UWP - Change the title bar text programmatically
The following Universal Windows Platform application development
tutorial demonstrates how we can set or change the title bar text
programmatically. Here we will get the application view for the current view.
Then we will set the title text for the application view.
The ApplicationView class represents the active application view and associated states and behaviors. The ApplicationView class GetForCurrentView() method gets the view state and behavior settings of the active application. This method returns an ApplicationView instance that can be used to get and set app display properties.
The ApplicationView class Title property gets or sets the displayed title of the window. This Title property value is a String which is the title of the window. So finally, using this ApplicationView Title property the UWP app developers can change the title bar text programmatically.
When the Title property is not set, the system shows the app's display name in the title bar, as specified in the Display name field in the app manifest. When the UWP app developers set the Title property, Windows may choose to append the app display name to the end of the Title value they set.
The ApplicationView class represents the active application view and associated states and behaviors. The ApplicationView class GetForCurrentView() method gets the view state and behavior settings of the active application. This method returns an ApplicationView instance that can be used to get and set app display properties.
The ApplicationView class Title property gets or sets the displayed title of the window. This Title property value is a String which is the title of the window. So finally, using this ApplicationView Title property the UWP app developers can change the title bar text programmatically.
When the Title property is not set, the system shows the app's display name in the title bar, as specified in the Display name field in the app manifest. When the UWP app developers set the Title property, Windows may choose to append the app display name to the end of the Title value they set.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Background="Pink" Padding="50">
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.ViewManagement;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
// Get the application view
ApplicationView appView = ApplicationView.GetForCurrentView();
// Change the application view title text
appView.Title = "This is new title";
}
}
}
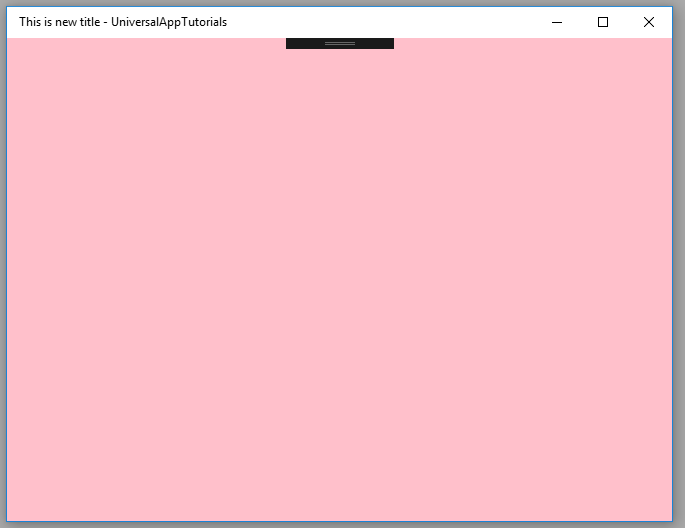