Compose TextField Request Focus
The TextField is a text input widget of android jetpack compose library. Android app users can modify text using the TextField widget. Jetpack compose TextField is an equivalent of the android view system EditText widget. An android app screen can contain multiple TextField widgets to collect user data. So, how we can focus on a specific TextField widget programmatically?
This android jetpack compose tutorial will demonstrate how we can focus a TextField widget in code. The ‘FocusRequester’ object is used in conjunction with ‘Modifier.focusRequester’ to send requests to change focus. So, we can use this Modifier object’s ‘focusRequester’ element to set the focus on a TextField widget programmatically.
The modifier ‘focusRequester()’ function has a required argument name ‘focusRequester’ and its data type is FocusRequester. So that we have to pass a FocusRequester object to this function to make it workable.
At the beginning of this tutorial, we create an instance of the FocusRequester object by calling the FocusRequester() function. Then we assign this FocusRequester instance to the TextField modifier ‘focusRequester()’ function argument value. Now we have a TextField with a FocusRequester instance.
Next, we call this FocusRequester object’s requestFocus() method by a Button click event to request TextField focus programmatically. Finally, we can use this FocusRequester.requestFocus() method to set focus on the TextField widget from any event.
This tutorial code is written in an android studio IDE, so you can copy the code and paste it into your android studio IDE main activity file to check how our code work in an emulator device or in a physical android device. Screenshots are also displayed at the bottom of the code snippets. Look at those to understand the code efficiently.
This android jetpack compose tutorial will demonstrate how we can focus a TextField widget in code. The ‘FocusRequester’ object is used in conjunction with ‘Modifier.focusRequester’ to send requests to change focus. So, we can use this Modifier object’s ‘focusRequester’ element to set the focus on a TextField widget programmatically.
The modifier ‘focusRequester()’ function has a required argument name ‘focusRequester’ and its data type is FocusRequester. So that we have to pass a FocusRequester object to this function to make it workable.
At the beginning of this tutorial, we create an instance of the FocusRequester object by calling the FocusRequester() function. Then we assign this FocusRequester instance to the TextField modifier ‘focusRequester()’ function argument value. Now we have a TextField with a FocusRequester instance.
Next, we call this FocusRequester object’s requestFocus() method by a Button click event to request TextField focus programmatically. Finally, we can use this FocusRequester.requestFocus() method to set focus on the TextField widget from any event.
This tutorial code is written in an android studio IDE, so you can copy the code and paste it into your android studio IDE main activity file to check how our code work in an emulator device or in a physical android device. Screenshots are also displayed at the bottom of the code snippets. Look at those to understand the code efficiently.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.focus.*
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.input.TextFieldValue
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(32.dp),
verticalArrangement = Arrangement.spacedBy(24.dp)
) {
var textState by remember { mutableStateOf(TextFieldValue()) }
val focusRequester = FocusRequester()
TextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Put your name here") },
modifier = Modifier.focusRequester(focusRequester),
)
Button(onClick = {
focusRequester.requestFocus()
}) {
Text(text = "Focus On TextField")
}
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
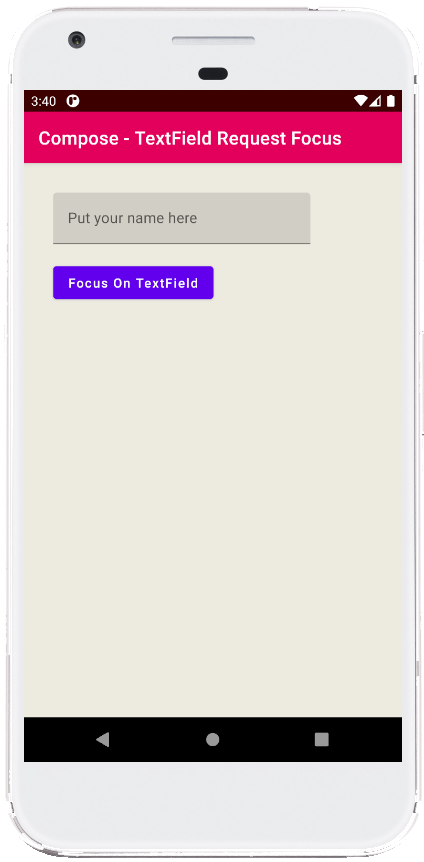
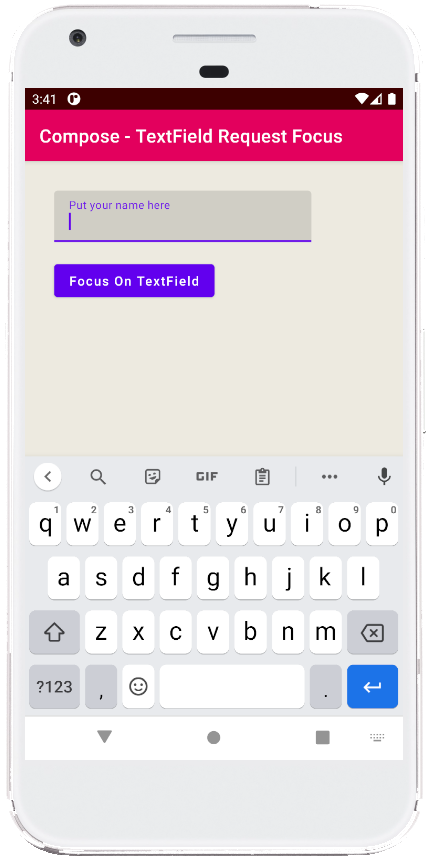
- jetpack compose - Draw line on Canvas
- jetpack compose - Draw circle on canvas
- jetpack compose - Rotate canvas
- jetpack compose - Canvas withTransform
- jetpack compose - Draw text on canvas
- jetpack compose - Double tap listener
- jetpack compose - Column vertical scrolling
- jetpack compose - Dragging
- jetpack compose - Weight modifier
- jetpack compose - Outlined Button
- jetpack compose - Button rounded corners
- jetpack compose - Button elevation
- jetpack compose - TextField remove underline
- jetpack compose - TextField background color
- jetpack compose - TextField hint