The code defines an activity named MainActivity
that displays an image in an ImageView
. The image is loaded from the drawable resources of the application.
Here's a step-by-step breakdown of the code:
Import statements:
- The code starts by importing necessary libraries.
androidx.appcompat.app.AppCompatActivity
is imported to create an activity that extends the functionality of the standardActivity
class.androidx.core.content.ContextCompat
provides methods to access resources from the context of an activity.kotlinx.android.synthetic.main.activity_main
is a synthetic import that allows you to access views from the layout fileactivity_main.xml
directly in your code.
Class definition:
- The code defines a class named
MainActivity
that inherits fromAppCompatActivity
. This class represents the main activity of the application.
- The code defines a class named
onCreate method:
- The
onCreate
method is the entry point of the activity. It's called when the activity is first created. - Inside the
onCreate
method:- The
setContentView(R.layout.activity_main)
method sets the layout of the activity to the one defined in theactivity_main.xml
file. - The code gets a reference to the
ImageView
from the layout usingkotlinx.android.synthetic.main.activity_main.imageView
. - The
ContextCompat.getDrawable(context, R.drawable.flower)
method retrieves a drawable resource from the application's resources identified by the nameflower
. - The retrieved drawable is then set to the
ImageView
using thesetImageDrawable
method.
- The
- The
In summary, this code snippet demonstrates how to load an image from the drawable resources and display it in an ImageView in an Android app written in Kotlin.
MainActivity.kt
package com.example.jetpack
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import androidx.core.content.ContextCompat
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val context = this
// set image view image from drawable
imageView.setImageDrawable(ContextCompat.getDrawable(
context,R.drawable.flower
))
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#636C5A"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="16dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="16dp"
android:layout_marginBottom="16dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:srcCompat="@tools:sample/avatars" />
</androidx.constraintlayout.widget.ConstraintLayout>
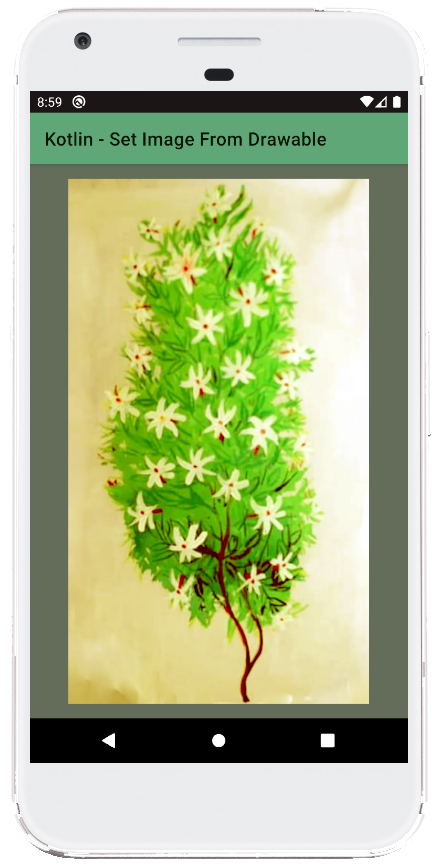