UWP - Multiline TextBox
The TextBox class represents a control that can be used to display and
edit plain text. A TextBox can be single or multi-line. The TextBox control
enables the UWP app user to enter text into a UWP app. The TextBox is
typically used to capture a single line of text but the UWP developers can
configure it to capture multiple lines of text. The text displays on the
screen in a simple uniform plaintext format.
The following Universal Windows Platform application development tutorial demonstrates how we can create/display a multiline TextBox. The AcceptReturns and TextWrapping properties control whether the TextBox displays text on more than one line.
The TextBox class AcceptsReturn property gets or sets the value that determines whether the text box allows and displays the newline or return characters. This property value is a Boolean. The property value is true if the text box allows newline characters otherwise the value is false.
The default value of this property is false. The UWP app developers have to set this AcceptsReturn property value to true to display a multiline TextBox on their app screen.
The UWP developers can enable multi-line text in a TextBox control by using the AcceptsReturn property. They can use ScrollViewer.HorizontalScrollBarVisibility or ScrollViewer.VerticalScrollBarVisibility attached properties to change scrollbar behavior.
The TextBox class TextWrapping property gets or sets how the line breaking occurs if a line of text extends beyond the available width of the text box. This property value is a TextWrapping which is one of the TextWrapping enumeration values. The default value is NoWrap. The TextWrapping enumeration specifies whether text wraps when it reaches the edge of its container.
Here we will set the TextWrapping property value to Wrap to display a multiline TextBox in our UWP app. TextWrapping value Wrap occurs line breaking if a line of text overflows beyond the available width of its container. Line breaking also occurs even if the text logic can't determine any line break opportunity.
The following Universal Windows Platform application development tutorial demonstrates how we can create/display a multiline TextBox. The AcceptReturns and TextWrapping properties control whether the TextBox displays text on more than one line.
The TextBox class AcceptsReturn property gets or sets the value that determines whether the text box allows and displays the newline or return characters. This property value is a Boolean. The property value is true if the text box allows newline characters otherwise the value is false.
The default value of this property is false. The UWP app developers have to set this AcceptsReturn property value to true to display a multiline TextBox on their app screen.
The UWP developers can enable multi-line text in a TextBox control by using the AcceptsReturn property. They can use ScrollViewer.HorizontalScrollBarVisibility or ScrollViewer.VerticalScrollBarVisibility attached properties to change scrollbar behavior.
The TextBox class TextWrapping property gets or sets how the line breaking occurs if a line of text extends beyond the available width of the text box. This property value is a TextWrapping which is one of the TextWrapping enumeration values. The default value is NoWrap. The TextWrapping enumeration specifies whether text wraps when it reaches the edge of its container.
Here we will set the TextWrapping property value to Wrap to display a multiline TextBox in our UWP app. TextWrapping value Wrap occurs line breaking if a line of text overflows beyond the available width of its container. Line breaking also occurs even if the text logic can't determine any line break opportunity.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<StackPanel Padding="50" Background="AliceBlue">
<TextBox
x:Name="TextBox1"
PlaceholderText="Input some text here."
AcceptsReturn="True"
TextWrapping="Wrap"
Margin="5"
/>
<TextBox
x:Name="TextBox2"
PlaceholderText="Input some text here."
Margin="5"
/>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
UpdateSecondTextBox();
}
private void UpdateSecondTextBox() {
/*
TextBox.AcceptsReturn
Gets or sets the value that determines whether the text
box allows and displays the newline or return characters.
TextBox.TextWrapping
Gets or sets how line breaking occurs if a line of text
extends beyond the available width of the text box.
*/
// Programmatically allow multiline input for second TextBox
TextBox2.AcceptsReturn = true;
TextBox2.TextWrapping = TextWrapping.Wrap;
}
}
}
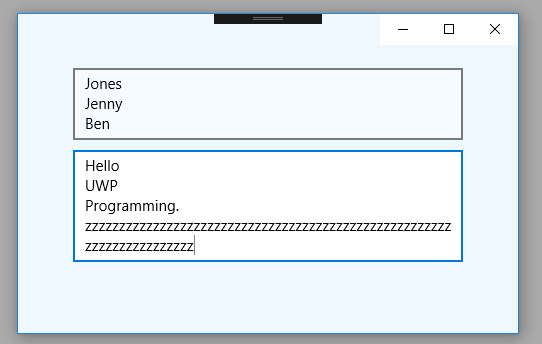