This code demonstrates two ways to set the corner radius of a CardView in an Android application written in Kotlin. The first method uses the app:cardCornerRadius
attribute directly in the layout XML file. The second method sets the corner radius programmatically in the activity code.
The code includes a MainActivity
class and the corresponding activity_main.xml
layout file. An additional snippet for the build.gradle
file (assuming it's located in the app
module) is required to specify the CardView library dependency.
Breakdown of the Code:
MainActivity.kt:
- Imports: Necessary libraries are imported, including
androidx.appcompat.app.AppCompatActivity
for the base activity class and the Kotlin synthetic imports for accessing views defined in the layout file. - onCreate method: This method is called when the activity is first created.
- Sets the content view of the activity to
activity_main.xml
. - Sets the corner radius of the second CardView with the ID
cardViewBottom
programmatically using theradius
property and a custom extension functiondpToPixels
to convert dp values to pixels.
- Sets the content view of the activity to
activity_main.xml:
- Defines the root layout as a
ConstraintLayout
. - Includes two
CardView
elements:- The first card has its corner radius set directly in the layout using the
app:cardCornerRadius
attribute. - The second card's ID is set to
cardViewBottom
.
- The first card has its corner radius set directly in the layout using the
dpToPixels extension function:
- This function converts a dp (density-independent pixel) value to its equivalent pixel size based on the device's display metrics.
build.gradle (app):
- (Code not provided, but assumed to be here)
- This file should include the dependency for the CardView library:
implementation "androidx.cardview:cardview:1.0.0"
Summary
This code provides a clear example of setting CardView corner radius in both XML and Kotlin. By combining these methods, you can achieve dynamic control over the appearance of your cards within your Android application.
MainActivity.kt
package com.example.jetpack
import android.content.Context
import android.os.Bundle
import android.util.TypedValue
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// set second card view corner radius programmatically
cardViewBottom.radius = 12.dpToPixels(this)
}
}
// extension function to convert dp to equivalent pixels
fun Int.dpToPixels(context: Context):Float = TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP,this.toFloat(),context.resources.displayMetrics
)
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:background="#EDEAE0">
<androidx.cardview.widget.CardView
android:id="@+id/cardView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
app:cardBackgroundColor="#E52B50"
app:cardCornerRadius="12dp"
app:cardElevation="4dp"
app:cardMaxElevation="6dp"
app:contentPadding="25dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:gravity="center"
android:padding="30dp"
android:text="CardView Corner Radius In XML"
android:textSize="30sp" />
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/cardViewBottom"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
app:cardBackgroundColor="#7BB661"
app:cardElevation="4dp"
app:cardMaxElevation="6dp"
app:contentPadding="25dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/cardView">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="30dp"
android:text="CardView Corner Radius Programmatically"
android:textSize="30sp" />
</androidx.cardview.widget.CardView>
</androidx.constraintlayout.widget.ConstraintLayout>
build.gradle(app) [code to add]
dependencies {
// card view
implementation "androidx.cardview:cardview:1.0.0"
}
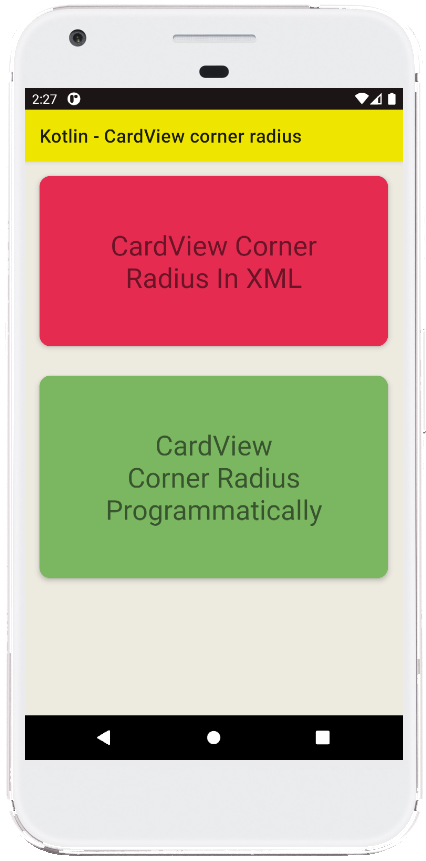
- android kotlin - Repeat a task periodically
- android kotlin - Get app first install time
- android kotlin - Get app version name and code
- android kotlin - Detect screen orientation change
- android kotlin - Get screen orientation programmatically
- android kotlin - Convert dp to pixels programmatically
- android kotlin - Get screen size from context
- android kotlin - RecyclerView horizontal
- android kotlin - RecyclerView divider line
- android kotlin - GridView item height
- android kotlin - GridView add item dynamically
- android kotlin - CheckBox checked change listener
- android kotlin - Set CardView elevation
- android kotlin - Set CardView background color
- android kotlin - RadioButton with image and text