This code demonstrates how to programmatically add a hyperlink to a TextView text in an Android application.
The code first defines a string variable htmlString
that contains HTML code for the desired text with a hyperlink. The <a>
tag is used to define the hyperlink, with the href
attribute specifying the URL of the link.
Next, the code uses the HtmlCompat.fromHtml()
function to convert the HTML string into a Spanned
object. The Spanned
object is an interface that represents text that can have different styles applied to different parts. In this case, the Spanned
object will contain the text with the hyperlink styled appropriately.
The code then sets the movementMethod
property of the TextView to a LinkMovementMethod
object. The LinkMovementMethod
class is responsible for handling link clicks in TextView widgets. When the user clicks on a part of the text that is formatted as a hyperlink, the LinkMovementMethod
will handle the click and launch the web browser to open the linked URL.
Finally, the code sets the text of the TextView to the Spanned
object that was created from the HTML string. This displays the text with the hyperlink in the TextView.
In summary,
The code creates a TextView with a hyperlink. Clicking the hyperlink will launch the web browser and open the linked URL.
Here is a table summarizing the steps involved in adding a hyperlink to a TextView text programmatically:
Step | Description |
---|---|
1 | Define a string variable containing HTML code for the desired text with a hyperlink. |
2 | Use the HtmlCompat.fromHtml() function to convert the HTML string into a Spanned object. |
3 | Set the movementMethod property of the TextView to a LinkMovementMethod object. |
4 | Set the text of the TextView to the Spanned object that was created from the HTML string. |
package com.example.jetpack
import android.os.Bundle
import android.text.method.LinkMovementMethod
import androidx.appcompat.app.AppCompatActivity
import androidx.core.text.HtmlCompat
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// string inside html markup objects
val htmlString = "Hyperlink inside TextView.<br />" +
"<a href='https://developer.android.com/'>developer.android.com</a>"
// spanned is the interface for text that has
// markup objects attached to ranges of it
val spanned = HtmlCompat.fromHtml(
htmlString, // source
HtmlCompat.FROM_HTML_MODE_LEGACY // flags
)
// LinkMovementMethod traverses links in the text buffer and scrolls
// if necessary. Supports clicking on links with DPad Center or Enter.
textView.movementMethod = LinkMovementMethod.getInstance()
// finally, show text view text with html hyperlink
textView.text = spanned
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="25dp"
android:textSize="30sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
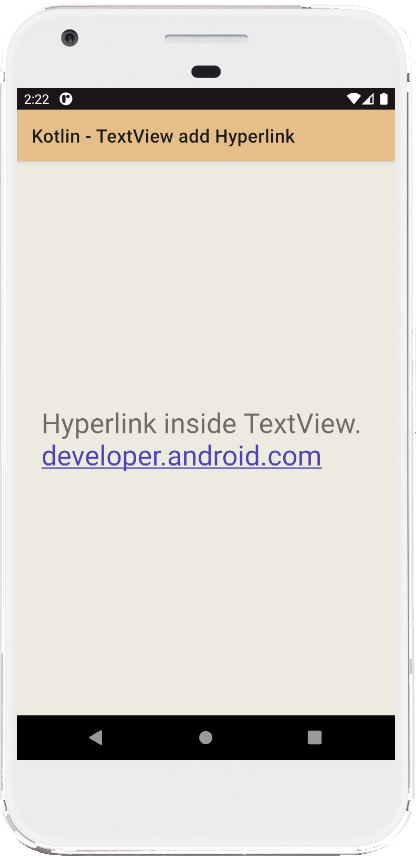

- android kotlin - Detect screen orientation change
- android kotlin - Convert dp to pixels programmatically
- android kotlin - Get screen size from context
- android kotlin - RecyclerView horizontal
- android kotlin - RecyclerView divider line
- android kotlin - GridView item height
- android kotlin - GridView add item dynamically
- android kotlin - CheckBox checked change listener
- android kotlin - Set CardView elevation
- android kotlin - RadioButton circle color programmatically
- android kotlin - Change checked RadioButton text color
- android kotlin - TextView margin programmatically
- android kotlin - Create TextView programmatically
- android kotlin - TextView get width height programmatically
- android kotlin - TextView html formatted text