Introduction
When working with strings in Adobe ColdFusion, you often need to extract a portion of a string for various purposes, such as truncating or formatting text. One common operation is retrieving a specific number of characters from the beginning of a string. ColdFusion provides the Left()
function to achieve this with ease. In this tutorial, we'll explore how to use the Left()
function to extract the leftmost characters from a string. This simple yet powerful tool is incredibly useful in a variety of scenarios, including string manipulation, data formatting, and user interface design.
This example demonstrates how to use ColdFusion's Left()
function to obtain the first few characters of a string, guiding you step-by-step through a practical example in a web-based format.
Setting Up the Page Structure
The example code begins by creating a basic HTML structure with a simple DOCTYPE
declaration and an HTML document header. It includes meta information for the character set (UTF-8), ensuring that the content is encoded properly and displayed correctly in web browsers. The title of the webpage is descriptive: "Left function example: how to get the leftmost count characters in a string," clearly indicating the focus of the tutorial.
In the body of the HTML, we use an h2
tag to display the heading "Left Function Example" with a hot pink color, making the title of the example visually stand out on the page. This aesthetic enhancement highlights the demonstration of ColdFusion's Left()
function.
Defining and Displaying the Test String
The first key step in this example is the definition of the string we will manipulate. Using the ColdFusion tag <cfset>
, we define a variable called TestString
and assign it the value "This is a test string." This string serves as the data we will use to demonstrate how the Left()
function works.
Next, we use a ColdFusion output block, <cfoutput>
, to display both the original string and the result of the Left()
function. Inside this block, the original TestString
is displayed with a label, followed by the output of the Left()
function, which extracts the first 5 characters from TestString
. The output is wrapped in bold tags (<b>
) to make the result visually prominent on the page.
Using the Left() Function
The main focus of this example is the Left()
function, which is used to extract a specified number of characters from the beginning of a string. In this case, the function is called as Left(TestString, 5)
. This instructs ColdFusion to return the first 5 characters of the string stored in the TestString
variable, which is "This ".
The Left()
function is simple yet powerful, allowing you to extract any number of characters from the start of a string. The function takes two arguments: the string you want to manipulate (in this case, TestString
), and the number of characters you want to retrieve (5). This flexibility allows for a wide range of string manipulation tasks in ColdFusion applications.
Output Display
The output generated by this example is straightforward. It first displays the full content of TestString
, followed by the first five characters extracted using the Left()
function. The final output on the page would look something like this:
**TestString: This is a test string
TestString[Left 5 characters]: This **
This output makes it clear how the Left()
function works in practice, showing the difference between the original string and the truncated version returned by the function.
Conclusion
In this tutorial, we've demonstrated how to use the Left()
function in ColdFusion to retrieve the leftmost characters of a string. This function is highly useful in various string manipulation scenarios, whether you're truncating text, formatting output, or handling user input. The Left()
function is easy to implement and can be adapted to many use cases, making it a valuable tool in any ColdFusion developer's toolkit.
By following this simple example, you now have a clear understanding of how to extract the leftmost portion of a string in ColdFusion, which you can apply to more complex projects and real-world applications.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Left function example: how to get the leftmost count characters in a string</title>
</head>
<body>
<h2 style="color:HotPink">Left Function Example</h2>
<cfset TestString="This is a test string">
<cfoutput>
<b>
TestString: #TestString#
<br />
TestString[Left 5 characters]: #Left(TestString,5)#
</b>
</cfoutput>
</body>
</html>
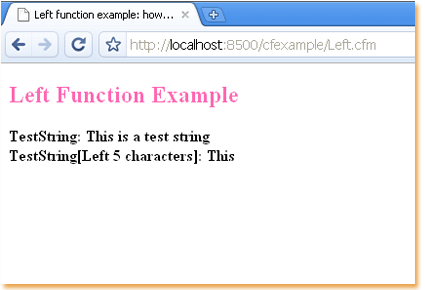
- Asc() - get the value of a character
- Compare() - compare two strings (case sensitive)
- CompareNoCase() - compare two strings (case insensitive)
- LCase() - convert a string to lowercase
- Len() - get the length of a string
- LTrim() - remove leading spaces from a string
- Mid() - extracts a substring from a string
- RemoveChars() - remove characters from a string
- RepeatString()
- Replace() - replace a substring in a string (case sensitive)