MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.foundation.layout.padding
import androidx.compose.material.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.text.font.FontStyle
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.text.style.TextDecoration
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
Text(
text = "Text In Compose.",
color = Color.Red,
textDecoration = TextDecoration.Underline,
textAlign = TextAlign.Center,
fontSize = 35.sp,
fontStyle = FontStyle.Italic,
fontWeight = FontWeight.Bold,
fontFamily = FontFamily.Monospace,
modifier = Modifier
.background(Color.Yellow)
.padding(15.dp)
.fillMaxWidth()
)
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
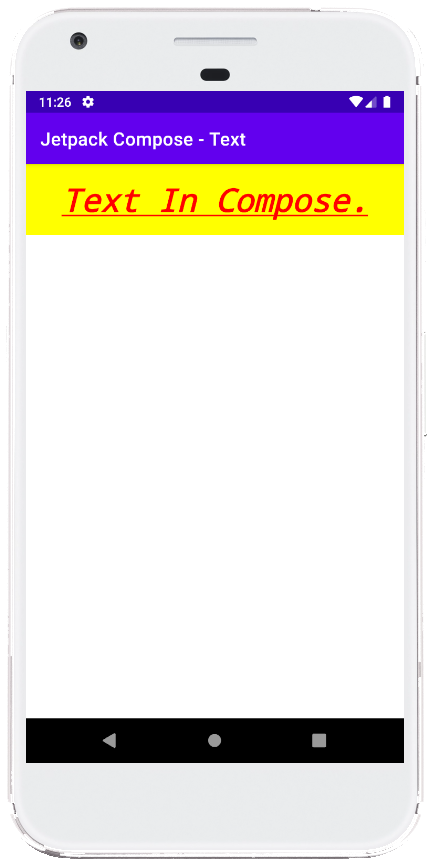
- jetpack compose - ClickableText example
- jetpack compose - How to use Button
- jetpack compose - How to use TextField
- jetpack compose - OutlinedTextField example
- jetpack compose - Password TextField example
- jetpack compose - Handle changes in a TextField
- jetpack compose - How to use Card
- jetpack compose - How to use Checkbox
- jetpack compose - How to use RadioButton
- jetpack compose - Radio group example
- jetpack compose - How to use Floating Action Button
- jetpack compose - Extended floating action button example
- jetpack compose - How to use DropdownMenu
- jetpack compose - How to use IconButton
- jetpack compose - How to use IconToggleButton