Introduction
This code snippet showcases how to retrieve the device's battery temperature programmatically in an Android application written in Kotlin. It utilizes the Android Broadcast mechanism to listen for battery-related changes and extract the temperature value.
Breakdown
The code is implemented within the MainActivity.kt
file. Here's a step-by-step breakdown:
Broadcast Receiver:
- A
BroadcastReceiver
subclass is defined to handle incoming broadcasts related to battery changes. - The
onReceive
method is overridden to handle the received intent.
- A
Obtaining Battery Temperature:
- Inside
onReceive
, theintent
object carries information about the battery change. - The
getIntExtra
method withBatteryManager.EXTRA_TEMPERATURE
as the key retrieves the battery temperature value in tenths of a degree Celsius (integer). - The retrieved value is converted to a float and divided by 10 to get the actual temperature.
- Inside
Displaying Temperature:
- The formatted temperature string, including the degree symbol (
°C
), is assigned to thetext
property of thetextView
in the layout.
- The formatted temperature string, including the degree symbol (
Activity Setup:
- The
onCreate
method of theMainActivity
class is responsible for setting up the broadcast receiver. - An
IntentFilter
object is created to specify the type of broadcast we're interested in (battery changes -Intent.ACTION_BATTERY_CHANGED
). - Finally, the
registerReceiver
method registers the previously defined broadcast receiver with the specified intent filter.
- The
Layout (activity_main.xml):
- This file defines the user interface layout of the activity. In this case, it simply contains a single
TextView
element where the battery temperature will be displayed.
- This file defines the user interface layout of the activity. In this case, it simply contains a single
Summary
This code demonstrates a practical approach to programmatically access and display the device's battery temperature in an Android app. By leveraging the BroadcastReceiver and BatteryManager functionalities, the application can stay updated on battery changes and provide users with this valuable information.
package com.example.jetpack
import android.content.BroadcastReceiver
import android.content.Context
import android.content.Intent
import android.content.IntentFilter
import android.os.BatteryManager
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
// initialize a new broadcast receiver instance
private val receiver:BroadcastReceiver = object: BroadcastReceiver(){
override fun onReceive(context: Context?, intent: Intent?) {
// get battery temperature programmatically from intent
// battery temperature in tenths of a degree centigrade
intent?.apply {
val temp = getIntExtra(
BatteryManager.EXTRA_TEMPERATURE,0
)/10F
// show the battery temperate in text view
textView.text = "Battery Temperature\n\n$temp${0x00B0.toChar()}C"
}
}
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// initialize a new intent filter instance
val filter = IntentFilter(Intent.ACTION_BATTERY_CHANGED)
// register the broadcast receiver
registerReceiver(receiver,filter)
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:gravity="center"
android:padding="32dp"
android:textColor="#0014A8"
android:textSize="35sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.25"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
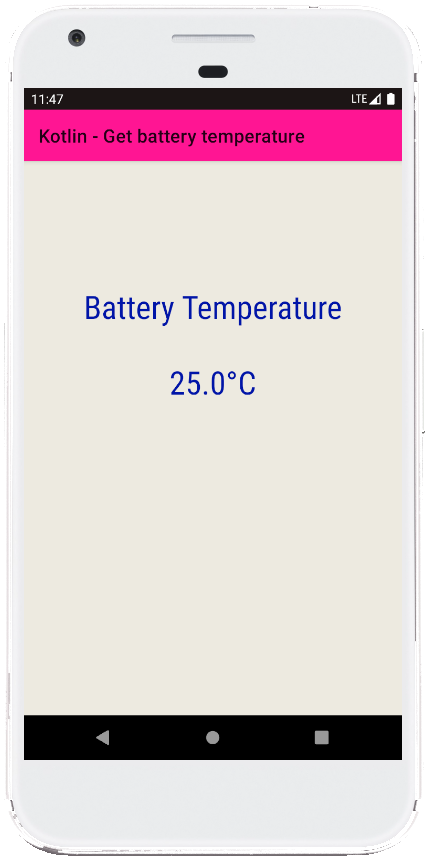
- android kotlin - Switch button listener
- android kotlin - Material switch button
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView rounded corners transparent
- android kotlin - ImageView set image from assets
- android kotlin - ImageView border shadow
- android kotlin - ImageView tint programmatically
- android kotlin - ImageView rounded top corners programmatically
- android kotlin - ImageView set image from Uri
- android kotlin - Get battery capacity programmatically
- android kotlin - Get battery percentage programmatically
- android kotlin - Get battery health programmatically
- android kotlin - Get battery status programmatically
- android kotlin - On back button pressed example
- android kotlin - Get string resource by name