Introduction
This code snippet showcases how to retrieve information about an installed application on an Android device using Kotlin programming language. The retrieved information includes the application's package name, version name, and version code.
Explanation
Import Statements:
The code imports necessary packages for working with Android UI components and accessing package information.
android.content.pm.PackageInfo
provides classes to represent information about an installed package.androidx.appcompat.app.AppCompatActivity
is the base class for activities in the AndroidX library.androidx.core.content.pm.PackageInfoCompat
offers utility methods for working withPackageInfo
.kotlinx.android.synthetic.main
enables the use of synthetic imports for views defined in the layout XML file.
MainActivity Class:
This class inherits from
AppCompatActivity
and represents the main activity of the application.onCreate Method:
This method is called when the activity is first created.
Retrieve Package Information:
- The code gets an instance of
PackageManager
usingpackageManager
. - It then calls
getPackageInfo
on thePackageManager
instance, passing the app's package name (packageName
) and a flag set to 0 to retrieve basic information. - The retrieved information is stored in the
packageInfo
variable of typePackageInfo
.
- The code gets an instance of
Extract App Version Details:
- The app's version name is extracted from the
packageInfo
object usingversionName
and stored in theversionName
string variable. - Similarly, the app's version code is retrieved using
PackageInfoCompat.getLongVersionCode
and assigned to theversionCode
long variable.
- The app's version name is extracted from the
Display Information:
- The collected details (package name, version name, and version code) are displayed in the TextView with the ID
textView
. - The text is appended line by line using the
append
method.
- The collected details (package name, version name, and version code) are displayed in the TextView with the ID
Summary
By running this code, the application retrieves and displays the installed app's package name, version name, and version code on the main screen.
Table: Summary of Retrieved Information
Information | Description |
---|---|
Package Name | The unique identifier of the application package. |
Version Name | The human-readable version name of the application. |
Version Code | The internal version number of the application (typically an integer). |
package com.example.jetpack
import android.content.pm.PackageInfo
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.core.content.pm.PackageInfoCompat
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the package info instance
val packageInfo: PackageInfo = packageManager.getPackageInfo(packageName, 0)
// get this app version name programmatically
val versionName: String = packageInfo.versionName
// get this app version code programmatically
val versionCode: Long = PackageInfoCompat.getLongVersionCode(packageInfo)
// display the collected information in text view
textView.text = "Package Name: $packageName"
textView.append("\nVersion Name: $versionName")
textView.append("\nVersion Code : $versionCode")
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#E5E4E2"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
android:fontFamily="sans-serif-condensed"
android:textColor="#191970"
android:textSize="25sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
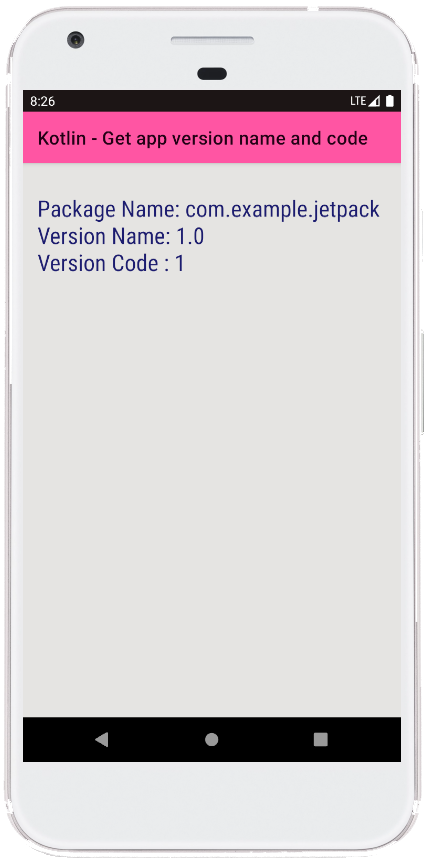
- android kotlin - Get app first install time
- android kotlin - Enable disable bluetooth programmatically
- android kotlin - Change screen orientation programmatically
- android kotlin - Change orientation without restarting activity
- android kotlin - Get screen width and height in dp
- android kotlin - Get screen density programmatically
- android kotlin - Convert pixels to dp programmatically
- android kotlin - Get screen size programmatically
- android kotlin - RecyclerView animation
- android kotlin - RecyclerView smooth scroll
- android kotlin - RecyclerView add remove item
- android kotlin - RecyclerView GridLayoutManager example
- android kotlin - RecyclerView StaggeredGridLayoutManager example
- android kotlin - Border/divider between GridView items
- android kotlin - GridView OnItemClickListener