Android Kotlin - Implementing RadioButton Click Event
This code demonstrates how to handle click events on RadioButtons in an Android application written in Kotlin. It creates a simple activity with a question, a group of RadioButtons representing different options, and a TextView to display the user's selection.
Breakdown:
MainActivity.kt:
- This file defines the main activity of the application.
- The
onCreate
method sets the layout for the activity (activity_main.xml
). - The
onRadioButtonClicked
function handles clicks on any RadioButton within the RadioGroup.- It uses a
when
statement to identify the clicked RadioButton based on its ID. - Depending on the ID, it updates the text of
textView2
to display a message indicating which option was selected.
- It uses a
activity_main.xml:
- This file defines the layout for the activity.
- It uses a ConstraintLayout to position the UI elements.
- A TextView displays a question to the user.
- A RadioGroup contains several MaterialRadioButtons representing the available options. Each RadioButton has an
onClick
attribute referencing theonRadioButtonClicked
function in the activity class. - Finally, another TextView displays the user's selected option.
Summary:
This code provides a basic example of handling RadioButton clicks in Kotlin. By using a when
statement and checking the ID of the clicked view, the application can determine which option the user selected and update the UI accordingly. This approach allows developers to create user interfaces where users can choose one option from a set of mutually exclusive choices.
MainActivity.kt
package com.example.jetpack
import android.os.Bundle
import android.view.View
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
fun onRadioButtonClicked(view: View) {
when(view.id){
R.id.radioFlash ->{
textView2.text = "Flash clicked."
}R.id.radioFlex ->{
textView2.text = "Flex clicked."
}R.id.radioColdFusion->{
textView2.text = "ColdFusion clicked."
}R.id.radioPhotoshop->{
textView2.text = "Photoshop clicked."
}
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:background="#F5F5F5">
<TextView
android:id="@+id/textView"
style="@style/TextAppearance.MaterialComponents.Headline6"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:padding="8dp"
android:text="Which is your most favorite?"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<RadioGroup
android:id="@+id/radioGroup"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="32dp"
android:layout_marginTop="2dp"
android:layout_marginEnd="16dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView">
<com.google.android.material.radiobutton.MaterialRadioButton
android:id="@+id/radioFlash"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onRadioButtonClicked"
android:text="Flash" />
<com.google.android.material.radiobutton.MaterialRadioButton
android:id="@+id/radioFlex"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onRadioButtonClicked"
android:text="Flex" />
<com.google.android.material.radiobutton.MaterialRadioButton
android:id="@+id/radioColdFusion"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onRadioButtonClicked"
android:text="ColdFusion" />
<com.google.android.material.radiobutton.MaterialRadioButton
android:id="@+id/radioPhotoshop"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="onRadioButtonClicked"
android:text="Photoshop" />
</RadioGroup>
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
tools:text="TextView"
android:textColor="#483D8B"
style="@style/TextAppearance.MaterialComponents.Headline5"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/radioGroup" />
</androidx.constraintlayout.widget.ConstraintLayout>
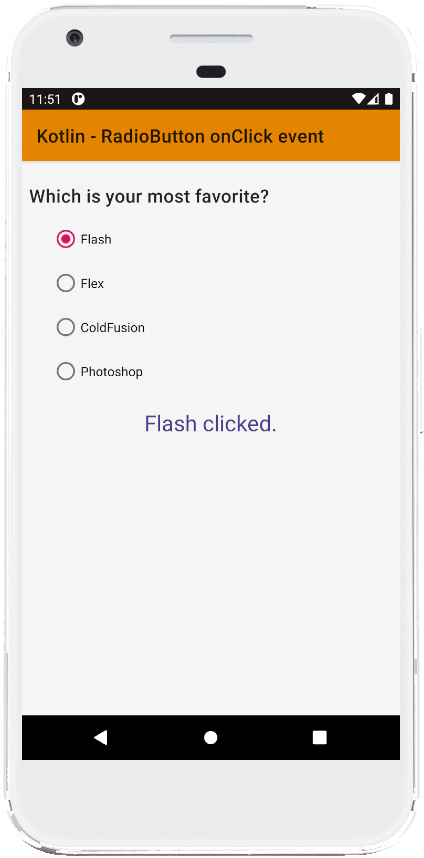

- android kotlin - RadioButton circle color programmatically
- android kotlin - Change checked RadioButton text color
- android kotlin - RadioGroup onCheckedChange listener
- android kotlin - Get RadioGroup selected item
- android kotlin - TextView add Hyperlink programmatically
- android kotlin - TextView get width height programmatically
- android kotlin - TextView html formatted text
- android kotlin - Coroutines with timeout or null
- android kotlin - Coroutines async with timeout
- android kotlin - Coroutines with timeout
- android kotlin - Coroutines download image from url
- android kotlin - Coroutines url to bitmap
- android kotlin - Coroutines async
- android kotlin - Canvas draw text inside circle
- android kotlin - Canvas draw text wrap