UWP - Launch the app in full-screen mode
The following Universal Windows Platform application development
tutorial demonstrates how we can launch an app in full-screen mode.
In this UWP tutorial, we will set the ApplicationView class PreferredLaunchWindowingMode property value to FullScreen to launch the app in full-screen mode.
The ApplicationView class represents the active application view and associated states and behaviors.
The ApplicationView class PreferredLaunchWindowingMode property gets or sets a value that indicates the windowing mode the app launches with. This property value is an ApplicationViewWindowingModeenumeration value that indicates the windowing mode of the app. By default, PreferredLaunchWindowingMode is set to Auto.
The ApplicationViewWindowingMode enumeration defines constants that specify whether the app window is auto-sized, full-screen, or set to a specific size on launch. The enumeration value FullScreen specifies the window is full-screen.
So finally, the UWP developers can launch the app in full-screen mode by setting the ApplicationView class ApplicationViewWindowingMode property value to ApplicationViewWindowingMode.FullScreen.
In this UWP tutorial, we will set the ApplicationView class PreferredLaunchWindowingMode property value to FullScreen to launch the app in full-screen mode.
The ApplicationView class represents the active application view and associated states and behaviors.
The ApplicationView class PreferredLaunchWindowingMode property gets or sets a value that indicates the windowing mode the app launches with. This property value is an ApplicationViewWindowingModeenumeration value that indicates the windowing mode of the app. By default, PreferredLaunchWindowingMode is set to Auto.
The ApplicationViewWindowingMode enumeration defines constants that specify whether the app window is auto-sized, full-screen, or set to a specific size on launch. The enumeration value FullScreen specifies the window is full-screen.
So finally, the UWP developers can launch the app in full-screen mode by setting the ApplicationView class ApplicationViewWindowingMode property value to ApplicationViewWindowingMode.FullScreen.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Padding="50" Background="SkyBlue">
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.ViewManagement;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
/*
ApplicationView
Represents the active application view and
associated states and behaviors.
ApplicationView.PreferredLaunchWindowingMode
Gets or sets a value that indicates the windowing mode the app launches with.
ApplicationViewWindowingMode
FullScreen: The window is launched in full-screen mode.
Full-screen mode in not the same as maximized.
*/
// Set app window preferred launch windowing mode to full screen
ApplicationView.PreferredLaunchWindowingMode = ApplicationViewWindowingMode.FullScreen;
}
}
}
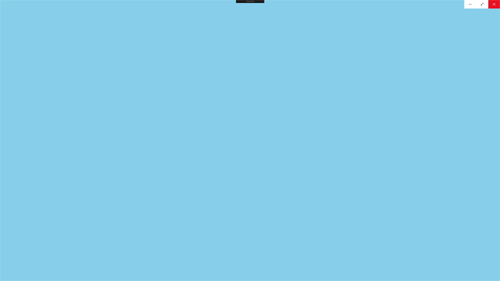