MainActivity.kt
package com.example.jetpack
import android.content.Context
import android.graphics.*
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
import java.io.IOException
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get bitmap from assets
val bitmap: Bitmap? = assetsToBitmap("flower.jpg")
// set the image view image from assets
bitmap?.apply {
imageView.setImageBitmap(this)
}
// make the bitmap rounded top corners
button.setOnClickListener {
bitmap?.toRoundedCorners(
topLeftRadius = 150F,
topRightRadius = 150F
)?.apply {
// show the rounded top corners bitmap in image view
imageView.setImageBitmap(this)
}
}
}
}
// extension function to make rounded corners bitmap
fun Bitmap.toRoundedCorners(
topLeftRadius: Float = 0F,
topRightRadius: Float = 0F,
bottomRightRadius: Float = 0F,
bottomLeftRadius: Float = 0F
):Bitmap?{
val bitmap = Bitmap.createBitmap(
width, // width in pixels
height, // height in pixels
Bitmap.Config.ARGB_8888
)
val canvas = Canvas(bitmap)
// the bounds of a round-rectangle to add to the path
val rectF = RectF(0f,0f,width.toFloat(),height.toFloat())
// float array of 8 values, 4 pairs of [x,y] radii
val radii = floatArrayOf(
topLeftRadius,topLeftRadius, // top left corner
topRightRadius,topRightRadius, // top right corner
bottomRightRadius,bottomRightRadius, // bottom right corner
bottomLeftRadius,bottomLeftRadius // bottom left corner
)
// path to draw rounded corners bitmap
val path = Path().apply {
// add a closed round-rectangle contour to the path
// each corner receives two radius values [x, y]
addRoundRect(
rectF,
radii,
// the direction to wind the round-rectangle's contour
Path.Direction.CCW
)
}
// intersect the current clip with the specified path
canvas.clipPath(path)
// draw the rounded corners bitmap on canvas
canvas.drawBitmap(this,0f,0f,null)
return bitmap
}
// extension function to get bitmap from assets
fun Context.assetsToBitmap(fileName:String): Bitmap?{
return try {
with(assets.open(fileName)){
BitmapFactory.decodeStream(this)
}
} catch (e: IOException) { null }
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
android:text="Round Top Corners"
android:backgroundTint="#333399"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="250dp"
android:layout_marginStart="8dp"
android:layout_marginTop="12dp"
android:layout_marginEnd="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
tools:srcCompat="@tools:sample/avatars" />
</androidx.constraintlayout.widget.ConstraintLayout>
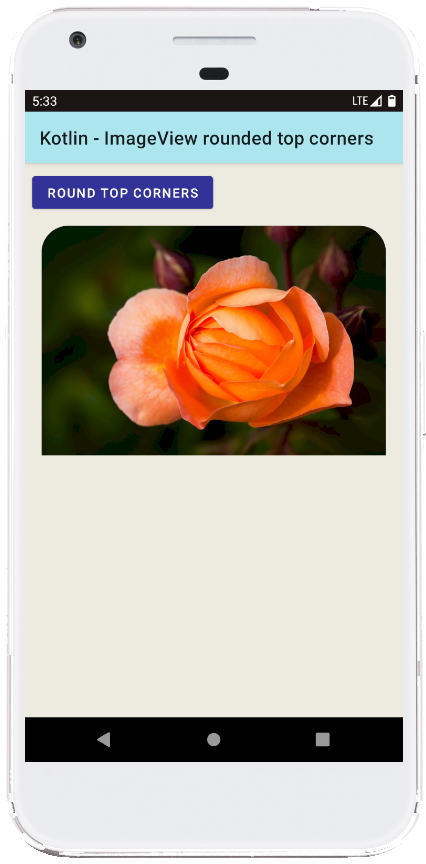
- android kotlin - Switch button listener
- android kotlin - Material switch button
- android kotlin - Create ImageView programmatically
- android kotlin - ImageView rounded corners transparent
- android kotlin - ImageView circle crop
- android kotlin - ImageView set image from assets
- android kotlin - ImageView border shadow
- android kotlin - ImageView border radius
- android kotlin - ImageView tint programmatically
- android kotlin - ImageView rounded corners programmatically
- android kotlin - ImageView set image from drawable
- android kotlin - ImageView set image from url
- android kotlin - Get battery percentage programmatically
- android kotlin - Get battery level programmatically
- android kotlin - Get battery voltage programmatically