Compose TextField IME Action Done
TextField is an equivalent widget of the android view system’s EditText widget. In a jetpack compose application TextField is used to enter and modify text. So, TextField is a Text input widget of the jetpack compose library.
The following jetpack compose tutorial will demonstrate to us how can we show IME action ‘Done’ on the soft keyboard and how we can respond to IME action Done click.
TextField constructor’s ‘keyboardOptions’ argument allows us to set the TextField-related soft keyboard’s IME action. We can set one of the options from Default, Done, Sent, Go, Next, Search, etc. The ‘ImeAction.Default’ is the default value for this argument. So using this keyboardOptions parameter we set the IME action Done. IME action Done represents that the user is done providing input to a group of inputs.
On the other hand, TextField ‘keyboardActions’ argument allows us to specify actions that will be triggered in response to users triggering IME action on the software keyboard. So, we can handle the IME action done event in this ‘keyboardActions’ parameter’s ‘onDone’ section.
In this tutorial, we clear the focus from the TextField widget on the IME action done event. We also show a message that the IME action ‘Done’ triggers with user inputted text.
Copy the following code snippet into your android studio IDE. Run the code on an emulator device to check how we implemented the TextField IME action ‘Done’. The screenshots also help you to understand the scenario without running it into a device.
The following jetpack compose tutorial will demonstrate to us how can we show IME action ‘Done’ on the soft keyboard and how we can respond to IME action Done click.
TextField constructor’s ‘keyboardOptions’ argument allows us to set the TextField-related soft keyboard’s IME action. We can set one of the options from Default, Done, Sent, Go, Next, Search, etc. The ‘ImeAction.Default’ is the default value for this argument. So using this keyboardOptions parameter we set the IME action Done. IME action Done represents that the user is done providing input to a group of inputs.
On the other hand, TextField ‘keyboardActions’ argument allows us to specify actions that will be triggered in response to users triggering IME action on the software keyboard. So, we can handle the IME action done event in this ‘keyboardActions’ parameter’s ‘onDone’ section.
In this tutorial, we clear the focus from the TextField widget on the IME action done event. We also show a message that the IME action ‘Done’ triggers with user inputted text.
Copy the following code snippet into your android studio IDE. Run the code on an emulator device to check how we implemented the TextField IME action ‘Done’. The screenshots also help you to understand the scenario without running it into a device.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.text.KeyboardActions
import androidx.compose.foundation.text.KeyboardOptions
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.platform.LocalFocusManager
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.text.input.ImeAction
import androidx.compose.ui.text.input.TextFieldValue
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(32.dp),
verticalArrangement = Arrangement.spacedBy(24.dp)
) {
var textState by remember { mutableStateOf(TextFieldValue()) }
var txt by remember { mutableStateOf("") }
val localFocusManager = LocalFocusManager.current
Text(
text = txt,
fontSize = 22.sp,
fontFamily = FontFamily.Monospace,
color = Color(0xFF0047AB)
)
OutlinedTextField(
value = textState,
onValueChange = { textState = it },
label = { Text(text = "Input your name") },
modifier = Modifier.fillMaxWidth(),
keyboardOptions = KeyboardOptions(
imeAction = ImeAction.Done
),
keyboardActions = KeyboardActions(
onDone = {
localFocusManager.clearFocus()
txt = "IME action done : " + textState.text
}
)
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
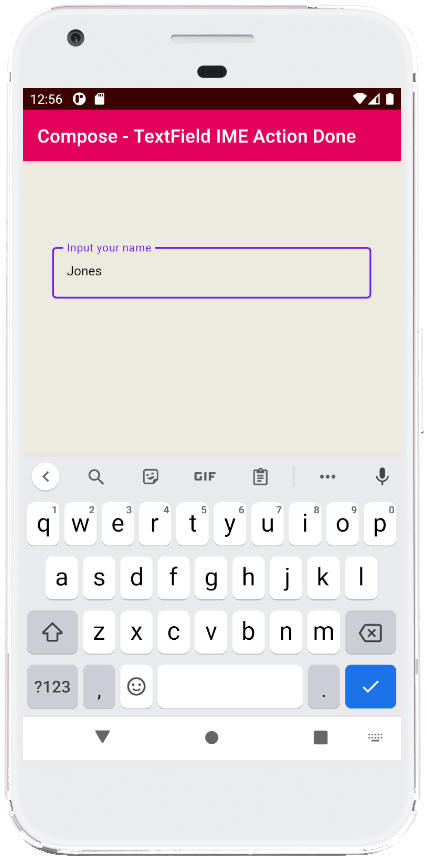
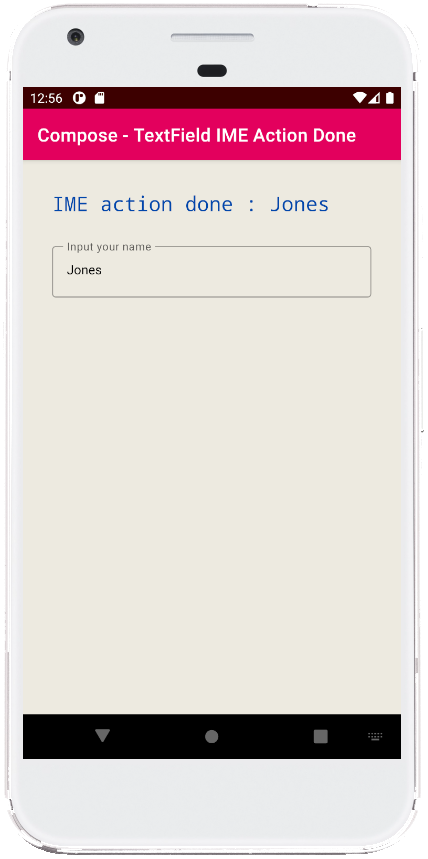
- jetpack compose - Infinite float animation
- jetpack compose - Draw circle on canvas
- jetpack compose - Canvas withTransform
- jetpack compose - Double tap listener
- jetpack compose - Dragging
- jetpack compose - Outlined Button
- jetpack compose - Button elevation
- jetpack compose - TextField size
- jetpack compose - Card click
- jetpack compose - LazyColumn items indexed
- jetpack compose - LazyColumn add remove item
- jetpack compose - LazyColumn selectable
- jetpack compose - Show toast message
- jetpack compose - Box alignment
- jetpack compose - Column border