Android Kotlin - How to show/add border/divider between GridView items
This code demonstrates how to create a simple GridView with borders between each item in Kotlin for Android development.
The code is divided into two parts:
- MainActivity.kt: This file handles the logic and data population for the GridView.
- activity_main.xml: This file defines the layout for the activity, including the GridView and a TextView.
Breakdown of MainActivity.kt:
- The
onCreate
function sets up the GridView by:- Defining a list of plant names.
- Creating an
ArrayAdapter
for the GridView that customizes the view for each item.- The custom view sets the text, centers it, and sets the background color.
- Setting a click listener for the GridView items to display the selected item text in a separate TextView.
Key points for creating borders:
- The borders are created by setting the background color of each GridView item along with the
horizontalSpacing
andverticalSpacing
properties of the GridView itself.- These spacings define the gaps between items and visually create the border effect.
Summary
This example provides a basic implementation of a GridView with borders between items using Kotlin. It demonstrates how to populate the GridView with data, customize the view for each item, and handle item clicks. While a more complex approach might involve custom drawables for borders, this method offers a simple solution using readily available properties.
MainActivity.kt
package com.example.jetpack
import android.graphics.Color
import android.os.Bundle
import android.view.Gravity
import android.view.View
import android.view.ViewGroup
import android.widget.ArrayAdapter
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// list of plants to populate grid view
val list = listOf(
"Catalina ironwood",
"Cabinet cherry",
"Pale corydalis",
"Pink corydalis",
"Belle Isle cress",
"Land cress",
"Cutleaf coneflower",
"Coast polypody"
)
// array adapter for grid view
gridView.adapter = object : ArrayAdapter<String>(this,
android.R.layout.simple_list_item_1,list){
override fun getView(position: Int, convertView: View?,
parent: ViewGroup): View {
return (super.getView(position, convertView, parent) as TextView)
.apply {
text = list[position]
gravity = Gravity.CENTER
// grid view vertical spacing, horizontal spacing
// and item background color together make border between items
setBackgroundColor(Color.parseColor("#C5E384"))
}
}
}
// set grid view item click listener
gridView.setOnItemClickListener { adapterView, view, i, l ->
// get grid view clicked item text
val selectedItem = adapterView.getItemAtPosition(i).toString()
textView.text = selectedItem
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:background="#EDEAE0">
<GridView
android:id="@+id/gridView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="8dp"
android:background="#9ACD32"
android:numColumns="2"
android:horizontalSpacing="3dp"
android:verticalSpacing="3dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:textColor="#004F98"
android:textSize="30sp"
android:fontFamily="sans-serif-condensed-medium"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/gridView"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
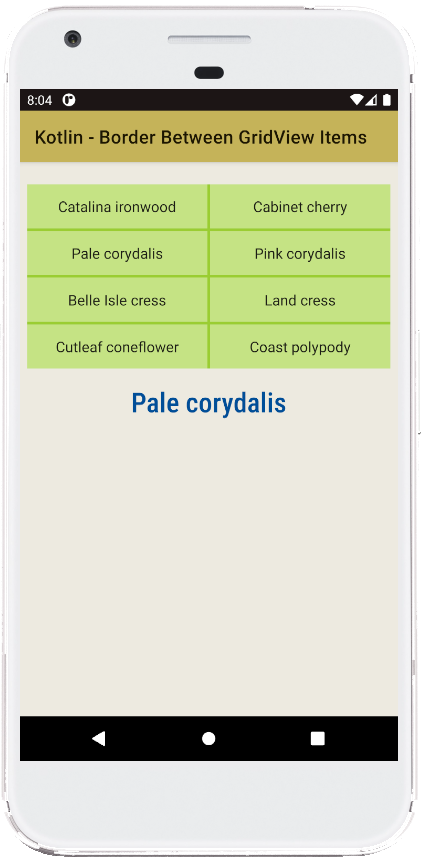
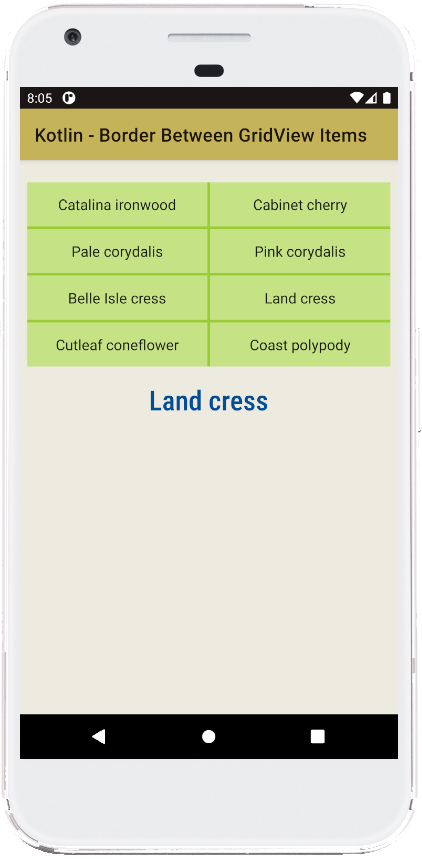
- android kotlin - GridView item height
- android kotlin - GridView add item dynamically
- android kotlin - GridView selected item background color
- android kotlin - GridView OnItemClickListener
- android kotlin - Set CardView background color
- android kotlin - Set CardView corner radius
- android kotlin - RadioButton with image and text
- android kotlin - Canvas draw line
- android kotlin - Canvas draw arc between two points
- android kotlin - Canvas draw path
- android kotlin - Canvas draw arc
- android kotlin - Canvas draw triangle
- android kotlin - Canvas draw text rotate
- android kotlin - Canvas draw text inside circle
- android kotlin - Canvas draw text wrap