MainActivity.kt
package com.example.jetpack
import android.content.Context
import android.graphics.*
import android.os.Bundle
import android.util.TypedValue
import androidx.appcompat.app.AppCompatActivity
import androidx.core.graphics.drawable.RoundedBitmapDrawable
import androidx.core.graphics.drawable.RoundedBitmapDrawableFactory
import kotlinx.android.synthetic.main.activity_main.*
import java.io.IOException
import kotlin.math.min
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get bitmap from assets folder
val bitmap: Bitmap? = assetsToBitmap("flower7.jpg")
// make the bitmap circular shape with maximum available size
bitmap?.circularIt(applicationContext)?.apply {
imageView.setImageDrawable(this)
}
// make the bitmap circular with specified diameter
bitmap?.circularIt(
context = applicationContext,
// make it smaller
200.dpToPixels(applicationContext)
)?.apply {
imageView2.setImageDrawable(this)
}
}
}
// extension function to make a bitmap circular
fun Bitmap.circularIt(
context: Context,
diameter:Int = min(width,height)
): RoundedBitmapDrawable? {
// Calculate the bitmap diameter and radius
val length = min(diameter,min(width,height))
val radius = length / 2F
val bitmap = Bitmap.createBitmap(length,length,Bitmap.Config.ARGB_8888)
val canvas = Canvas(bitmap)
canvas.drawColor(Color.WHITE)
// draw bitmap at canvas center
canvas.drawBitmap(
this, // bitmap
(diameter - this.width)/2F, // left
(diameter - this.height)/2F, // top
null // paint
)
// return the rounded bitmap drawable as circle shape
return RoundedBitmapDrawableFactory.create(context.resources,bitmap).apply {
isCircular = true
setAntiAlias(true)
}
}
// extension function to convert dp to equivalent pixels
fun Int.dpToPixels(context: Context):Int = TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP, this.toFloat(), context.resources.displayMetrics
).toInt()
// extension function to get bitmap from assets
fun Context.assetsToBitmap(fileName: String): Bitmap?{
return try {
with(assets.open(fileName)){
BitmapFactory.decodeStream(this)
}
} catch (e: IOException) { null }
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#DCDCDC"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="280dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@tools:sample/avatars" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="0dp"
android:layout_height="280dp"
android:layout_marginStart="8dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView"
tools:srcCompat="@tools:sample/avatars" />
</androidx.constraintlayout.widget.ConstraintLayout>
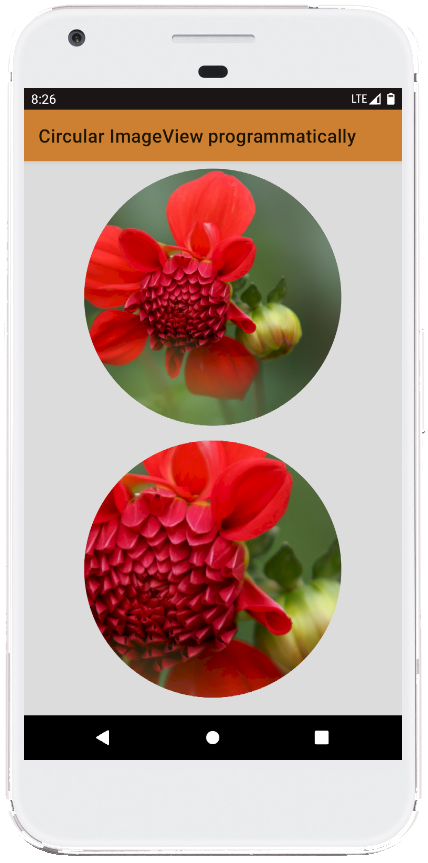
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView rounded corners transparent
- android kotlin - ImageView circle crop
- android kotlin - Circular ImageView with border
- android kotlin - ImageView rounded corners programmatically
- android kotlin - ImageView set image from drawable
- android kotlin - ImageView set image from url
- android kotlin - Get screen size category
- android kotlin - Get API level programmatically
- android kotlin - Play default ringtone programmatically
- android kotlin - Get screen size programmatically
- android kotlin - RecyclerView animation
- android kotlin - RecyclerView smooth scroll
- android kotlin - Set CardView corner radius
- android kotlin - RadioButton with image and text