Flutter - OutlinedButton Icon
The OutlinedButton class represents a material design outlined button that is
essentially a TextButton with an outlined border. The outlined buttons are
medium-emphasis buttons. In a flutter application, the OutlinedButtons contain
actions that are important, but they are not the primary action in an app. An
OutlinedButton widget’s default style is defined by defaultStyleOf. The
flutter application developers can override the OutlinedButton’s style with
its style parameter. An OutlinedButton has a default ButtonStyle.side which
defines the appearance of the outline. The flutter app developers can specify
an OutlinedButton's shape and the appearance of its outline by specifying both
the ButtonStyle.shape and ButtonStyle.side properties.
The following flutter application development tutorial will demonstrate how to create an OutlinedButton widget with an icon. Here we will use the OutlinedButton.icon() constructor to create an OutlinedButton with an icon and text.
The OutlinedButton.icon({Key? key, required VoidCallback? onPressed, VoidCallback? onLongPress, ButtonStyle? style, FocusNode? focusNode, bool? autofocus, Clip? clipBehavior, MaterialStatesController? statesController, required Widget icon, required Widget label}) constructor creates a text button from a pair of widgets that serve as the button's icon and label.
The icon and label are arranged in a row inside the OutlinedButton widget. The icon and label arguments must not be null. So the flutter developers must have to pass the icon and label parameters value to this specified OutlinedButton constructor.
The icon parameter value is an Icon widget. The Icon class represents a graphical icon widget drawn with a glyph from a font described in an IconData such as material's predefined IconDatas in Icons.
So finally, the flutter application developers can create an OutlinedButton with an icon and text by using the OutlinedButton’s specified constructor. They have to pass the icon widget to this constructor to display it on the OutlinedButton. And also have to pass a value for the label parameter to display the text on OutlinedButton.
The following flutter application development tutorial will demonstrate how to create an OutlinedButton widget with an icon. Here we will use the OutlinedButton.icon() constructor to create an OutlinedButton with an icon and text.
The OutlinedButton.icon({Key? key, required VoidCallback? onPressed, VoidCallback? onLongPress, ButtonStyle? style, FocusNode? focusNode, bool? autofocus, Clip? clipBehavior, MaterialStatesController? statesController, required Widget icon, required Widget label}) constructor creates a text button from a pair of widgets that serve as the button's icon and label.
The icon and label are arranged in a row inside the OutlinedButton widget. The icon and label arguments must not be null. So the flutter developers must have to pass the icon and label parameters value to this specified OutlinedButton constructor.
The icon parameter value is an Icon widget. The Icon class represents a graphical icon widget drawn with a glyph from a font described in an IconData such as material's predefined IconDatas in Icons.
So finally, the flutter application developers can create an OutlinedButton with an icon and text by using the OutlinedButton’s specified constructor. They have to pass the icon widget to this constructor to display it on the OutlinedButton. And also have to pass a value for the label parameter to display the text on OutlinedButton.
main.dart
import 'package:flutter/material.dart';
void main() => runApp(const FlutterExample());
class FlutterExample extends StatelessWidget {
const FlutterExample({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Example',
theme: ThemeData(primarySwatch: Colors.green),
home: const StateExample()
);
}
}
class StateExample extends StatefulWidget {
const StateExample({Key? key}) : super(key: key);
@override
_StateExampleState createState() => _StateExampleState();
}
class _StateExampleState extends State<StateExample>{
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: const Color(0xFFFEFEFA),
appBar: AppBar(
title: const Text("Flutter - OutlinedButton Icon")
),
body: bodyContent(),
);
}
bodyContent() {
return Center(
child: OutlinedButton.icon(
icon: const Icon(
Icons.favorite,
color: Colors.pink,
),
label: const Text("Like Us"),
style: OutlinedButton.styleFrom(
padding: const EdgeInsets.all(20),
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(8))
)
),
onPressed: (){
// do something here
},
)
);
}
}
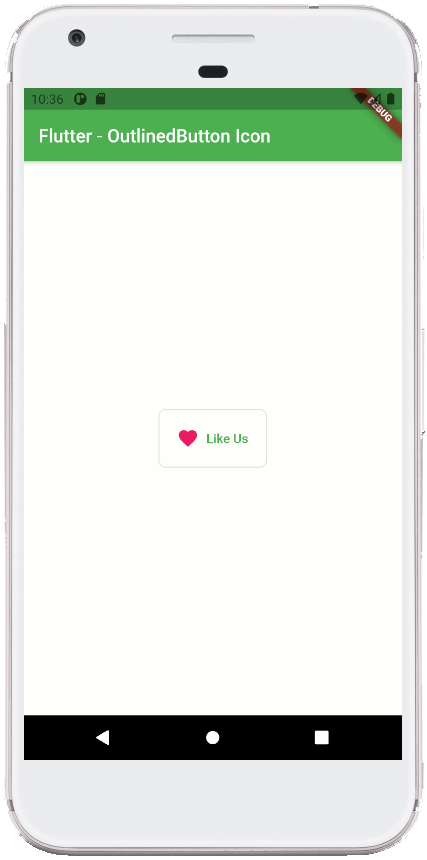