The code demonstrates how to continuously display the current time using Jetpack Compose. It achieves this by leveraging a flow and a state composable.
Flow to emit time:
- A
flow
namedtimerFlow
is created that emits a string value every 1 second. - Inside the
flow
, thegetTime
function is called to get the current time in hh:mm:ss format. - The retrieved time is then emitted using the
emit
function.
- A
Composable to display time:
- The
MainContent
composable is responsible for displaying the current time. - It defines a function named
getTime
that retrieves the current time as a string. - A state variable named
currentTime
is created usingcollectAsState
which initially holds the value returned by thegetTime
function. - The
currentTime
is then displayed within aText
composable with a font size of 40sp.
- The
Summary
The code effectively utilizes a flow to periodically update the current time and a state composable to display it on the screen. This approach ensures that the UI stays up-to-date with the latest time.
MainActivity.kt
package com.cfsuman.composestate
import android.os.Bundle
import android.text.format.DateFormat
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material.*
import androidx.compose.runtime.Composable
import androidx.compose.runtime.collectAsState
import androidx.compose.runtime.getValue
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.TextStyle
import androidx.compose.ui.unit.sp
import com.cfsuman.composestate.ui.theme.ComposeStateTheme
import kotlinx.coroutines.delay
import kotlinx.coroutines.flow.flow
import java.util.*
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
ComposeStateTheme {
Scaffold(
topBar = { TopAppBar(
title = { Text(text = "Compose - Flow Current Time")}
) },
content = { MainContent() },
backgroundColor = Color(0xFFEDEAE0)
)
}
}
}
}
@Composable
fun MainContent() {
fun getTime():String{
return DateFormat.format("hh:mm:ss",
Date(System.currentTimeMillis())).toString()
}
val timerFlow = flow<String> {
delay(1000)
emit(getTime())
}
val currentTime by timerFlow
.collectAsState(initial = getTime())
Box(
Modifier.fillMaxSize(),
contentAlignment = Alignment.Center
) {
Text(
text = currentTime,
style = TextStyle(fontSize = 40.sp)
)
}
}
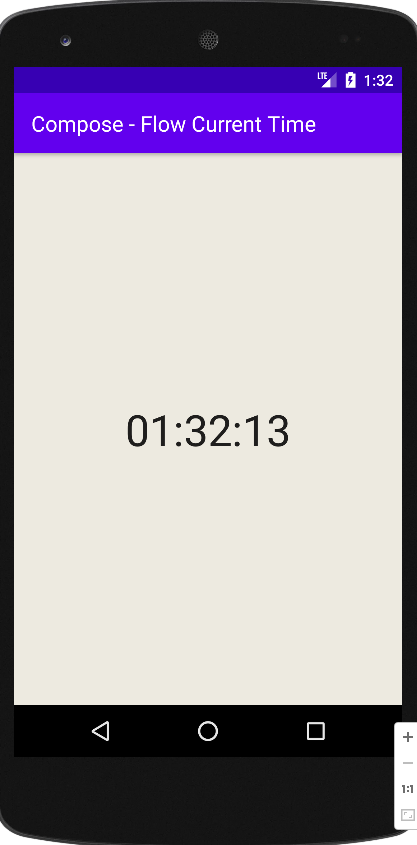
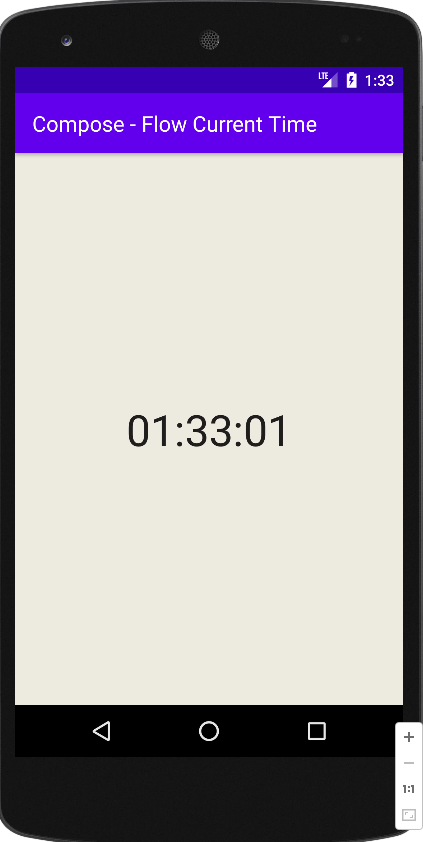
- jetpack compose - How to flow a list
- jetpack compose - flowOf flow builder
- jetpack compose - Convert list to flow
- jetpack compose - Count down flow
- jetpack compose - How to use ViewModel state
- jetpack compose - Flow using ViewModel
- jetpack compose - Count up flow by ViewModel
- jetpack compose flow - Room add remove update
- jetpack compose flow - Room implement search
- jetpack compose - Search Room data using ViewModel
- jetpack compose - ViewModel Room add insert data
- jetpack compose - ViewModel Room edit update data
- jetpack compose - ViewModel Room delete clear data
- compose glance - How to create app widget
- jetpack compose - Icon from vector resource