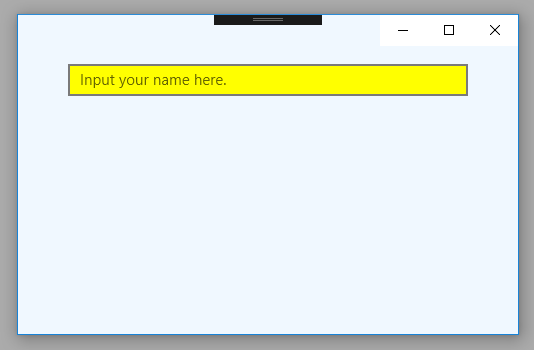
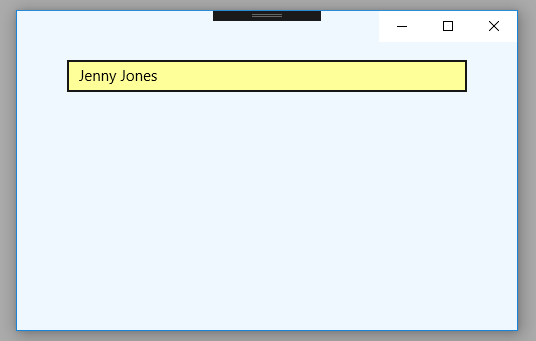