Introduction
This code snippet demonstrates how to retrieve the screen size of an Android device in dp (density-independent pixels) using Kotlin. Dp is a unit that ensures consistent sizing across devices with varying resolutions. It is ideal for designing layouts that adapt to different screen sizes.
Breakdown of the Code
Activity Class (MainActivity.kt):
- This class represents the main activity of the Android application.
- The
onCreate()
method is called when the activity is first created. - In this method, the following functionalities are implemented:
- The layout file (activity_main.xml) is inflated using
setContentView
. - A text view (
textView
) is used to display the screen size information. - Two extension properties are utilized to retrieve screen size data:
displayMetrics
: This property retrieves an instance ofDisplayMetrics
, a class that provides details about the display, including its size, density, and font scaling.screenSizeInDp
: This property calculates the screen width and height in dp using thedisplayMetrics
and stores the values in aPoint
object.
- The layout file (activity_main.xml) is inflated using
- The screen size information (width and height in dp) is then appended to the text view's content.
Extension Properties (MainActivity.kt):
- The code defines two extension properties for the
Activity
class:displayMetrics
: This property retrieves theDisplayMetrics
instance using the appropriate method based on the Android API level.- For API levels below 30, it uses
windowManager.defaultDisplay.getMetrics(displayMetrics)
. - For API levels 30 and above, it leverages
display.getRealMetrics(displayMetrics)
.
- For API levels below 30, it uses
screenSizeInDp
: This property calculates the screen width and height in dp. It first retrieves the display metrics and then divides the width and height in pixels by the display density. The result is rounded to the nearest integer usingroundToInt()
and stored in aPoint
object.
- The code defines two extension properties for the
Layout File (activity_main.xml):
- This file defines the layout of the main activity screen.
- It contains a single text view (
textView
) that will be used to display the screen size information retrieved from the Kotlin code.
Summary
By combining the functionalities of the activity class, extension properties, and layout file, this code effectively retrieves and displays the screen size of the Android device in dp. This approach ensures that the layout adapts to different screen resolutions while maintaining consistent sizing.
Here is a table summarizing the key points of the code:
Property/Method | Description |
---|---|
displayMetrics | Extension property to get an instance of DisplayMetrics |
screenSizeInDp | Extension property to calculate the screen width and height in dp |
onCreate() | Method called when the activity is first created |
textView | Text view to display the screen size information |
package com.example.jetpack
import android.app.Activity
import android.graphics.Point
import android.os.Build
import android.os.Bundle
import android.util.DisplayMetrics
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
import kotlin.math.roundToInt
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// show screen size in dp
textView.text = "Screen size in dp"
screenSizeInDp.apply {
// screen width in dp
textView.append("\n\nWidth : $x dp")
// screen height in dp
textView.append("\nHeight : $y dp")
}
}
}
// extension property to get display metrics instance
val Activity.displayMetrics: DisplayMetrics
get() {
// display metrics is a structure describing general information
// about a display, such as its size, density, and font scaling
val displayMetrics = DisplayMetrics()
if (Build.VERSION.SDK_INT >= 30){
display?.apply {
getRealMetrics(displayMetrics)
}
}else{
// getMetrics() method was deprecated in api level 30
windowManager.defaultDisplay.getMetrics(displayMetrics)
}
return displayMetrics
}
// extension property to get screen width and height in dp
val Activity.screenSizeInDp: Point
get() {
val point = Point()
displayMetrics.apply {
// screen width in dp
point.x = (widthPixels / density).roundToInt()
// screen height in dp
point.y = (heightPixels / density).roundToInt()
}
return point
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:background="#E5E4E2">
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="16dp"
tools:text="TextView"
android:fontFamily="sans-serif-condensed"
android:textSize="30sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
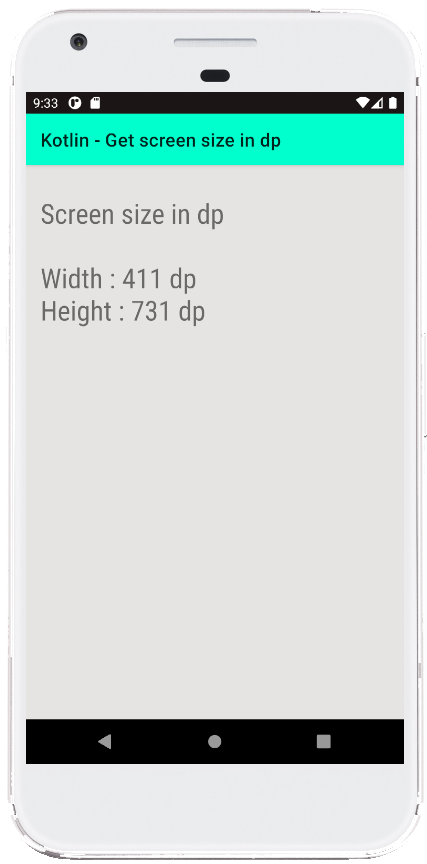
- android kotlin - EditText allow only numbers
- android kotlin - EditText allow only characters
- android kotlin - EditText hide keyboard after enter
- android kotlin - EditText hide keyboard click outside
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView rounded corners transparent
- android kotlin - ImageView tint programmatically
- android kotlin - ImageView rounded top corners programmatically
- android kotlin - Detect screen orientation change
- android kotlin - Get screen orientation programmatically
- android kotlin - Get screen density programmatically
- android kotlin - Convert pixels to dp programmatically
- android kotlin - Get screen size programmatically
- android kotlin - RecyclerView animation
- android kotlin - RecyclerView smooth scroll