Flutter - TextButton Background Color
The TextButton class represents a material design text button. The flutter
developers can use text buttons on toolbars, in dialogs, or in line with other
content. Text buttons do not have visible borders. The flutter developers
should avoid using text buttons where they would blend in with other content
such as in the middle of lists. The TextButton style can be overridden with
its style parameter.
The following flutter application development tutorial will demonstrate how we can set a background color for the TextButton. We will change the TextButton widget’s default background color. Here we will use the TextButton class’s styleFrom() method to change the TextButton’s default background color.
The TextButton class’s styleFrom() method is a static convenience method that constructs a text button ButtonStyle given simple values. By default, TextButton class styleFrom() method returns a ButtonStyle that doesn't override anything.
The ButtonStyle class represents the visual properties that most buttons have in common. The ButtonStyle class’s all properties are null by default.
The flutter developers can change a TextButton’s background color by passing a value to the styleFrom() method’s backgroundColor parameter.
So finally, the flutter app developers can set a background color for the TextButton widget by passing a value to the TextButton class’s styleFrom() method backgroundColor argument.
The following flutter application development tutorial will demonstrate how we can set a background color for the TextButton. We will change the TextButton widget’s default background color. Here we will use the TextButton class’s styleFrom() method to change the TextButton’s default background color.
The TextButton class’s styleFrom() method is a static convenience method that constructs a text button ButtonStyle given simple values. By default, TextButton class styleFrom() method returns a ButtonStyle that doesn't override anything.
The ButtonStyle class represents the visual properties that most buttons have in common. The ButtonStyle class’s all properties are null by default.
The flutter developers can change a TextButton’s background color by passing a value to the styleFrom() method’s backgroundColor parameter.
So finally, the flutter app developers can set a background color for the TextButton widget by passing a value to the TextButton class’s styleFrom() method backgroundColor argument.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const FlutterExample());
class FlutterExample extends StatelessWidget {
const FlutterExample({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Example',
theme: ThemeData(primarySwatch: Colors.red),
home: const StateExample()
);
}
}
class StateExample extends StatefulWidget {
const StateExample({Key? key}) : super(key: key);
@override
_StateExampleState createState() => _StateExampleState();
}
class _StateExampleState extends State<StateExample>{
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: const Color(0xFFFEFEFA),
appBar: AppBar(
title: const Text("Flutter - TextButton Background Color")
),
body: bodyContent(),
);
}
bodyContent() {
return Center(
child: TextButton(
child: const Text("Click Me"),
style: TextButton.styleFrom(
padding: const EdgeInsets.all(20),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(12))
),
backgroundColor: const Color(0xFFE3DAC9)
),
onPressed: (){
// do something here
},
)
);
}
}
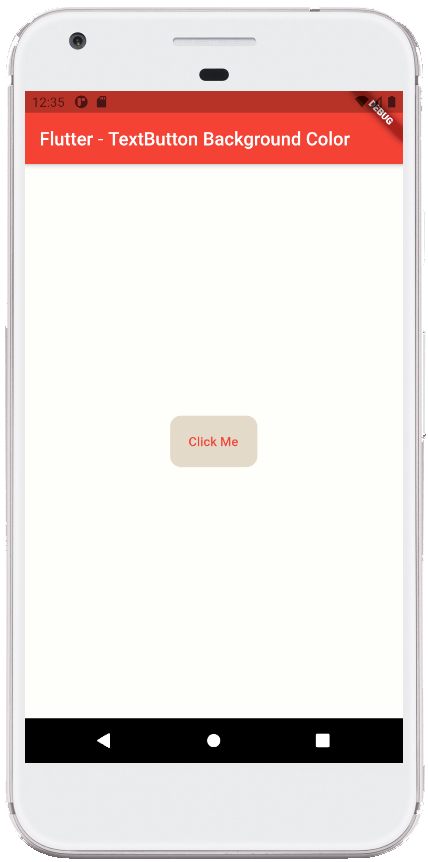