MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.*
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.CircleShape
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.graphics.Color
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.graphics.SolidColor
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedObjectState: Bundle?) {
super.onCreate(savedObjectState)
setContent {
GetScaffold()
}
}
@Composable
fun GetScaffold() {
Scaffold(
topBar = {
TopAppBar(
title = {
Text(
text = "Compose - Combined Clickable"
)
},
backgroundColor = Color(0xFF1ca9c9),
)
},
content = { MainContent() },
)
}
@OptIn(ExperimentalFoundationApi::class)
@Composable
fun MainContent() {
val counter = remember {mutableStateOf(0)}
val message = remember { mutableStateOf("Click Action")}
Column(
horizontalAlignment = Alignment.CenterHorizontally,
verticalArrangement = Arrangement.spacedBy(24.dp),
modifier = Modifier.padding(12.dp)
) {
Text(
text = message.value,
modifier = Modifier
.clip(RoundedCornerShape(12.dp))
.background(Color(0xFFD6b75a))
.fillMaxWidth()
.padding(12.dp)
.wrapContentSize(Alignment.Center),
style = MaterialTheme.typography.h4
)
Box(
modifier = Modifier
.size(250.dp)
.clip(CircleShape)
.background(SolidColor(Color(0xFFE6dbad)))
.combinedClickable(
enabled = true,
onClick = {
counter.value++
message.value = "Clicked"
},
onDoubleClick = {
counter.value +=2
message.value = "Double Clicked"
},
onLongClick = {
counter.value +=5
message.value = "Long Clicked"
}
)
.padding(12.dp),
contentAlignment = Alignment.Center
) {
Text(
text = "Counter ${counter.value}",
style = MaterialTheme.typography.h5
)
}
}
}
}


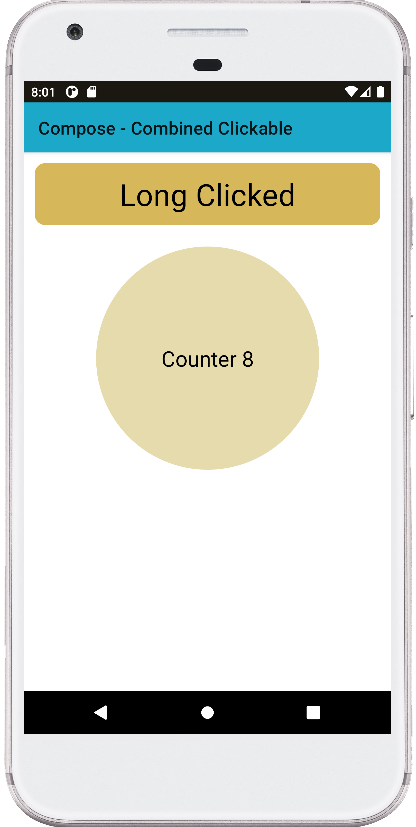
- jetpack compose - Background brush
- jetpack compose - Double click listener
- jetpack compose - Long click listener
- jetpack compose - Pass onClick event to function
- jetpack compose - TabRow custon indicator
- jetpack compose - Get primary language
- jetpack compose - Get screen orientation
- jetpack compose - How to change StatusBar color
- jetpack compose - How to change NavigationBar color
- jetpack compose - How to change SystemBars color
- jetpack compose - How to hide system bars
- jetpack compose - Detect screen orientation change
- jetpack compose ktor - How to get api data
- jetpack compose - Icon from vector resource
- jetpack compose - IconButton from vector resource