Creating a Horizontal Scrolling List with RecyclerView in Android (Kotlin)
This code demonstrates how to create a horizontally scrolling list of items using RecyclerView in an Android application written in Kotlin.
The code is divided into three parts:
- MainActivity.kt: This file defines the main activity of the application. It retrieves data (a list of animal names), sets up the RecyclerView with a horizontal layout manager, and binds the data to a custom adapter class.
- RecyclerViewAdapter.kt: This file defines the adapter class for the RecyclerView. It inflates a custom view for each item, binds the data to the view, and manages the number of items in the list.
- Layout XML files: These files define the layouts for the main activity (
activity_main.xml
) and the custom view for each item (custom_view.xml
).
Summary
By combining these components, the code creates a RecyclerView that displays a list of animal names horizontally. Users can scroll through the list to see all the items. This approach is useful for presenting collections of data where horizontal browsing is preferred.
MainActivity.kt
package com.cfsuman.kotlintutorials
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
class MainActivity : AppCompatActivity() {
private lateinit var context:MainActivity
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Get the context
context = this;
// Get the widgets reference from XML layout
val recyclerView = findViewById<RecyclerView>(R.id.recyclerView)
// initialize a mutable list of animals
val animals = mutableListOf(
"Horse", "Donkey", "Goat",
"Squirrel", "Mouse", "Chameleon",
"Deer", "Raccoon", "Antelope",
"Beaver", "Weasel"
)
// initialize an instance of linear layout manager
val layoutManager = LinearLayoutManager(
context, // context
RecyclerView.HORIZONTAL, // orientation
false // reverse layout
).apply {
// specify the layout manager for recycler view
recyclerView.layoutManager = this
}
// finally, data bind the recycler view with adapter
RecyclerViewAdapter(animals).apply {
recyclerView.adapter = this
}
}
}
RecyclerViewAdapter.kt
package com.cfsuman.kotlintutorials
import android.view.LayoutInflater
import android.view.View
import android.view.ViewGroup
import android.widget.TextView
import androidx.recyclerview.widget.RecyclerView
class RecyclerViewAdapter(private val animals: MutableList<String>)
: RecyclerView.Adapter<RecyclerViewAdapter.ViewHolder>() {
override fun onCreateViewHolder(
parent: ViewGroup, viewType: Int): ViewHolder {
// inflate the custom view from xml layout file
val view: View = LayoutInflater.from(parent.context)
.inflate(R.layout.custom_view,parent,false)
// return the view holder
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
// display the current animal
holder.animal.text = animals[position]
}
override fun getItemCount(): Int {
// number of items in the data set held by the adapter
return animals.size
}
class ViewHolder(itemView: View)
: RecyclerView.ViewHolder(itemView){
val animal: TextView = itemView.findViewById(R.id.tvAnimal)
}
// this two methods useful for avoiding duplicate item
override fun getItemId(position: Int): Long {
return position.toLong()
}
override fun getItemViewType(position: Int): Int {
return position
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rootLayout"
android:background="#DCDCDC"
android:padding="8dp">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
custom_view.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/cardView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:cardBackgroundColor="#F8F8F8"
app:cardCornerRadius="8dp"
app:cardElevation="4dp"
app:cardMaxElevation="6dp"
app:contentPadding="24dp"
android:layout_margin="4dp">
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/tvAnimal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:fontFamily="sans-serif"
android:textSize="24sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
</androidx.cardview.widget.CardView>
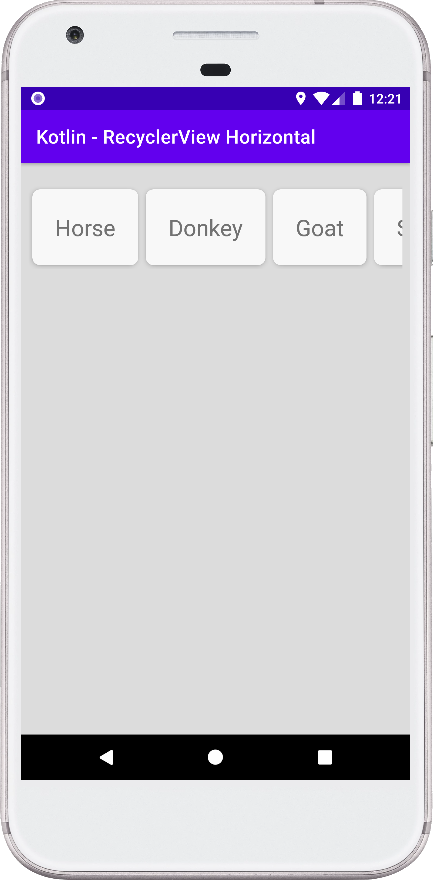

- android kotlin - Switch button listener
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView set image from assets
- android kotlin - ImageView tint programmatically
- android kotlin - ImageView set image from Uri
- android kotlin - Get battery temperature programmatically
- android kotlin - Repeat a task periodically
- android kotlin - Get app first install time
- android kotlin - Detect screen orientation change
- android kotlin - RecyclerView animation
- android kotlin - RecyclerView smooth scroll
- android kotlin - RecyclerView divider line
- android kotlin - RecyclerView add remove item
- android kotlin - RecyclerView GridLayoutManager example
- android kotlin - RecyclerView StaggeredGridLayoutManager example