This code demonstrates how to resize an ImageView programmatically in an Android application written in Kotlin. It allows the user to click a button and randomly resize the image displayed in the ImageView.
The code includes three parts:
- MainActivity.kt: This file contains the main logic for the activity. It loads an image from the assets folder, displays it on the ImageView, and implements a button click listener to resize the ImageView with random dimensions.
- Extension functions: The code defines three extension functions:
resize
: This function allows resizing the ImageView by setting new width and height values for its LayoutParams.assetsToBitmap
: This function retrieves a Bitmap image from the app's assets folder.dpToPixels
: This function converts a value in dp (density-independent pixels) to its equivalent pixel size based on the device's screen density.
- activity_main.xml: This file defines the layout for the activity, including the ImageView, a button to trigger resizing, and a TextView to display the new dimensions after resizing.
Summary
This code provides a simple example of programmatically resizing an ImageView in an Android app. It demonstrates loading an image, attaching a click listener to a button, and using extension functions for common operations like resizing and unit conversion. With a few clicks, the user can see the image displayed in the ImageView change size based on random dimensions.
MainActivity.kt
package com.example.jetpack
import android.content.Context
import android.graphics.Bitmap
import android.graphics.BitmapFactory
import android.os.Bundle
import android.util.TypedValue
import android.widget.ImageView
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
import java.io.IOException
import kotlin.random.Random
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get bitmap from assets folder
val bitmap = assetsToBitmap("rose3.jpg")
// show bitmap on image view
bitmap?.apply {
imageView.setImageBitmap(this)
}
// button click to resize image view on random size
button.setOnClickListener {
// random width and height value in dp and convert to pixels
val newWidth = Random.nextInt(10,500)
.dpToPixels(applicationContext)
val newHeight = Random.nextInt(10,300)
.dpToPixels(applicationContext)
// resize image view
imageView.resize(newWidth,newHeight)
textView.text = "Resized ($newWidth px * $newHeight px)"
}
}
}
// extension function to resize image view programmatically
fun ImageView.resize(
newWidth: Int = layoutParams.width, // pixels
newHeight: Int = layoutParams.height // pixels
){
layoutParams.apply {
width = newWidth // in pixels
height = newHeight // in pixels
layoutParams = this
}
}
// extension function to get bitmap from assets
fun Context.assetsToBitmap(fileName: String): Bitmap?{
return try {
with(assets.open(fileName)){
BitmapFactory.decodeStream(this)
}
} catch (e: IOException) { null }
}
// extension function to convert dp to equivalent pixels
fun Int.dpToPixels(context: Context):Int = TypedValue.applyDimension(
TypedValue.COMPLEX_UNIT_DIP, this.toFloat(), context.resources.displayMetrics
).toInt()
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#EDEAE0"
tools:context=".MainActivity">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="280dp"
android:layout_marginStart="8dp"
android:layout_marginTop="32dp"
android:layout_marginEnd="8dp"
android:background="#E3DAC9"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/button"
tools:srcCompat="@tools:sample/avatars" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="Resize ImageView"
android:backgroundTint="#3B2F2F"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
tools:text="TextView"
android:textSize="25sp"
android:fontFamily="sans-serif-condensed-medium"
app:layout_constraintEnd_toEndOf="@+id/imageView"
app:layout_constraintStart_toStartOf="@+id/imageView"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
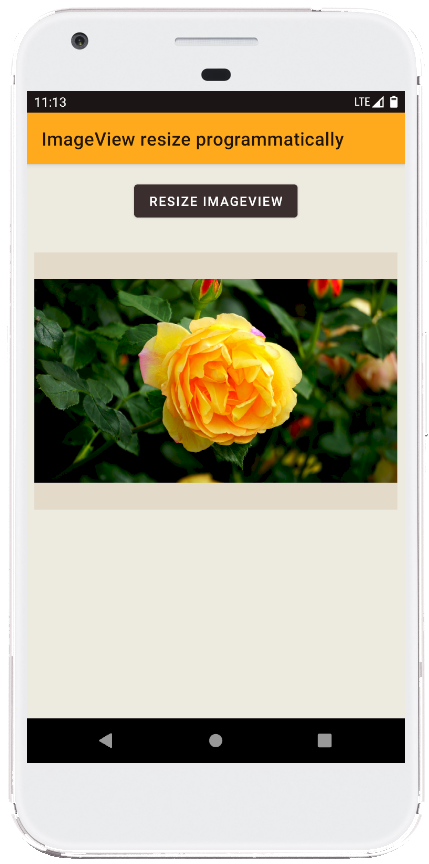
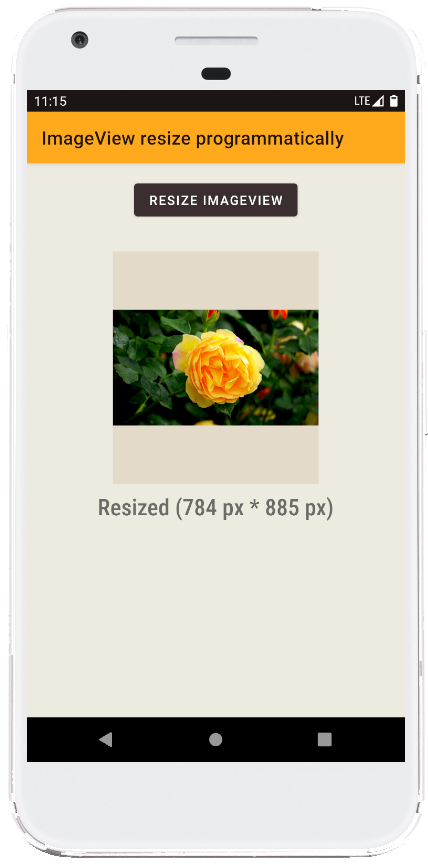
- android kotlin - EditText allow only numbers
- android kotlin - EditText allow only characters
- android kotlin - EditText limit number range
- android kotlin - EditText input filter decimal
- android kotlin - EditText remove underline while typing
- android kotlin - EditText change cursor color programmatically
- android kotlin - EditText hide keyboard after enter
- android kotlin - EditText hide keyboard click outside
- android kotlin - Switch button listener
- android kotlin - Material switch button
- android kotlin - Get raw resource uri
- android kotlin - Button with icon
- android kotlin - Canvas center text
- android kotlin - Canvas draw text
- android kotlin - Canvas draw circle