Introduction
When developing dynamic web applications with ColdFusion, you’ll often need to manipulate and transform strings. One common task is converting text to uppercase for uniformity or emphasis in display. ColdFusion provides a straightforward function called UCase()
that allows you to easily convert any string to its uppercase equivalent.
In this tutorial, we will explore a simple example of how to use the UCase()
function in ColdFusion to convert a string to uppercase. We'll also break down the code to understand how it works and why it’s useful in real-world scenarios.
Setting Up the Test String
The example code begins by defining a test string using the <cfset>
tag. This tag is used to assign values to variables in ColdFusion. In this case, a variable named TestString
is initialized with the value "My name is JONES"
. The mix of lowercase and uppercase letters in this string allows us to demonstrate how the UCase()
function transforms it into a fully uppercase version.
Displaying the Original String
After setting the test string, the code uses ColdFusion's <cfoutput>
tag to display dynamic content on the web page. Inside the <cfoutput>
block, the original TestString
is displayed using ColdFusion's variable output syntax: #TestString#
. This feature is important because it allows developers to embed dynamic data in their HTML output, such as variables or expressions, without the need for additional scripting languages.
Converting the String to Uppercase
Next, the code demonstrates the use of the UCase()
function. This built-in ColdFusion function takes a string as an argument and returns the uppercase version of that string. In the example, UCase(TestString)
is used to transform the original string into uppercase, which is then displayed using the same #variable#
output syntax.
Formatting the Output
The output of both the original string and the uppercase version is enclosed in HTML <b>
tags, which boldens the text for emphasis. Additionally, a <br />
tag is used to insert a line break, ensuring that the two versions of the string appear on separate lines. The use of basic HTML alongside ColdFusion code makes the output visually distinct and easier to read.
Conclusion
The UCase()
function in ColdFusion is a simple yet powerful tool for string manipulation, especially when you need to standardize text or format it for display purposes. By following this example, you’ve seen how to easily convert a string to uppercase and how to integrate this functionality within a ColdFusion page.
Whether you’re preparing data for display, processing form inputs, or generating reports, understanding how to manipulate strings in ColdFusion can make your development process more efficient and effective.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>UCase function example: how to convert a string to uppercase</title>
</head>
<body>
<h2 style="color:hotpink">UCase Function Example</h2>
<cfset TestString="My name is JONES">
<cfoutput>
<b>
TestString: #TestString#
<br />
TestString[uppercase]: #UCase(TestString)#
</b>
</cfoutput>
</body>
</html>
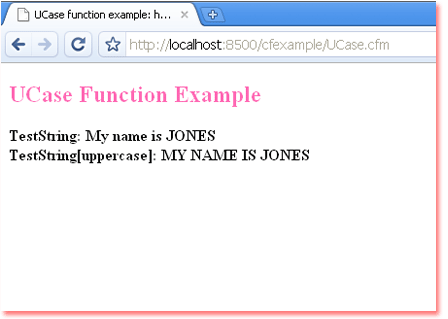
- LCase() - convert a string to lowercase
- Left() - get the leftmost count characters in a string
- Len() - get the length of a string
- LTrim() - remove leading spaces from a string
- Mid() - extracts a substring from a string
- RemoveChars() - remove characters from a string
- RepeatString()
- Replace() - replace a substring in a string (case sensitive)
- Trim() - remove leading and trailing spaces from a string