The code demonstrates how to retrieve a string resource by its name in an Android application written in Kotlin. It defines an extension function stringFromResourcesByName
that takes the resource name as a parameter and returns the corresponding string value.
Here's a breakdown of the code:
Import Statements:
- The code starts by importing necessary classes like
Context
,Resources
, etc. These classes provide access to Android's resource framework.
- The code starts by importing necessary classes like
MainActivity Class:
- The
MainActivity
class inherits fromAppCompatActivity
, which is the base class for most activities in Android applications.
- The
onCreate Method:
- The
onCreate
method is the entry point of the activity's lifecycle. It's called when the activity is first created.
- The
Setting Layout:
- Inside the
onCreate
method, thesetContentView
method is used to set the layout file (activity_main.xml
) for the activity.
- Inside the
Get String Resource:
- The following line retrieves the string resource by name:
KotlintextView.text = applicationContext.stringFromResourcesByName("developer")
- Here,
applicationContext.stringFromResourcesByName("developer")
calls the extension function to get the string resource named "developer". The retrieved string value is then assigned to the text property of the TextView with the idtextView
.
stringFromResourcesByName Extension Function:
The
stringFromResourcesByName
function is defined as an extension function of theContext
class. This allows you to call this function on any context object.The function takes a string parameter
resourceName
which represents the name of the string resource to retrieve.Inside the function, it attempts to get the resource ID for the given resource name using
getIdentifier
method. It takes three arguments:- The resource name (
resourceName
) - The resource type (
"string"
in this case) - The package name of the application (
packageName
)
- The resource name (
If the resource is found, the function retrieves the string value using the
getString
method with the resource ID and returns it.If the resource is not found, it catches a
Resources.NotFoundException
and returns null.
Summary:
The provided code snippet showcases a practical approach to fetching string resources by name in an Android Kotlin project. The stringFromResourcesByName
extension function offers a concise way to access string resources from within your activities or other parts of your code. This promotes better code organization and maintainability by separating UI strings from the application logic.
package com.example.jetpack
import android.content.Context
import android.content.res.Resources
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get string from resources by resource name and show it
textView.text = applicationContext
.stringFromResourcesByName("developer")
}
}
// extension function to get a string resource by resource name
fun Context.stringFromResourcesByName(resourceName: String): String? {
return try {
// get the string resource id by name
val resourceId = resources.getIdentifier(
resourceName,
"string",
packageName
)
// return the string value
getString(resourceId)
}catch (e:Resources.NotFoundException){
null
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:fontFamily="sans-serif-condensed"
android:gravity="center"
android:padding="32dp"
android:textColor="#0014A8"
android:textSize="35sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
<resources>
<string name="app_name">Kotlin - Get string resource by name</string>
<string name="developer">Saiful Alam, Khulna, Bangladesh</string>
</resources>
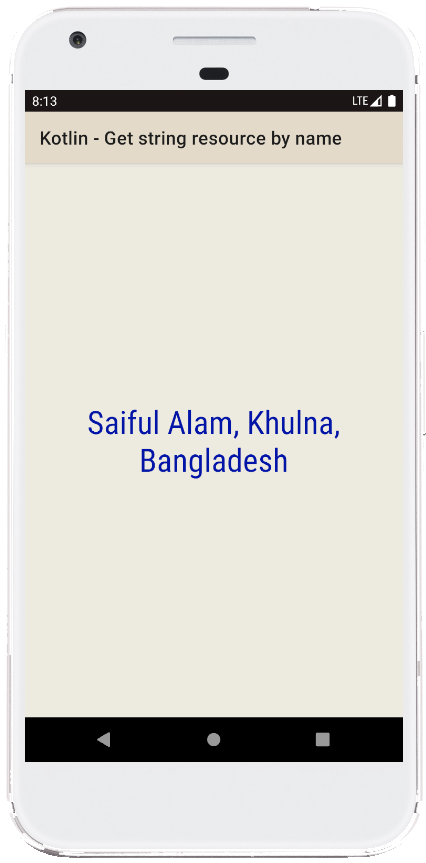
- android kotlin - Get battery temperature programmatically
- android kotlin - Get battery health programmatically
- android kotlin - Get battery status programmatically
- android kotlin - On back button pressed example
- android kotlin - Get screen size category
- android kotlin - Get API level programmatically
- android kotlin - Play default ringtone programmatically
- android kotlin - Canvas draw line
- android kotlin - Canvas draw arc between two points
- android kotlin - Canvas draw path
- android kotlin - Canvas draw multiline text
- android kotlin - Canvas center text