Compose TextField Background Color
Text is the main thing of any application. The jetpack compose makes it easier for the android application developer to display text, enter text and modify text. To write text inside an android application the jetpack compose library provides a widget name TextField. Displaying and managing a TextField widget is very easy in jetpack compose.
Sometimes android app developers need to change the default background color of a TextField widget in jetpack compose. Changing the TextField background color is a little bit difficult. We can’t directly put a color for any property of the TextField widget to change its background color.
This android application development tutorial will demonstrate to us how we can change the TextField background color in jetpack compose. TextField has a property/parameter/argument named ‘colors’. Here we can assign some values to change the TextField default colors such as background color, text color, cursor color, placeholder color, disabled text color, error label color, etc.
So, we can change the jetpack compose TextField background color by assigning its ‘colors’ property value. The ‘colors’ property value overrides the TextField default colors backgroundColor. This backgroundColor property value will show in our TextField as its background color. The following jetpack compose kotlin code and rendered screenshot will help you to better understand the thing.
Sometimes android app developers need to change the default background color of a TextField widget in jetpack compose. Changing the TextField background color is a little bit difficult. We can’t directly put a color for any property of the TextField widget to change its background color.
This android application development tutorial will demonstrate to us how we can change the TextField background color in jetpack compose. TextField has a property/parameter/argument named ‘colors’. Here we can assign some values to change the TextField default colors such as background color, text color, cursor color, placeholder color, disabled text color, error label color, etc.
So, we can change the jetpack compose TextField background color by assigning its ‘colors’ property value. The ‘colors’ property value overrides the TextField default colors backgroundColor. This backgroundColor property value will show in our TextField as its background color. The following jetpack compose kotlin code and rendered screenshot will help you to better understand the thing.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.input.TextFieldValue
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(32.dp),
verticalArrangement = Arrangement.spacedBy(24.dp)
) {
var textState by remember { mutableStateOf(TextFieldValue()) }
TextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Put your name here") },
colors = TextFieldDefaults.textFieldColors(
backgroundColor = Color(0xFFFFB7C5)
)
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
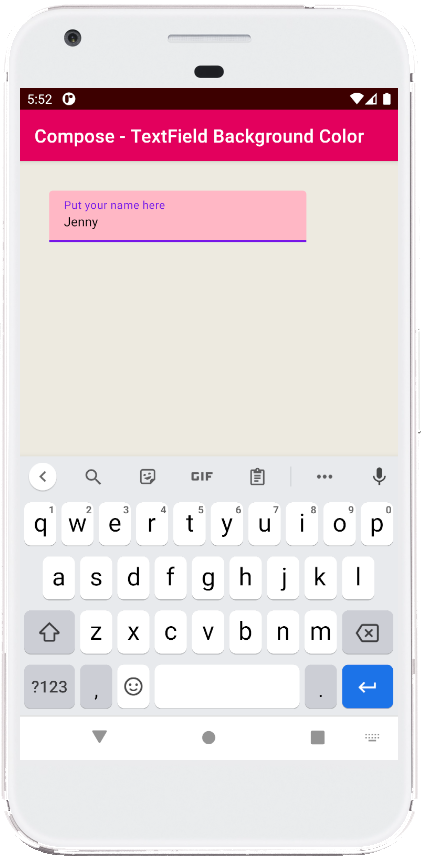
- jetpack compose - Button elevation
- jetpack compose - TextField request focus
- jetpack compose - TextField remove underline
- jetpack compose - TextField hint
- jetpack compose - TextField size
- jetpack compose - TextField error
- jetpack compose - TextField input type
- jetpack compose - TextField clear focus
- jetpack compose - TextField focus change listener
- jetpack compose - TextField IME action done
- jetpack compose - Card click
- jetpack compose - LazyColumn items indexed
- jetpack compose - LazyColumn add remove item
- jetpack compose - LazyColumn selectable
- jetpack compose - Row scrolling