UWP - Select TextBox all text on focus
The following Universal Windows Platform application development
tutorial demonstrates how we can select all text of a TextBox when the
specified TextBox gets focused.
In this UWP tutorial, we will handle the TextBox class GotFocus event to select all text of this TextBox instance. We will use the TextBox class SelectAll() method to select TextBox all text when TextBox is focused.
The TextBox class represents a control that can be used to display and edit plain text. A TextBox can be single or multi-line. The TextBox control enables the UWP app user to enter text into a UWP app. The TextBox is typically used to capture a single line of text but the UWP developers can configure it to capture multiple lines of text. The text displays on the screen in a simple uniform plaintext format.
The TextBox GotFocus event occurs when the TextBox UIElement receives focus. The GotFocus event is raised asynchronously, so the focus can move again before bubbling is complete. The TextBox GotFocus event is inherited from UIElement.
On the TextBox GotFocus event, we will call the TextBox SelectAll() method to select all the text of the specified TextBox control. The TextBox class SelectAll() method selects the entire contents of the text box.
In this UWP tutorial, we will handle the TextBox class GotFocus event to select all text of this TextBox instance. We will use the TextBox class SelectAll() method to select TextBox all text when TextBox is focused.
The TextBox class represents a control that can be used to display and edit plain text. A TextBox can be single or multi-line. The TextBox control enables the UWP app user to enter text into a UWP app. The TextBox is typically used to capture a single line of text but the UWP developers can configure it to capture multiple lines of text. The text displays on the screen in a simple uniform plaintext format.
The TextBox GotFocus event occurs when the TextBox UIElement receives focus. The GotFocus event is raised asynchronously, so the focus can move again before bubbling is complete. The TextBox GotFocus event is inherited from UIElement.
On the TextBox GotFocus event, we will call the TextBox SelectAll() method to select all the text of the specified TextBox control. The TextBox class SelectAll() method selects the entire contents of the text box.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Padding="50" Background="AliceBlue">
<!--
GotFocus
Occurs when a UIElement receives focus.
(Inherited from UIElement)
-->
<TextBox
x:Name="TextBox1"
PlaceholderText="Input some text here."
Margin="5"
GotFocus="TextBox_GotFocus"
/>
<TextBox
x:Name="TextBox2"
PlaceholderText="Input some text here."
Margin="5"
GotFocus="TextBox_GotFocus"
/>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
}
void TextBox_GotFocus(object sender, RoutedEventArgs e)
{
// Get the instance of TextBox
TextBox textBox = sender as TextBox;
// Select all existing text of TextBox on focus
textBox.SelectAll();
}
}
}
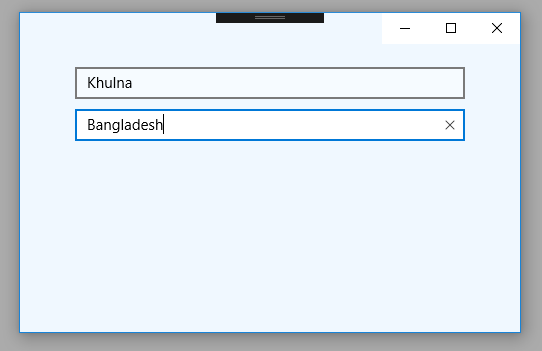
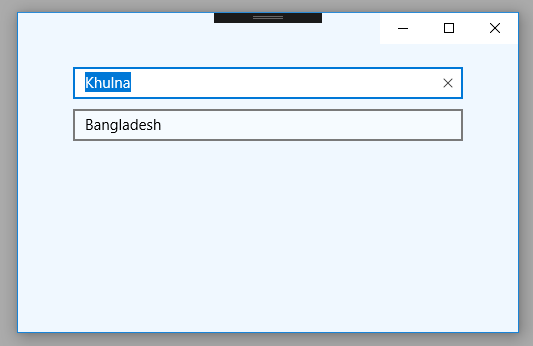
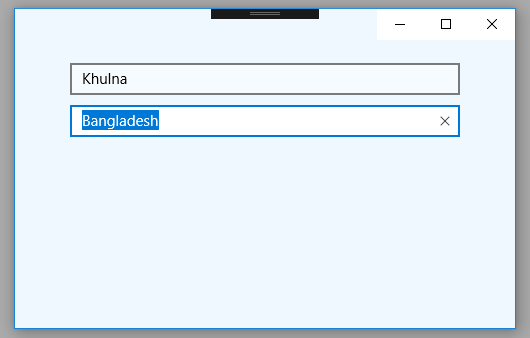