Introduction
This code demonstrates how to programmatically create an ImageView and add it to a ConstraintLayout in Android. An ImageView is a fundamental view for displaying images and can be used in various layouts.
Explanation
-
Import Statements: The code begins by importing essential libraries like Context, android.graphics, Bundle, View, ImageView, AppCompatActivity, ConstraintLayout, etc. These libraries provide the functionalities used throughout the program.
-
MainActivity Class: The MainActivity class inherits from AppCompatActivity, which serves as the foundation class for most activities in Android. The onCreate() method acts as the entry point of the activity and is invoked when the activity is first created.
-
Create ImageView Programmatically: Inside the onCreate() method, an ImageView is constructed programmatically using the applicationContext.createImageView() extension function. This function generates an ImageView instance, sets its layout parameters, and assigns it an id.
-
Add ImageView to ConstraintLayout: The newly created ImageView is then incorporated into the constraint layout using the constraintLayout.addViewTop(context, view) extension function. This function not only adds the view but also establishes constraints using ConstraintSet to position the ImageView at the top of the parent layout with a 10dp margin and centered horizontally.
-
Set Image from Assets: The setImageBitmap(assetsToBitmap("flowers5.jpg")) method is employed to set the image from the assets folder. The assetsToBitmap() extension function retrieves the specified image file from the assets folder and converts it into a Bitmap object.
-
Set Image Scale Type: The adjustViewBounds property is set to true, and the scaleType property is set to ImageView.ScaleType.CENTER_CROP to guarantee that the image is displayed within the ImageView's boundaries.
-
Extension Functions: The code also defines three extension functions: createImageView(), addViewTop(), and assetsToBitmap(). These functions enhance code readability and reusability.
Summary
This code example showcases how to programmatically create an ImageView, add it to a ConstraintLayout, and display an image from the assets folder. By leveraging extension functions, the code maintains readability and promotes reusability.
Table of Extension Functions
Function Name | Description |
---|---|
createImageView() | Creates an ImageView programmatically, sets its layout parameters, and assigns it an id. |
addViewTop(context, view) | Adds a view to the top of a ConstraintLayout and sets constraints to position it. |
assetsToBitmap(fileName) | Retrieves an image from the assets folder and converts it into a Bitmap object. |
MainActivity.kt
package com.example.jetpack
import android.content.Context
import android.graphics.Bitmap
import android.graphics.BitmapFactory
import android.os.Bundle
import android.util.TypedValue
import android.view.View
import android.widget.ImageView
import androidx.appcompat.app.AppCompatActivity
import androidx.constraintlayout.widget.ConstraintLayout
import androidx.constraintlayout.widget.ConstraintLayout.LayoutParams.PARENT_ID
import androidx.constraintlayout.widget.ConstraintSet
import kotlinx.android.synthetic.main.activity_main.*
import java.io.IOException
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// create an image view programmatically and add to layout
applicationContext.createImageView().apply {
// add image view to constraint layout
constraintLayout.addViewTop(applicationContext,this)
// get bitmap from assets folder
setImageBitmap(assetsToBitmap("flowers5.jpg"))
// set image scale type
adjustViewBounds = true
scaleType = ImageView.ScaleType.CENTER_CROP
}
}
}
// extension function to create an image view programmatically
fun Context.createImageView(): ImageView {
return ImageView(this).apply {
layoutParams = ConstraintLayout.LayoutParams(
ConstraintLayout.LayoutParams.MATCH_PARENT, // width
//ConstraintLayout.LayoutParams.WRAP_CONTENT // height
285.dpToPixels(context) // height
)
id = View.generateViewId()
}
}
// extension function to add view top of constraint layout
fun ConstraintLayout.addViewTop(context: Context, view: View){
addView(view)
val set = ConstraintSet()
set.clone(this)
// connect view start with parent start
set.connect(
view.id,ConstraintSet.START,
PARENT_ID,ConstraintSet.START,
5.dpToPixels(context) // margin
)
// connect view top with parent top
set.connect(
view.id, ConstraintSet.TOP,
PARENT_ID,ConstraintSet.TOP,
10.dpToPixels(context) // margin
)
// connect view end with parent end
set.connect(
view.id, ConstraintSet.END,
PARENT_ID,ConstraintSet.END,
5.dpToPixels(context) // margin
)
set.applyTo(this)
}
// extension function to get bitmap from assets
fun Context.assetsToBitmap(fileName: String): Bitmap?{
return try {
with(assets.open(fileName)){
BitmapFactory.decodeStream(this)
}
} catch (e: IOException) { null }
}
// extension function to convert dp to equivalent pixels
fun Int.dpToPixels(context: Context):Int = TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP, this.toFloat(), context.resources.displayMetrics
).toInt()
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity" />
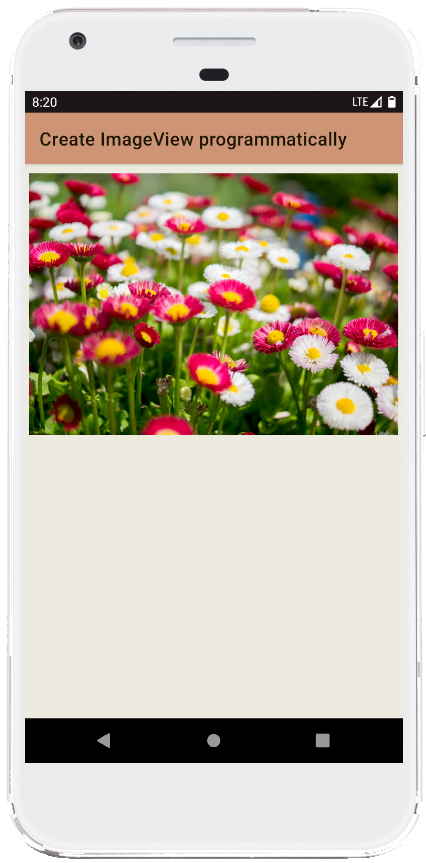
- android kotlin - ImageView rounded corners transparent
- android kotlin - ImageView circle crop
- android kotlin - Circular ImageView with border
- android kotlin - Circular ImageView programmatically
- android kotlin - ImageView border radius
- android kotlin - ImageView add border programmatically
- android kotlin - ImageView add border
- android kotlin - ImageView rounded corners programmatically
- android kotlin - ImageView set image from drawable
- android kotlin - ImageView set image from url
- android kotlin - Get battery percentage programmatically
- android kotlin - Get battery level programmatically
- android kotlin - Get battery voltage programmatically
- android kotlin - On back button pressed example
- android kotlin - Get string resource by name