Compose LazyColumn Items Indexed
The LazyColumn is a special type of Column layout that has items vertical scrolling feature enabled. In the pre-compose application, there is a similar widget name RecyclerView. Like the RecyclerView widget, LayColumn is used to display a long list of items.
Items may come from a room database, API data, or whatever it is. The main feature of the LazyColumn widget is, that it shows a vertically scrolling list that only composes and lays out the currently visible items. This feature allows a LazyColumn to scroll a long list very smoothly and it also takes a small size of device memory.
We can add a single item to the LazyColumn items collection, we also can add multiple items to its items collection at once. But how does an android jetpack compose developers add items to the LazyColumn collection of items with their index value? This jetpack compose tutorial will describe to us how we can get LazyColumn items with their corresponding index value.
The LazyColumn’s ‘LazyListScope.itemsIndexed()’ inline function allows us to pass an items list to the LazyColumn items collection. This inline function also allows us to access two variables while iterating its items, the first one is the item’s index value and the second one is the item itself. So, we can easily display the item’s associated index value to the app user interface and we also can use this index value to perform other related actions.
In this jetpack compose tutorial we display a list of items with the item counter. We generate the counter by adding one with the item index value because the index is zero-based.
The following kotlin code is written in an android studio IDE. Copy the code and paste it into your android studio compose project main activity file and run the app on an emulator to see the result. At the bottom of this tutorial we also displayed screenshots of this code output, this also can help you to understand the terms without running it on an android device.
Items may come from a room database, API data, or whatever it is. The main feature of the LazyColumn widget is, that it shows a vertically scrolling list that only composes and lays out the currently visible items. This feature allows a LazyColumn to scroll a long list very smoothly and it also takes a small size of device memory.
We can add a single item to the LazyColumn items collection, we also can add multiple items to its items collection at once. But how does an android jetpack compose developers add items to the LazyColumn collection of items with their index value? This jetpack compose tutorial will describe to us how we can get LazyColumn items with their corresponding index value.
The LazyColumn’s ‘LazyListScope.itemsIndexed()’ inline function allows us to pass an items list to the LazyColumn items collection. This inline function also allows us to access two variables while iterating its items, the first one is the item’s index value and the second one is the item itself. So, we can easily display the item’s associated index value to the app user interface and we also can use this index value to perform other related actions.
In this jetpack compose tutorial we display a list of items with the item counter. We generate the counter by adding one with the item index value because the index is zero-based.
The following kotlin code is written in an android studio IDE. Copy the code and paste it into your android studio compose project main activity file and run the app on an emulator to see the result. At the bottom of this tutorial we also displayed screenshots of this code output, this also can help you to understand the terms without running it on an android device.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.itemsIndexed
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Box(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(8.dp)
) {
val alphabets = listOf("A", "B", "C", "D", "E", "F",
"G", "H", "I", "J", "K", "L", "M", "N", "O", "P",
"Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z")
LazyColumn(
Modifier.fillMaxSize(),
verticalArrangement = Arrangement.spacedBy(8.dp)
) {
itemsIndexed(alphabets){ index, item ->
Card(
modifier = Modifier
.fillMaxWidth()
.wrapContentHeight(
align = Alignment.CenterVertically
),
elevation = 4.dp,
shape = RoundedCornerShape(8.dp),
backgroundColor = Color(0xFF7BB661)
) {
Text(
text = "${index+1}. " + item,
modifier = Modifier.padding(25.dp),
textAlign = TextAlign.Center,
fontSize = 35.sp,
fontWeight = FontWeight.Bold
)
}
}
}
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}

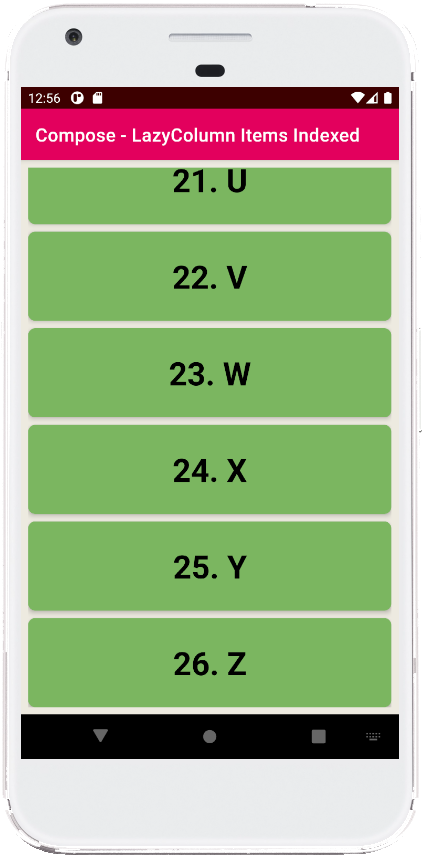
- jetpack compose - TextField size
- jetpack compose - TextField error
- jetpack compose - TextField IME action done
- jetpack compose - Card click
- jetpack compose - LazyColumn add remove item
- jetpack compose - LazyColumn selectable
- jetpack compose - Box rounded corners
- jetpack compose - Box center
- jetpack compose - Column center
- jetpack compose - Column background color
- jetpack compose - Column border
- jetpack compose - Column spacing
- jetpack compose - Column scrollable
- jetpack compose - Row spacing
- jetpack compose - Row scrolling