This code snippet showcases the Jetpack Compose way of updating an existing Android View.
Here's a breakdown of the code:
The
setContent
method sets the content of the Activity using a Composable function. In this example, theGetScaffold
composable is set as the content.The
GetScaffold
composable function creates a Scaffold layout, which is a common layout structure in Jetpack Compose. It includes a TopAppBar, content area, and a background color.The
TopAppBar
composable function creates the app bar section at the top of the screen. It displays the title 'Compose - Update AndroidView' and sets the background color to 0xFFC0E8D5 (a light green shade).The
MainContent
composable function defines the content area of the Scaffold. It uses aColumn
layout to arrange its child composables vertically in the center of the screen.An
AndroidView
composable is used to integrate an existingTextView
from the Android View system. Theupdate
block is used to modify the text and text size of theTextView
dynamically. Whenever the button is clicked, the text size is incremented by 5, and theTextView
is updated accordingly.
In summary, this code demonstrates how to integrate and update an existing Android View within a Jetpack Compose layout. The AndroidView
composable provides a bridge between Compose and the traditional Android View system, allowing for the use of existing View components within Compose applications.
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.TextView
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.graphics.Color
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.graphics.toArgb
import androidx.compose.ui.unit.dp
import androidx.compose.ui.viewinterop.AndroidView
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
GetScaffold()
}
}
@Composable
fun GetScaffold(){
Scaffold(
topBar = {
TopAppBar(
title = { Text(
text = "Compose - Update AndroidView"
)},
backgroundColor = Color(0xFFC0E8D5),
)
},
content = {MainContent()},
backgroundColor = Color(0xFFEDEAE0),
)
}
@Composable
fun MainContent(){
var txtSize by remember { mutableStateOf(20F)}
Column(
modifier = Modifier
.fillMaxSize()
.padding(12.dp),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
){
AndroidView(factory = { context ->
TextView(context).apply {
setTextColor(Color(0xFF333399).toArgb())
}
},
update = {
it.text = "Update AndroidView (TextSize : $txtSize)"
it.textSize = txtSize
},
modifier = Modifier
.padding(12.dp)
.clip(RoundedCornerShape(12.dp))
.background(Color(0xFFF0FFFF))
.padding(16.dp)
)
Button(onClick = {
txtSize += 5
}) {
Text(text = "Update Android View")
}
}
}
}
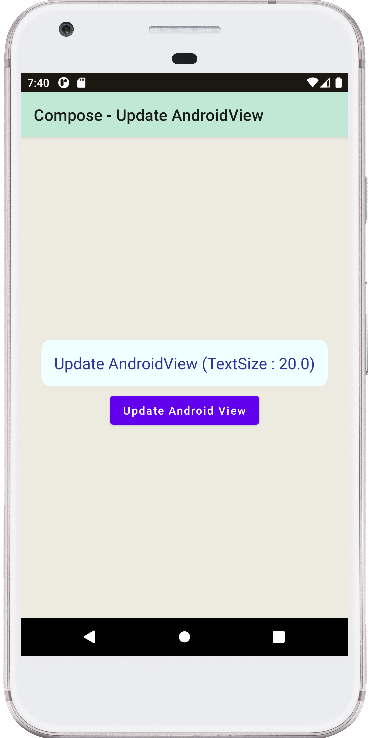
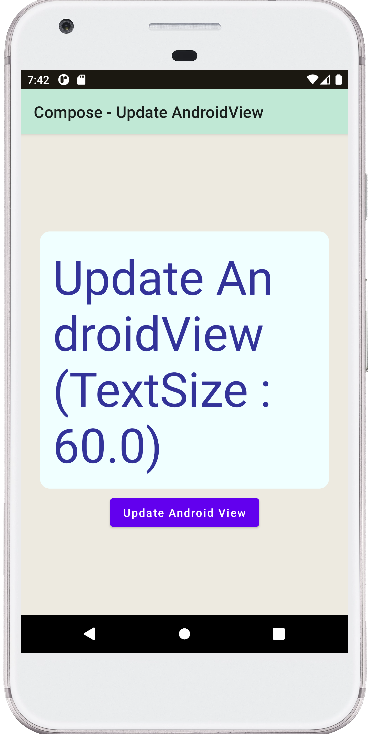
- jetpack compose - Box gravity
- jetpack compose - Card padding
- jetpack compose - Card background color
- jetpack compose - How to use ModalDrawer
- jetpack compose - How to use BadgeBox
- jetpack compose - TopAppBar center title
- jetpack compose - TopAppBar menu
- jetpack compose - Snackbar action
- jetpack compose - Snackbar dismiss listener
- jetpack compose - How to use AndroidView
- jetpack compose - AndroidView click event
- jetpack compose - AndroidView modifier
- jetpack compose - How to use bottom navigation
- jetpack compose - Icon from vector resource