String remove substring
The following asp.net c# example code demonstrate us how can we remove a
substring from a string programmatically at run timein an asp.net
application. .Net framework's String.Remove() method allow us to remove
specified characters (range of characters)from a string and return a new
string object.
String.Remove(Int32, Int32) overloaded member allow us to return a new string in which a specified number of characters in thecurrent instance beginning at a specified index position have been deleted. So, we can delete specified number of charactersfrom a string which are beginning at a specified index position.
So, to delete a substring from a string, we need to know the starting index value of this substring and the length of thissubstring (number of characters in substring).
String.IndexOf(String) overloaded method reports the zero-based index of the first occurrence of the specified string in thisinstance. String.Length property return the number of characters in the instance string.
Finally, we can delete/remove a substring from a string by this wayString.Remove(String.IndexOf(Substring), Substring.Length).
String.Remove(Int32, Int32) overloaded member allow us to return a new string in which a specified number of characters in thecurrent instance beginning at a specified index position have been deleted. So, we can delete specified number of charactersfrom a string which are beginning at a specified index position.
So, to delete a substring from a string, we need to know the starting index value of this substring and the length of thissubstring (number of characters in substring).
String.IndexOf(String) overloaded method reports the zero-based index of the first occurrence of the specified string in thisinstance. String.Length property return the number of characters in the instance string.
Finally, we can delete/remove a substring from a string by this wayString.Remove(String.IndexOf(Substring), Substring.Length).
string-remove-substring.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
//this section create a string variable.
string plants = "Clumpfoot Cabbage. California Walnut. Catalina Ironwood";
Label1.Text = "string of plants..................<br />";
Label1.Text += plants+"<br />";
//substring to remove from string.
string substring = "Cabbage";
//this line get index of substring from string
int indexOfSubString = plants.IndexOf(substring);
//remove specified substring from string
string withoutSubString = plants.Remove(indexOfSubString, substring.Length);
Label1.Text += "<br />string removed substring...........";
Label1.Text += "<br />" + withoutSubString;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - string remove substring</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:MidnightBlue; font-style:italic;">
c# example - string remove substring
</h2>
<hr width="550" align="left" color="Gainsboro" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="string remove substring"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
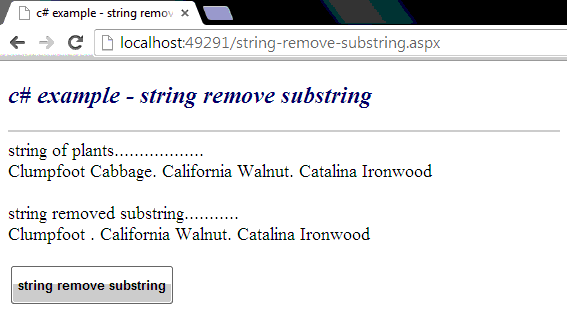