MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.background
import androidx.compose.foundation.gestures.rememberTransformableState
import androidx.compose.foundation.gestures.transformable
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.runtime.*
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.geometry.Offset
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.graphicsLayer
import androidx.compose.ui.layout.ContentScale
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
var scale by remember { mutableStateOf(1f) }
var rotation by remember { mutableStateOf(0f) }
var offset by remember { mutableStateOf(Offset.Zero) }
val state = rememberTransformableState {
zoomChange, offsetChange, rotationChange ->
scale *= zoomChange
rotation += rotationChange
offset += offsetChange
}
Column(
Modifier
.background(Color(0xFFEDEAE0))
.fillMaxSize()
.padding(16.dp)
) {
Image(
painter = painterResource(id = R.drawable.flower9),
contentDescription = "Localized description",
contentScale = ContentScale.Crop,
modifier = Modifier
.background(Color.Transparent)
.fillMaxSize()
.graphicsLayer(
scaleX = scale,
scaleY = scale,
rotationZ = rotation,
translationX = offset.x,
translationY = offset.y
)
.transformable(state = state)
.clip(RoundedCornerShape(16.dp)),
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
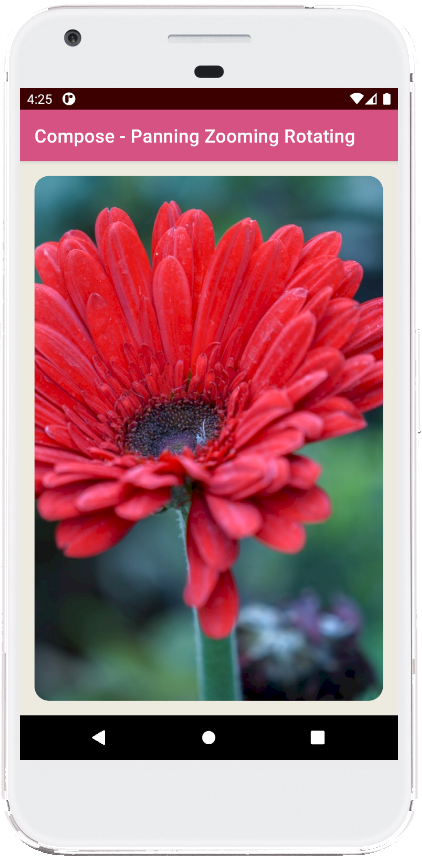
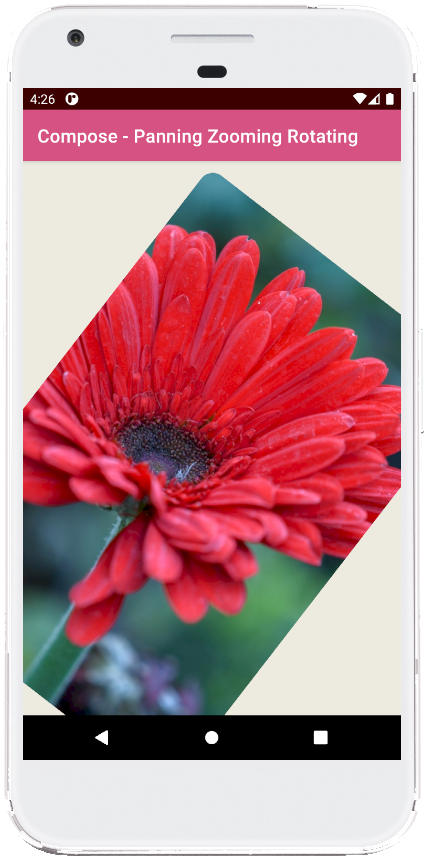
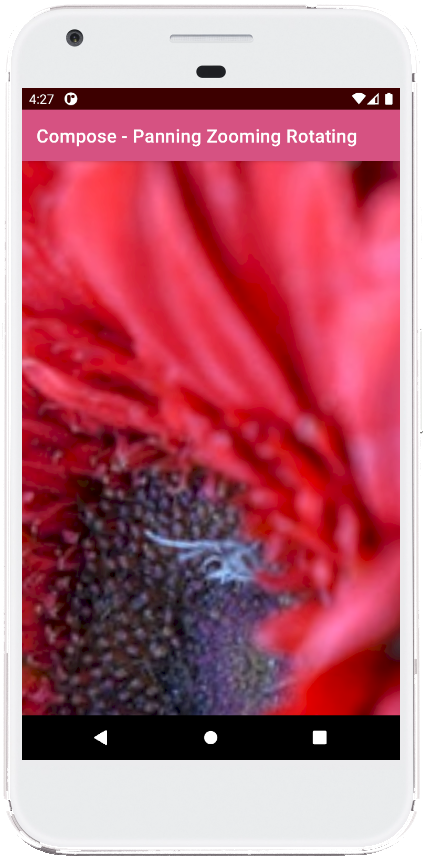
- jetpack compose - Navigation drawer example
- jetpack compose - Cut corner shape example
- jetpack compose - Image from drawable
- jetpack compose - Image clickable
- jetpack compose - Image border
- jetpack compose - Image shape
- jetpack compose - Image tint
- jetpack compose - Image from assets folder
- jetpack compose - How to use LazyColumn
- jetpack compose - Accessing string resources
- jetpack compose - String resource positional formatting
- jetpack compose - Dragging
- jetpack compose - Multiple draggable objects
- jetpack compose - Swiping
- jetpack compose - Weight modifier