This code demonstrates how to integrate an Android View into a Jetpack Compose layout.
Here's a breakdown of the code:
Setting up the Activity:
- The
MainActivity
class extendsAppCompatActivity
and overrides theonCreate
method. - Inside
onCreate
, thesetContent
method is called to set the content of the activity using Jetpack Compose. TheGetScaffold
composable is passed as the content.
- The
Scaffold with TopAppBar:
- The
GetScaffold
composable creates a Scaffold layout, which is a common layout structure in Jetpack Compose that provides a standard app bar, content area, and optional floating action button. - The Scaffold has a
TopAppBar
defined with a title and a background color.
- The
Main Content:
- The
MainContent
composable defines the content that will be displayed inside the Scaffold. - It uses a
Column
layout to arrange its child composables vertically. - Inside the Column, there's an
AndroidView
composable.
- The
Integrating AndroidView:
- The
AndroidView
composable allows you to embed an Android View directly within your Jetpack Compose layout. - In this example, it's used to create a
TextView
with some styling and display the current value of a counter that's managed by the Jetpack Compose code.
- The
Counter and Button:
- A
var
calledcounter
is created usingremember
to store the state of the counter. - A
Button
is displayed with the text "Update Counter". Clicking the button increments the counter state. - The
AndroidView
's content is updated whenever the counter state changes using theupdate
lambda.
- A
Overall, this code snippet showcases how Jetpack Compose can be used to integrate existing Android Views with composables, allowing for more flexibility in building user interfaces.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.TextView
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.graphics.Color
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.graphics.toArgb
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.unit.dp
import androidx.compose.ui.viewinterop.AndroidView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
GetScaffold()
}
}
@Composable
fun GetScaffold(){
Scaffold(
topBar = {
TopAppBar(
title = { Text(
text = "Compose - Using AndroidView"
)},
backgroundColor = Color(0xFFC0E8D5),
)
},
content = {MainContent()},
backgroundColor = Color(0xFFEDEAE0),
)
}
@Composable
fun MainContent(){
var counter by remember { mutableStateOf(0)}
Column(
modifier = Modifier
.fillMaxSize()
.padding(12.dp),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
){
AndroidView(factory = { context ->
TextView(context).apply {
setTextColor(Color.Blue.toArgb())
textSize = 24F
}
},
update = {
it.text = "State from composable ($counter)"
},
modifier = Modifier
.padding(12.dp)
.clip(RoundedCornerShape(12.dp))
.background(Color(0xFFF0FFFF))
.padding(16.dp)
)
Button(onClick = {
counter++
}) {
Text(text = "Update Counter")
}
}
}
}
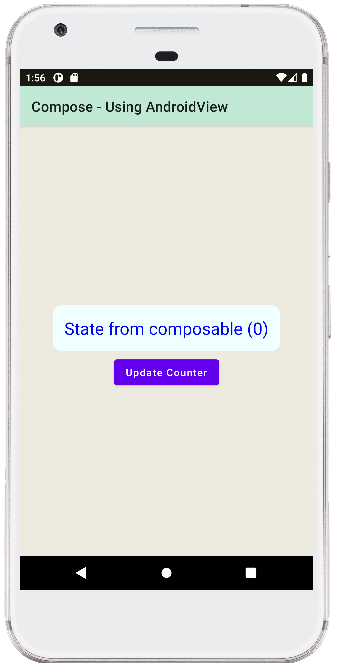
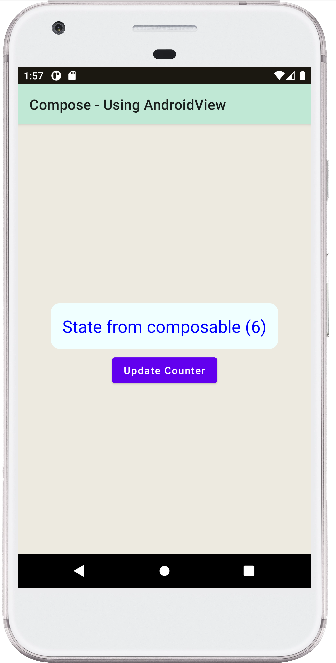
- jetpack compose - TopAppBar center title
- jetpack compose - TopAppBar menu
- jetpack compose - Snackbar action
- jetpack compose - Snackbar dismiss listener
- jetpack compose - How to update AndroidView
- jetpack compose - AndroidView click event
- jetpack compose - AndroidView modifier
- jetpack compose - How to use bottom navigation
- jetpack compose flow - Room add remove update
- jetpack compose flow - Room implement search
- jetpack compose - ViewModel Room edit update data
- jetpack compose - ViewModel Room delete clear data
- compose glance - How to create app widget
- jetpack compose - Icon from vector resource