Android Kotlin - Volley String Request: Breakdown
This code demonstrates using Volley, a popular Android network library, to fetch a string response from a URL using Kotlin. The code consists of three parts:
MainActivity.kt: This file defines the main activity of the app. It contains the user interface elements (button, progress bar, and text view) and handles user interaction with the button.
VolleySingleton.kt: This file implements a singleton class for managing the Volley request queue. This ensures there's only one instance of the request queue, improving efficiency.
activity_main.xml: This file defines the layout of the main activity screen, including the button, progress bar, and text view.
How it Works
UI Setup: When the activity starts (onCreate), the code retrieves references to the button, progress bar, and text view from the layout. It also enables scrolling for the text view to accommodate longer responses.
Fetch String on Button Click: Clicking the "Run Volley" button triggers the following actions:
- The button is disabled to prevent multiple clicks.
- The progress bar is shown to indicate ongoing network activity.
- A StringRequest object is created. This object defines the request type (GET in this case), URL to fetch, and two listener functions.
- The first listener (
{ response -> ... }
) handles a successful response. It hides the progress bar and displays the fetched string in the text view. - The second listener (
{error -> ... }
) handles any errors during the request. It hides the progress bar and displays an error message in the text view.
- The first listener (
- The StringRequest object is added to the request queue managed by the VolleySingleton class.
VolleySingleton: This class provides a single instance of the request queue, accessible throughout the application. It uses application context to avoid memory leaks and allows adding any type of Volley request (not just StringRequest) to the queue.
package com.cfsuman.kotlintutorials
import android.app.Activity
import android.os.Bundle
import android.text.method.ScrollingMovementMethod
import android.view.View
import android.widget.*
import com.android.volley.Request
import com.android.volley.toolbox.StringRequest
class MainActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the widgets reference from XML layout
val button = findViewById<Button>(R.id.button)
val progressBar = findViewById<ProgressBar>(R.id.progressBar)
val textView = findViewById<TextView>(R.id.textView)
// Make text view content scrollable
textView.movementMethod = ScrollingMovementMethod()
// url to fetch string
val url = "https://android--code.com/"
// fetch string from url using volley network library
button.setOnClickListener {
// disable the button itself
it.isEnabled = false
progressBar.visibility = View.VISIBLE
// request a string response from the provided url
val stringRequest = StringRequest(
Request.Method.GET, url,
{ response ->
progressBar.visibility = View.INVISIBLE
// display the response string on text view
textView.text = response
},{error->
progressBar.visibility = View.INVISIBLE
textView.text = error.message
}
)
VolleySingleton.getInstance(applicationContext)
.addToRequestQueue(stringRequest)
}
}
}
package com.cfsuman.kotlintutorials
import android.content.Context
import com.android.volley.Request
import com.android.volley.RequestQueue
import com.android.volley.toolbox.Volley
class VolleySingleton constructor(context: Context) {
companion object {
@Volatile
private var INSTANCE: VolleySingleton? = null
fun getInstance(context: Context) =
INSTANCE ?: synchronized(this) {
INSTANCE ?: VolleySingleton(context).also {
INSTANCE = it
}
}
}
private val requestQueue: RequestQueue by lazy {
// applicationContext is key, it keeps you from leaking the
// Activity or BroadcastReceiver if someone passes one in.
Volley.newRequestQueue(context.applicationContext)
}
fun <T> addToRequestQueue(req: Request<T>) {
requestQueue.add(req)
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#DCDCDC"
android:padding="24dp">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Run Volley"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="8dp"
android:visibility="invisible"
app:layout_constraintBottom_toBottomOf="@+id/button"
app:layout_constraintStart_toEndOf="@+id/button"
app:layout_constraintTop_toTopOf="@+id/button" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginTop="16dp"
android:fontFamily="sans-serif"
android:textSize="24sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
// Volley network library
implementation 'com.android.volley:volley:1.2.1'
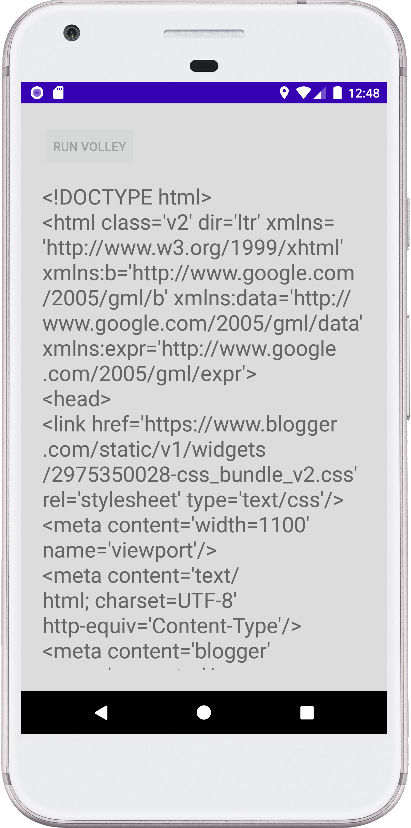
- android kotlin - Volley JsonArrayRequest
- android kotlin - Volley JsonObjectRequest
- android kotlin - Volley image request
- android kotlin - Chip center text
- android kotlin - Get screen size category
- android kotlin - Get API level programmatically
- android kotlin - Play default ringtone programmatically
- android kotlin - Enable disable bluetooth programmatically
- android kotlin - Change screen orientation programmatically
- android kotlin - Change orientation without restarting activity
- android kotlin - Get screen width and height in dp
- android kotlin - Get screen density programmatically
- android kotlin - Convert pixels to dp programmatically
- android kotlin - Canvas draw text
- android kotlin - Canvas draw circle