Compose TextField Input Type
TextField is an equivalent widget of the android view system’s EditText widget. Jetpack compose TextField allows users to enter and modify text. That’s why TextField is a Text input widget of the jetpack compose library.
The following jetpack compose tutorial will demonstrate to us how we can input different types of text on the TextField widget using different types of Software keyboards. Such as how we can enter email, phone number, simple text, password, decimal, number, URI, etc to TextField with the specified soft keyboard type.
The TextField constructor’s ‘keyboardOptions’ parameter’s ‘keyboardType’ property allows us to define the Keyboard type for the focused TextField. The ‘keyboardType’ element accepts one value from Ascii, Decimal, Email, Number, NumberPassword, Password, Phone, Text, and Uri.
Those keyboard types are used for different purposes such as the ‘Decimal’ type soft keyboard used to request an IME that is capable of inputting decimals. The ‘Email’ type keyboard is used for inputting email addresses. The ‘Number’ type soft keyboard is used for inputting digits.
The ‘Password’ type keyboard is used for entering passwords. The ‘Uri’ type keyboard is used for entering URIs. And finally, the ‘Text’ type soft keyboard is used for displaying a regular keyboard and this ‘Text’ type keyboard is the default value for the ‘keyboardType’ element.
The following tutorial code placed some TextField widgets on the app screen. TextFields displays different types of keyboards in a focused state. Such as we displayed a regular Text type keyboard for a TextField, and a ‘Password’ type keyboard for another TextField. We also displayed an ‘Email’ type keyboard and a ‘Number’ type keyboard for separate TextFields.
Copy the code and run it into your android studio IDE to practically test how we displayed different types of soft keyboards for different TextField widgets. At the bottom of the code, we displayed screenshots of this tutorial's emulator output screen.
The following jetpack compose tutorial will demonstrate to us how we can input different types of text on the TextField widget using different types of Software keyboards. Such as how we can enter email, phone number, simple text, password, decimal, number, URI, etc to TextField with the specified soft keyboard type.
The TextField constructor’s ‘keyboardOptions’ parameter’s ‘keyboardType’ property allows us to define the Keyboard type for the focused TextField. The ‘keyboardType’ element accepts one value from Ascii, Decimal, Email, Number, NumberPassword, Password, Phone, Text, and Uri.
Those keyboard types are used for different purposes such as the ‘Decimal’ type soft keyboard used to request an IME that is capable of inputting decimals. The ‘Email’ type keyboard is used for inputting email addresses. The ‘Number’ type soft keyboard is used for inputting digits.
The ‘Password’ type keyboard is used for entering passwords. The ‘Uri’ type keyboard is used for entering URIs. And finally, the ‘Text’ type soft keyboard is used for displaying a regular keyboard and this ‘Text’ type keyboard is the default value for the ‘keyboardType’ element.
The following tutorial code placed some TextField widgets on the app screen. TextFields displays different types of keyboards in a focused state. Such as we displayed a regular Text type keyboard for a TextField, and a ‘Password’ type keyboard for another TextField. We also displayed an ‘Email’ type keyboard and a ‘Number’ type keyboard for separate TextFields.
Copy the code and run it into your android studio IDE to practically test how we displayed different types of soft keyboards for different TextField widgets. At the bottom of the code, we displayed screenshots of this tutorial's emulator output screen.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.input.KeyboardType
import androidx.compose.ui.text.input.PasswordVisualTransformation
import androidx.compose.ui.text.input.TextFieldValue
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(32.dp),
verticalArrangement = Arrangement.spacedBy(24.dp)
) {
var textState by remember { mutableStateOf(TextFieldValue()) }
TextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Keyboard Type Text") },
modifier = Modifier.fillMaxWidth(),
keyboardOptions = KeyboardOptions(
keyboardType = KeyboardType.Text
)
)
TextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Keyboard Type Password") },
modifier = Modifier.fillMaxWidth(),
keyboardOptions = KeyboardOptions(
keyboardType = KeyboardType.Password
),
visualTransformation = PasswordVisualTransformation()
)
OutlinedTextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Keyboard Type Email") },
modifier = Modifier.fillMaxWidth(),
keyboardOptions = KeyboardOptions(
keyboardType = KeyboardType.Email
)
)
OutlinedTextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Keyboard Type Number") },
modifier = Modifier.fillMaxWidth(),
keyboardOptions = KeyboardOptions(
// more types are Ascii, NumberPassword, Phone, Uri
keyboardType = KeyboardType.Number
)
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
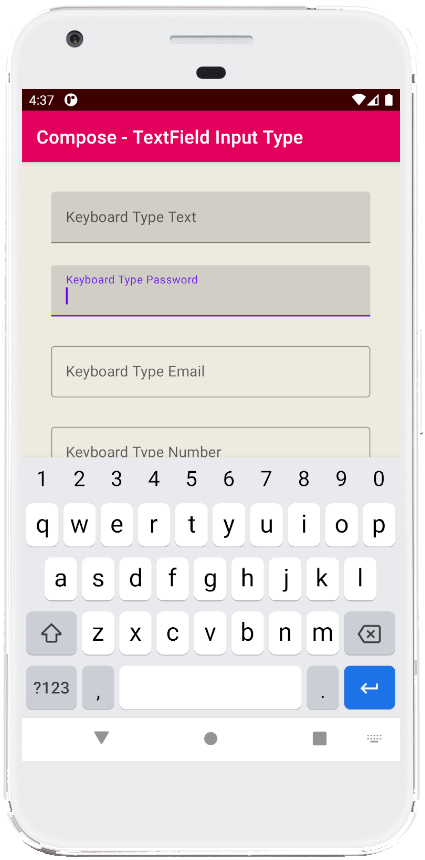

- jetpack compose - TextField size
- jetpack compose - TextField error
- jetpack compose - TextField clear focus
- jetpack compose - TextField focus change listener
- jetpack compose - LazyColumn add remove item
- jetpack compose - LazyColumn selectable
- jetpack compose - Box rounded corners
- jetpack compose - Box center
- jetpack compose - Column center
- jetpack compose - Column background color
- jetpack compose - Column border
- jetpack compose - Column spacing
- jetpack compose - Column scrollable
- jetpack compose - Row spacing
- jetpack compose - Row scrolling