Introduction
This code demonstrates two ways to play the device's default ringtone programmatically in an Android app written in Kotlin. It provides a user interface with two buttons:
- Play Default Ringtone: This button utilizes the
Ringtone
class to play the system's default ringtone. - Play Ringtone As Media: This button plays the ringtone through a
MediaPlayer
instance, treating it as a media resource.
Breakdown of the Code
The code consists of two parts: the MainActivity.kt
file containing the activity logic and the activity_main.xml
file defining the user interface.
MainActivity.kt:
- Imports: Necessary libraries for audio playback, context, and UI components are imported.
- Class Definition: The
MainActivity
class inherits fromAppCompatActivity
and handles the activity lifecycle. - onCreate: This method is called when the activity is first created. Here:
- The layout (
activity_main.xml
) is inflated. - Two extension properties are defined:
defaultRingtone
: Retrieves the system's default ringtone as aRingtone
object.defaultRingtonePlayer
: Creates aMediaPlayer
instance configured with the default ringtone URI.
- Button click listeners are set:
- Button 1 (
button
) plays the ringtone using thedefaultRingtone
property and stops it on a long press. - Button 2 (
button2
) plays the ringtone using thedefaultRingtonePlayer
and stops it on a long press.
- Button 1 (
- The layout (
Extension Properties:
defaultRingtone
:- Gets the default ringtone URI using
RingtoneManager
. - Creates a
Ringtone
object with the obtained URI.
- Gets the default ringtone URI using
defaultRingtonePlayer
:- Retrieves the default ringtone URI from
Settings.System
. - Creates a
MediaPlayer
instance with the URI, effectively treating the ringtone as media.
- Retrieves the default ringtone URI from
activity_main.xml:
- Defines the activity layout with two buttons:
- Button 1: Triggers playing the ringtone using the
Ringtone
class. - Button 2: Triggers playing the ringtone using the
MediaPlayer
instance.
- Button 1: Triggers playing the ringtone using the
Summary
This code provides a flexible approach for playing the device's default ringtone. It demonstrates using both the Ringtone
class for standard ringtone playing and the MediaPlayer
class for more granular control over playback. The extension properties offer a convenient way to access the default ringtone throughout the activity.
package com.example.jetpack
import android.content.Context
import android.media.MediaPlayer
import android.media.Ringtone
import android.media.RingtoneManager
import android.os.Bundle
import android.provider.Settings
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get device default ringtone and play
val ringtone = applicationContext.defaultRingtone
button.setOnClickListener {
ringtone.play()
}
// stop playing ringtone
button.setOnLongClickListener {
ringtone.stop()
true
}
// play default ringtone as media
val player = applicationContext.defaultRingtonePlayer
button2.setOnClickListener {
player.start()
}
// stop media player
button2.setOnLongClickListener {
player.stop()
true
}
}
}
// extension property to get device default ringtone
val Context.defaultRingtone:Ringtone
get() {
val uri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_RINGTONE)
return RingtoneManager.getRingtone(this,uri)
}
// extension property to get media player with default ringtone
val Context.defaultRingtonePlayer:MediaPlayer
get() {
// get default ringtone uri
val uri = Settings.System.DEFAULT_RINGTONE_URI
// create media player with default ringtone
return MediaPlayer.create(this, uri)
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#E5E4E2"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Play Default Ringtone"
android:backgroundTint="#00563F"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Play Ringtone As Media"
android:backgroundTint="#7B3F00"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button" />
</androidx.constraintlayout.widget.ConstraintLayout>
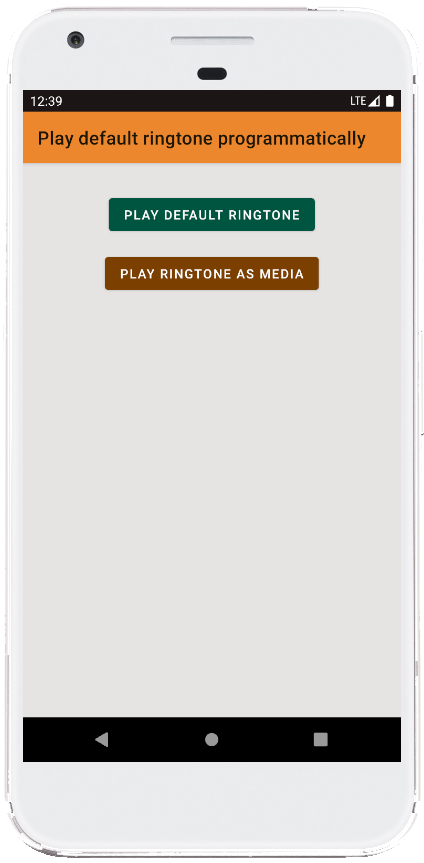
- android kotlin - EditText allow only numbers
- android kotlin - EditText limit number range
- android kotlin - EditText input filter decimal
- android kotlin - EditText remove underline while typing
- android kotlin - EditText change cursor color programmatically
- android kotlin - EditText hide keyboard after enter
- android kotlin - EditText hide keyboard click outside
- android kotlin - Switch button listener
- android kotlin - Material switch button
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView rounded corners transparent
- android kotlin - Repeat a task periodically
- android kotlin - Do a task after a delay
- android kotlin - Get screen size category
- android kotlin - Get API level programmatically