MainActivity.kt
package com.cfsuman.kotlintutorials
import android.app.Activity
import android.os.Bundle
import android.widget.NumberPicker
import android.widget.TextView
class MainActivity : Activity() {
private lateinit var numberPicker:NumberPicker
private lateinit var textView:TextView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the widgets reference from XML layout
numberPicker = findViewById(R.id.numberPicker)
textView = findViewById(R.id.textView)
// display string values to the number picker
// at first, initialize a new array instance with string values
val colors = arrayOf("Royal purple", "Sapphire", "Sandy brown",
"Black olive", "Android green", "Pink lace", "Barbie Pink")
// set the number picker minimum and maximum values
numberPicker.minValue = 0
numberPicker.maxValue = colors.size - 1
// set the valued to be displayed in number picker
numberPicker.displayedValues = colors
// get number picker selected value
textView.text = "Selected Color : ${colors[numberPicker.value]}"
// number picker value change listener
numberPicker.setOnValueChangedListener { picker, oldVal, newVal ->
// display the picker current selected value to text view
textView.text = "Selected Color : ${colors[newVal]}"
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#DCDCDC"
android:padding="32dp">
<NumberPicker
android:id="@+id/numberPicker"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:descendantFocusability="blocksDescendants"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginTop="12dp"
android:fontFamily="sans-serif-condensed-medium"
android:gravity="center"
android:padding="8dp"
android:textColor="#4F42B5"
android:textSize="22sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/numberPicker"
tools:text="TextView" />
</androidx.constraintlayout.widget.ConstraintLayout>
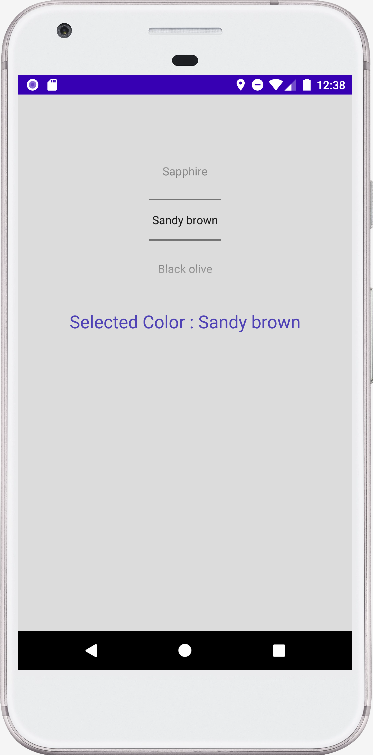
- android kotlin - ChipGroup single selection
- android kotlin - NumberPicker divider height
- android kotlin - NumberPicker remove divider
- android kotlin - NumberPicker divider color
- android kotlin - EditText set max length programmatically
- android kotlin - EditText rounded corners programmatically
- android kotlin - EditText border color programmatically
- android kotlin - EditText enter key listener
- android kotlin - EditText listener
- android kotlin - EditText TextWatcher
- android kotlin - ImageView border radius
- android kotlin - ImageView add border programmatically
- android kotlin - ImageView add border
- android kotlin - On back button pressed example
- android kotlin - Get string resource by name