Android Kotlin: Converting Drawable to Bitmap
This code demonstrates how to convert a drawable resource referenced in the Android project to a Bitmap object in Kotlin. Bitmaps are a fundamental data structure for representing images in Android. They allow for pixel manipulation and various image processing tasks.
The code is implemented within the MainActivity.kt
file, which is the main activity class for this example.
Code Breakdown
- Import Statements: Necessary classes for working with Bitmaps, Drawables, and resources are imported.
- Activity Class Definition: The
MainActivity
class inherits fromAppCompatActivity
. - onCreate Method: This method is called when the activity is first created.
- The layout (
activity_main.xml
) is inflated usingsetContentView
. - Drawable to Bitmap Conversion:
ResourcesCompat.getDrawable(resources, R.drawable.flower, null)
retrieves the drawable resource referenced byR.drawable.flower
from the application resources.- The retrieved drawable is cast to a
BitmapDrawable
as this type of drawable holds a Bitmap internally. - Finally, the
bitmap
property of theBitmapDrawable
is accessed to obtain the actual Bitmap object.
- Setting Image Source: The
setImageBitmap
method of theimageView
is called, passing the convertedbitmap
as its argument. This sets the image displayed by theImageView
to the converted drawable.
- The layout (
Summary
By converting the drawable resource to a Bitmap, the code gains the ability to manipulate the image data or perform further processing if needed. This approach is useful when you require more control over the image data compared to simply referencing a drawable resource directly in the layout.
MainActivity.kt
package com.example.jetpack
import android.graphics.Bitmap
import android.graphics.drawable.BitmapDrawable
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.core.content.res.ResourcesCompat
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// convert drawable to bitmap
val bitmap:Bitmap = (ResourcesCompat.getDrawable(
resources,R.drawable.flower,null)
as BitmapDrawable).bitmap
// set image view image source bitmap
imageView.setImageBitmap(bitmap)
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@tools:sample/avatars" />
</androidx.constraintlayout.widget.ConstraintLayout>
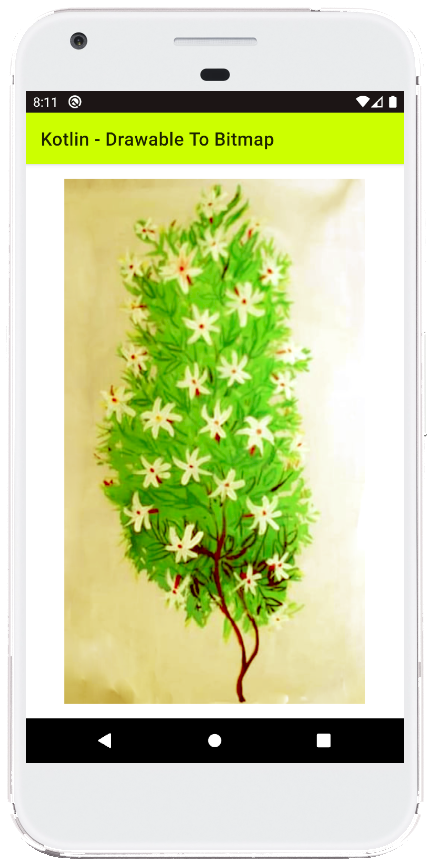