Introduction
This code demonstrates how to implement navigation within a Jetpack Compose application using the Navigation Compose library. It showcases a simple app with three color-coded screens ("Red", "Green", and "Blue") that users can navigate between.
Breakdown
The code is structured within the MainActivity.kt
file. Here's a breakdown of the key components:
NavController: This object, created using
rememberNavController()
, is the central point for navigation control. It keeps track of the back stack (history of visited screens) and facilitates navigation actions.Scaffold: This composable provides a pre-built layout structure with a TopAppBar and content area. It's used here to organize the app's overall UI.
MainContent & MainNavigation: These composables handle the central content area.
MainContent
uses aBox
with centered alignment to position the navigation component,MainNavigation
.NavHost: This composable is the core for navigation. It takes the
navController
and defines the starting destination ("redScreen") and available composables ("redScreen", "greenScreen", and "blueScreen").Color-Coded Screens: Each screen (
RedScreen
,GreenScreen
, andBlueScreen
) uses aColumn
layout with centered content. They display a colored background and a title text. Buttons within each screen utilize thenavController.navigate
function to navigate to other screens.
Summary
The provided code offers a basic example of navigation in Jetpack Compose. It demonstrates how to set up navigation using rememberNavController
and NavHost
. The code also showcases how to define composables as destinations and navigate between them using buttons and the navController.navigate
function. This is a foundational concept for building multi-screen Compose applications.
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.graphics.Color
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import androidx.navigation.NavController
import androidx.navigation.NavHostController
import androidx.navigation.compose.NavHost
import androidx.navigation.compose.composable
import androidx.navigation.compose.rememberNavController
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
GetScaffold()
}
}
@Composable
fun GetScaffold(){
val navController:NavHostController = rememberNavController()
Scaffold(
topBar = {
TopAppBar(
title = { Text(
text = "Compose - Navigation Controller"
)},
backgroundColor = Color(0xFFC0E8D5),
)
},
content = {MainContent(navController)},
backgroundColor = Color(0xFFEDEAE0),
)
}
@Composable
fun MainContent(navController: NavHostController){
Box(
modifier = Modifier.fillMaxSize(),
contentAlignment = Alignment.Center,
){
MainNavigation(navController)
}
}
@Composable
fun MainNavigation(navController: NavHostController){
NavHost(
navController = navController,
startDestination = "redScreen"
){
composable("redScreen"){RedScreen(navController)}
composable("greenScreen"){GreenScreen(navController)}
composable("blueScreen"){BlueScreen(navController)}
}
}
@Composable
fun RedScreen(navController: NavController){
Column(
modifier = Modifier
.fillMaxSize()
.background(Color(0xFFE25822)),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "Red Screen",
style = MaterialTheme.typography.h5
)
Spacer(modifier = Modifier.height(24.dp))
Row(
horizontalArrangement = Arrangement.spacedBy(12.dp)
) {
Button(onClick = {
navController.navigate("greenScreen")
}) {
Text(text = "Go Green")
}
Button(onClick = {
navController.navigate("blueScreen")
}) {
Text(text = "Go Blue")
}
}
}
}
@Composable
fun GreenScreen(navController: NavController){
Column(
modifier = Modifier
.fillMaxSize()
.background(Color(0xFF7BB661)),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "Green Screen",
style = MaterialTheme.typography.h5
)
Spacer(modifier = Modifier.height(24.dp))
Row(
horizontalArrangement = Arrangement.spacedBy(12.dp)
) {
Button(onClick = {
navController.navigate("redScreen")
}) {
Text(text = "Go Red")
}
Button(onClick = {
navController.navigate("blueScreen")
}) {
Text(text = "Go Blue")
}
}
}
}
@Composable
fun BlueScreen(navController: NavController){
Column(
modifier = Modifier
.fillMaxSize()
.background(Color(0xFFA7D8DE)),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "Blue Screen",
style = MaterialTheme.typography.h5
)
Spacer(modifier = Modifier.height(24.dp))
Row(
horizontalArrangement = Arrangement.spacedBy(12.dp)
) {
Button(onClick = {
navController.navigate("redScreen")
}) {
Text(text = "Go Red")
}
Button(onClick = {
navController.navigate("greenScreen")
}) {
Text(text = "Go Green")
}
}
}
}
}
// compose navigation component
implementation 'androidx.navigation:navigation-compose:2.4.0-alpha10'
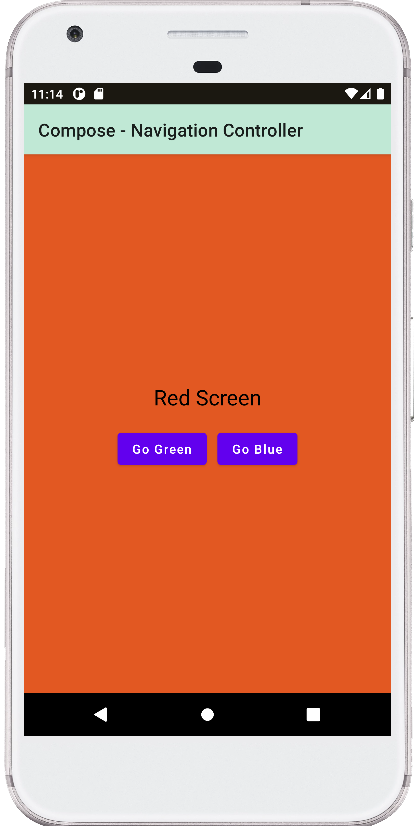
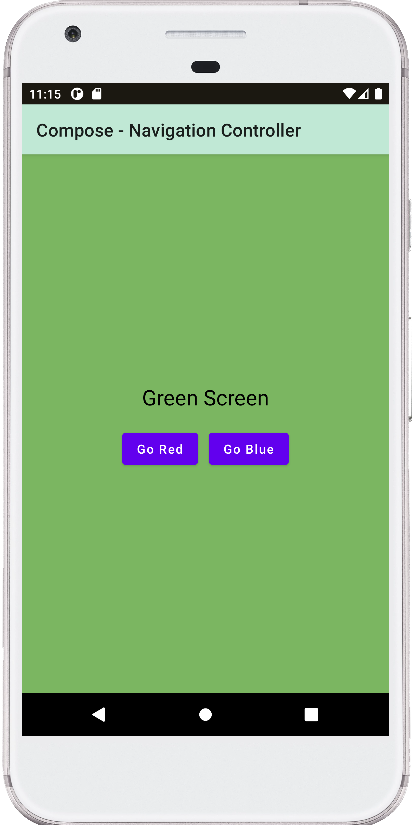
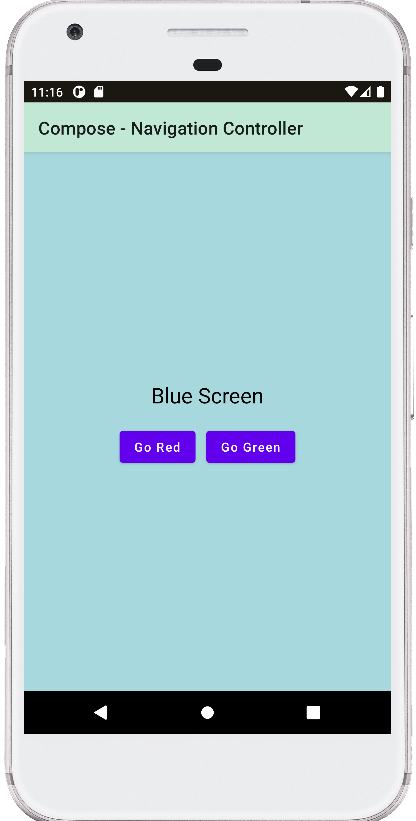
- jetpack compose - Navigate with argument
- jetpack compose - Navigation multiple arguments
- jetpack compose - Navigation arguments data type
- jetpack compose - Navigation object argument
- jetpack compose - WebView ProgressIndicator
- jetpack compose - WebView progress percentage
- jetpack compose - Backdrop scaffold
- jetpack compose - Double click listener
- jetpack compose - Long click listener
- jetpack compose - Pass onClick event to function
- jetpack compose - TabRow custon indicator
- jetpack compose - Get primary language
- jetpack compose - Get screen orientation
- jetpack compose - Icon from vector resource