This code demonstrates a Jetpack Compose application that displays a list of colors in a LazyColumn. Here's a breakdown of the code:
1. Define a data source for the list:
- The
colorsFlow
variable is a flow that emits a list of strings after a one-second delay. This simulates fetching data from an asynchronous source.
2. Collect the data in the UI:
- The
MainContent
composable usescollectAsState
to subscribe to thecolorsFlow
and collect the emitted list of colors. Thecolors
variable stores the current value of the flow.
3. Display the list using LazyColumn:
- A
LazyColumn
is used to display the list of colors efficiently. It renders only the visible items on the screen and improves performance for large lists.
4. Render each color in a Card:
- The
items
function iterates over thecolors
list and renders each color inside aCard
with some padding.
Summary: This code showcases how to use Jetpack Compose to declaratively build a user interface with a list of items fetched from an asynchronous data source. It leverages the power of flows to manage the asynchronous data flow and recompose the UI as the data updates.
MainActivity.kt
package com.cfsuman.composestate
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material.*
import androidx.compose.runtime.Composable
import androidx.compose.runtime.collectAsState
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.cfsuman.composestate.ui.theme.ComposeStateTheme
import kotlinx.coroutines.delay
import kotlinx.coroutines.flow.flow
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ComposeStateTheme {
Scaffold(
topBar = { TopAppBar(
title = { Text(text = "Compose - Flow List")}
) },
content = { MainContent() },
backgroundColor = Color(0xFFEDEAE0)
)
}
}
}
}
@Composable
fun MainContent() {
val colorsFlow = flow<List<String>> {
delay(1000)
val list = listOf("Red","Green","Yellow","Blue","Black")
emit(list)
}
val colors by colorsFlow
.collectAsState(initial = emptyList())
Box(
Modifier.fillMaxSize(),
contentAlignment = Alignment.Center
) {
LazyColumn(
Modifier.fillMaxSize().padding(12.dp),
verticalArrangement = Arrangement.spacedBy(12.dp)
){
items(colors){color->
Card(modifier = Modifier.fillMaxWidth()) {
Text(
text = color,
modifier = Modifier.padding(24.dp)
)
}
}
}
}
}
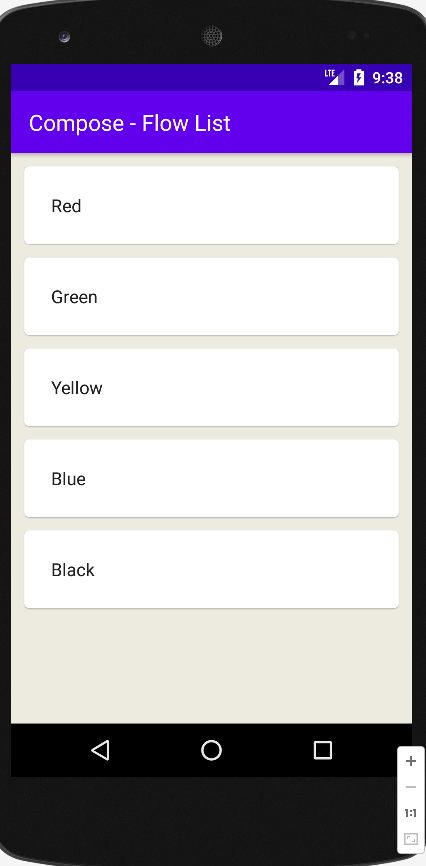
- jetpack compose - Kotlinx serialization allow special floating point values
- jetpack compose - Kotlinx serialization build json array
- jetpack compose - Flow current time
- jetpack compose - flowOf flow builder
- jetpack compose - Convert list to flow
- jetpack compose - Count down flow
- jetpack compose - How to use ViewModel state
- jetpack compose - Flow using ViewModel
- jetpack compose - Search Room data using ViewModel
- jetpack compose - ViewModel Room add insert data
- jetpack compose - Icon from vector resource