Displaying HTML formatted text in an Android TextView (Kotlin)
This code snippet demonstrates how to display text with HTML formatting within a TextView element in an Android application written in Kotlin.
Explanation:
The code resides in the MainActivity.kt
file and defines an AppCompatActivity
subclass. The onCreate
method is responsible for initializing the activity's layout and functionalities when it's first created. Here's a breakdown of the key steps:
Define HTML String: A String variable named
htmlString
is created. It holds the text content you want to display, but wrapped in HTML tags to specify formatting. In this example, it includes bold, italic, underlined text, and line breaks using<br />
tags.Convert to Spanned: The
HtmlCompat.fromHtml
function is used to convert thehtmlString
into aSpanned
object.Spanned
is an interface in Android that represents text with embedded formatting information. ThefromHtml
function parses the HTML tags and creates aSpanned
object with the corresponding formatting applied. The code also uses theFROM_HTML_MODE_LEGACY
flag, which ensures compatibility with older Android versions.Set Text on TextView: Finally, the
Spanned
object containing the formatted text is assigned to thetext
property of thetextView
element. This displays the text with the desired HTML formatting within the TextView.
Summary:
By leveraging the HtmlCompat.fromHtml
function, this code effectively transforms a basic String with HTML markup into a Spanned
object understood by the TextView. This allows the TextView to render the text with the specified formatting (bold, italic, underline, line breaks) within the application's UI.
package com.example.jetpack
import android.os.Bundle
import android.text.Spanned
import androidx.appcompat.app.AppCompatActivity
import androidx.core.text.HtmlCompat
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// string inside html markup objects
val htmlString : String = "TextView first line... <br />" +
"<b>Bold Text</b> | <i>Italic Text</i> and <br/>" +
"<u>Underlined text</u>"
// spanned is the interface for text that has
// markup objects attached to ranges of it
val spanned : Spanned = HtmlCompat
// HtmlCompat is the backwards compatible version of Html
.fromHtml(
htmlString, // source
// flag that separate block-level elements with blank lines
// (two newline characters) in between
HtmlCompat.FROM_HTML_MODE_LEGACY // flags
)
// finally, show html formatted text in text view
textView.text = spanned
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="25dp"
tools:text="TextView"
android:textSize="30sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
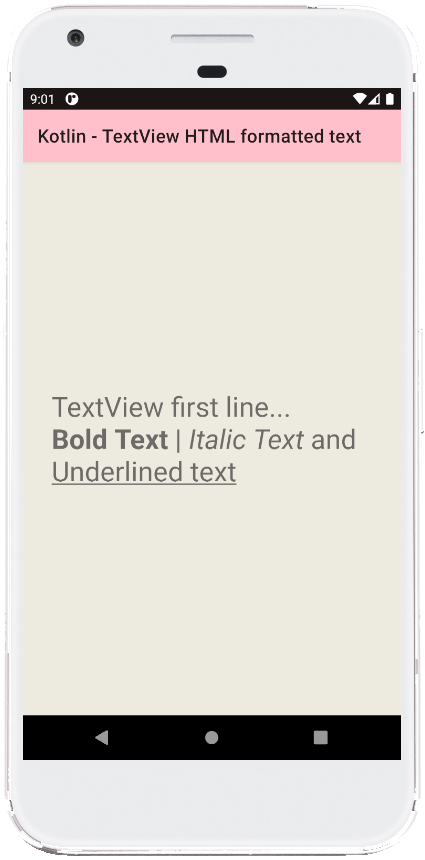
- android kotlin - Create ImageView programmatically
- android kotlin - Get battery temperature programmatically
- android kotlin - Get battery health programmatically
- android kotlin - Convert dp to pixels programmatically
- android kotlin - Get screen size from context
- android kotlin - GridView item height
- android kotlin - GridView add item dynamically
- android kotlin - CheckBox checked change listener
- android kotlin - Set CardView elevation
- android kotlin - RadioButton circle color programmatically
- android kotlin - Change checked RadioButton text color
- android kotlin - TextView margin programmatically
- android kotlin - Create TextView programmatically
- android kotlin - TextView add Hyperlink programmatically
- android kotlin - TextView get width height programmatically