Introduction
Manipulating strings is an essential aspect of web development, and Adobe ColdFusion offers various built-in functions to simplify such tasks. One common need is removing specific characters from a string. This tutorial will guide you through the process of using the RemoveChars
function in ColdFusion, demonstrating how to efficiently remove portions of a string based on specific criteria such as the starting position and the number of characters to remove. The example code we’ll walk through is a simple yet powerful tool for learning how to implement this function in real-world scenarios.
Overview of the Example
The example code provided demonstrates how to use the RemoveChars
function in a ColdFusion file (CFM). The goal of this example is to show how easy it is to remove a subset of characters from a string based on its position and length. By utilizing this function, developers can manipulate string data dynamically, allowing for flexible and efficient processing of user input, database records, or other forms of text-based data.
HTML Structure
The code starts with a basic HTML structure that includes the standard DOCTYPE
declaration and HTML tags. This ensures the page is well-formed and compatible with modern web standards. The <meta>
tag defines the character set as UTF-8, ensuring the page can handle a wide range of characters, which is important for any text manipulation task.
The title of the page is set to "RemoveChars function example: how to remove characters from a string," indicating the focus of the demonstration. This helps users or developers quickly understand the purpose of the page. Inside the <body>
, there’s an <h2>
element with a heading styled in “HotPink,” which adds a touch of color to the demonstration, making it visually appealing.
Defining and Displaying the String
In the ColdFusion part of the code, a variable named TestString
is defined using the cfset
tag. This variable holds the text "Test string for RemoveChars function," which will be used in the example to demonstrate how to remove characters.
Next, the cfoutput
block is used to display content dynamically. Inside the cfoutput
, the value of TestString
is shown on the web page, enclosed in a <b>
tag to make it bold. This allows users to see the original string before any changes are applied. The usage of ColdFusion variables in the output is done with the #
signs, which is typical in ColdFusion syntax for outputting values.
Applying the RemoveChars Function
The real functionality comes in the next line of the cfoutput
block. The RemoveChars
function is used here to remove characters from the string, starting at the 6th character and removing 6 characters in total. The syntax RemoveChars(TestString,6,6)
tells ColdFusion to start at position 6 in the TestString
and remove the next 6 characters. This operation is then displayed right below the original string, allowing a clear comparison between the original and modified versions of the string.
In this case, the characters "string" are removed from the text, leaving the output as "Test for RemoveChars function." This demonstrates how the function can be used to modify a string by targeting a specific portion of it.
Conclusion
The RemoveChars
function in ColdFusion is a simple yet powerful tool for manipulating strings by removing specific characters based on their position and count. In this example, we walked through how to define a string, display it, and then apply the RemoveChars
function to modify that string dynamically. This function is particularly useful in scenarios where you need to clean up or format text by removing unwanted characters, such as when processing user input or preparing data for display.
By understanding how to utilize ColdFusion's built-in functions like RemoveChars
, you can enhance your web applications' flexibility and improve your ability to handle complex text processing tasks efficiently.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>RemoveChars function example: how to remove characters from a string</title>
</head>
<body>
<h2 style="color:HotPink">RemoveChars Function Example</h2>
<cfset TestString="Test string for RemoveChars function">
<cfoutput>
<b>
TestString: #TestString#
<br />
TestString[after remove substring start 6, count 6]: #RemoveChars(TestString,6,6)#
</b>
</cfoutput>
</body>
</html>
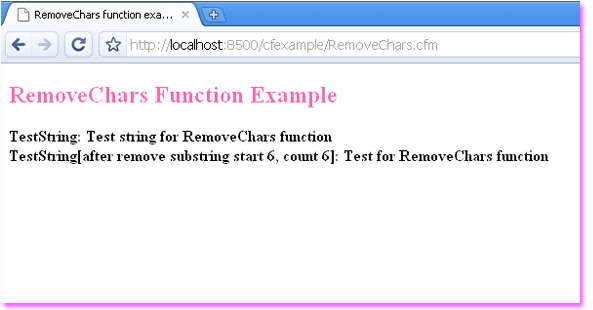
- LCase() - convert a string to lowercase
- Left() - get the leftmost count characters in a string
- Len() - get the length of a string
- Mid() - extracts a substring from a string
- RepeatString()
- Replace() - replace a substring in a string (case sensitive)
- ReplaceNoCase() - replace a substring in a string (case insensitive)
- Reverse() - reverse chracters in a string or digits in a number
- Right() - get the rightmost count characters in a string
- RTrim() - remove spaces from the end of a string