Compose TextField Remove Underline
The TextField is the text input widget of android jetpack compose library. TextField is an equivalent widget of the android view system’s EditText widget. TextField is used to enter and modify text.
The following jetpack compose tutorial will demonstrate to us how we can remove (actually hide) the underline from a TextField widget in an android application. We have to apply a simple trick to remove (hide) the underline from the TextField.
The TextField constructor’s ‘colors’ argument allows us to set or change colors for TextField’s various components such as text color, cursor color, label color, error color, background color, focused and unfocused indicator color, etc.
Jetpack developers can pass a TextFieldDefaults.textFieldColors() function with arguments value for the TextField ‘colors’ argument. There are many arguments for this ‘TextFieldDefaults.textFieldColors()’function such as textColor, disabledTextColor, backgroundColor, cursorColor, focusedIndicatorColor, unfocusedIndicatorColor, disabledIndicatorColor, errorIndicatorColor, leadingIconColor, etc.
The arguments named with ‘indicator’ means the underline of the TextField widget. So we can change the underline color of the TextField widget using those indicator colors. We can simply hide the underline from a TextField by setting a transparent color to those. Practically, we do not remove the underline from TextField, we only hide the underline from a TextField widget by setting its color value to transparent.
Jetpack developers can hide the underline from its focused state using the ‘focusedIndicatorColor’ argument. To hide underline from its unfocused state we can use the ‘unfocusedIndicatorColor’ argument value. The ‘disabledIndicatorColor’ allows us to hide TextField underline on its disabled state. And the ‘errorIndicatorColor’ allows setting an underline color for the TextField error state. We have to set the value of those colors to a transparent color.
This jetpack compose tutorial is written in an android studio IDE. Copy the code and run it on an emulator device or a real device to test how we hide the underline from a TextField widget. We also displayed screenshots of this tutorial’s emulator screen.
The following jetpack compose tutorial will demonstrate to us how we can remove (actually hide) the underline from a TextField widget in an android application. We have to apply a simple trick to remove (hide) the underline from the TextField.
The TextField constructor’s ‘colors’ argument allows us to set or change colors for TextField’s various components such as text color, cursor color, label color, error color, background color, focused and unfocused indicator color, etc.
Jetpack developers can pass a TextFieldDefaults.textFieldColors() function with arguments value for the TextField ‘colors’ argument. There are many arguments for this ‘TextFieldDefaults.textFieldColors()’function such as textColor, disabledTextColor, backgroundColor, cursorColor, focusedIndicatorColor, unfocusedIndicatorColor, disabledIndicatorColor, errorIndicatorColor, leadingIconColor, etc.
The arguments named with ‘indicator’ means the underline of the TextField widget. So we can change the underline color of the TextField widget using those indicator colors. We can simply hide the underline from a TextField by setting a transparent color to those. Practically, we do not remove the underline from TextField, we only hide the underline from a TextField widget by setting its color value to transparent.
Jetpack developers can hide the underline from its focused state using the ‘focusedIndicatorColor’ argument. To hide underline from its unfocused state we can use the ‘unfocusedIndicatorColor’ argument value. The ‘disabledIndicatorColor’ allows us to hide TextField underline on its disabled state. And the ‘errorIndicatorColor’ allows setting an underline color for the TextField error state. We have to set the value of those colors to a transparent color.
This jetpack compose tutorial is written in an android studio IDE. Copy the code and run it on an emulator device or a real device to test how we hide the underline from a TextField widget. We also displayed screenshots of this tutorial’s emulator screen.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.input.TextFieldValue
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(32.dp),
) {
var textState by remember { mutableStateOf(TextFieldValue()) }
TextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Put your name here") },
colors = TextFieldDefaults.textFieldColors(
focusedIndicatorColor = Color.Transparent,
unfocusedIndicatorColor = Color.Transparent,
disabledIndicatorColor = Color.Transparent,
errorIndicatorColor = Color.Transparent
),
enabled = true,
isError = false
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
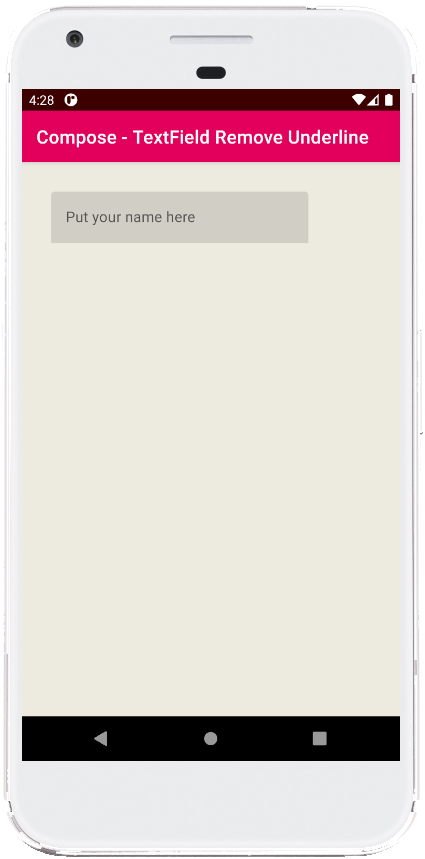
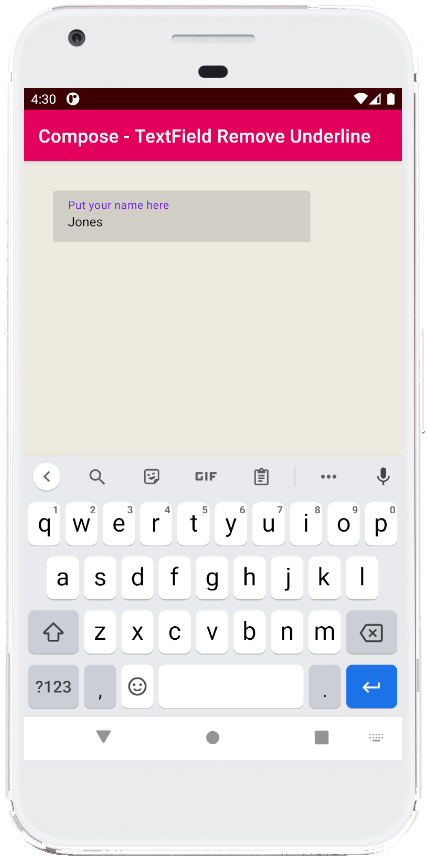
- jetpack compose - Button elevation
- jetpack compose - TextField request focus
- jetpack compose - TextField background color
- jetpack compose - TextField hint
- jetpack compose - Row align end
- jetpack compose - Box center alignment
- jetpack compose - How to use ModalDrawer
- jetpack compose - Snackbar action
- jetpack compose - Navigation arguments data type
- jetpack compose - Combined clickable
- jetpack compose - How to change NavigationBar color
- jetpack compose - Kotlinx serialization lenient parsing
- jetpack compose - Kotlinx serialization encode defaults
- jetpack compose - How to flow a list
- jetpack compose flow - Room implement search