Android Kotlin - How to draw a text with shadow effect on a Canvas
This code demonstrates how to draw text with a shadow effect on an Android Canvas in Kotlin. It achieves this by creating a custom function drawTextShadow()
that generates a Bitmap with the desired text and shadow.
Breakdown:
Setting Up the Activity:
- The
MainActivity
class extendsActivity
and overrides theonCreate
method. - Inside
onCreate
, the code inflates the layout file (activity_main.xml
) and retrieves the reference to theImageView
usingfindViewById
.
- The
Drawing Text with Shadow:
- The
drawTextShadow()
function creates a newBitmap
with a specific size and configuration. - A
Canvas
is then created based on theBitmap
. - A
TextPaint
object is configured to define text properties like anti-aliasing, color, size, alignment, and typeface. - Importantly,
setShadowLayer
is called on theTextPaint
to define the shadow effect. This method takes parameters for the blur radius, offset in X and Y directions, and the shadow color. - The center coordinates of the canvas are calculated to position the text properly.
- Finally, the
drawText
method draws the text "ANDROID" on theCanvas
using the configuredTextPaint
.
- The
Displaying the Text:
- Back in
onCreate
, thedrawTextShadow
function is called and the returnedBitmap
is set as the image source for theImageView
.
- Back in
Layout:
- The
activity_main.xml
file defines a simple ConstraintLayout with anImageView
filling the available space.
- The
Summary:
This code provides a clear example of how to leverage the setShadowLayer
method of TextPaint
to create a text shadow effect on an Android Canvas. By customizing the various parameters within this method, you can control the appearance of the text shadow and achieve different visual styles.
MainActivity.kt
package com.cfsuman.kotlintutorials
import android.app.Activity
import android.graphics.*
import android.os.Bundle
import android.text.TextPaint
import android.widget.ImageView
class MainActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the widgets reference from XML layout
val imageView = findViewById<ImageView>(R.id.imageView)
// show drawing on image view
imageView.setImageBitmap(drawTextShadow())
}
}
// function to draw text shadow on canvas
fun drawTextShadow():Bitmap?{
val bitmap = Bitmap.createBitmap(
1400,
900,
Bitmap.Config.ARGB_8888
)
// canvas for drawing
val canvas = Canvas(bitmap).apply {
drawColor(Color.parseColor("#A2A2D0"))
}
// text paint to draw text
val textPaint = TextPaint().apply {
isAntiAlias = true
color = Color.parseColor("#333399")
textSize = 280F
textAlign = Paint.Align.CENTER
typeface = Typeface.DEFAULT_BOLD
// This draws a shadow layer below the main layer,
// with the specified offset and color, and blur radius.
setShadowLayer(
20F, // radius
5F, // dx
5F, // dy
Color.parseColor("#333399") // color
)
}
// calculate center position to draw text on canvas
val x = canvas.width / 2F
val y = (canvas.height /2 ) - (textPaint.descent()
+ textPaint.ascent()) / 2
// finally, draw text with shadow on canvas
canvas.drawText(
"ANDROID",
x,
y,
textPaint
)
return bitmap
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/rootLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#DCDCDC"
android:padding="24dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
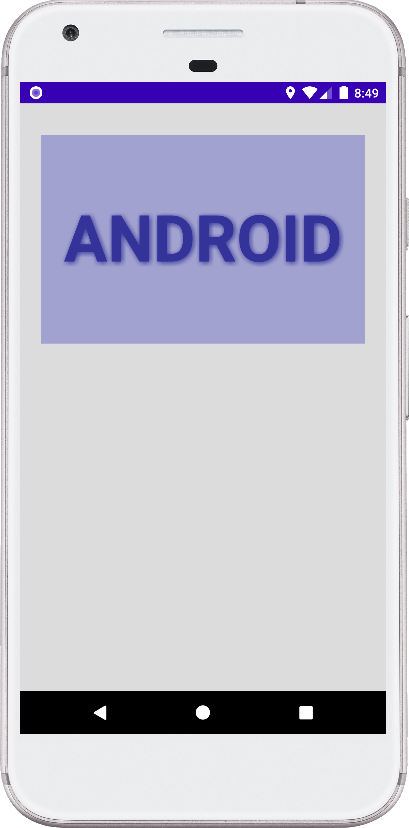
- android kotlin - Coroutines start undispatched
- android kotlin - Coroutines delay
- android kotlin - Canvas draw dotted line
- android kotlin - Canvas draw dashed line
- android kotlin - Canvas draw point
- android kotlin - Canvas erase drawing
- android kotlin - Canvas draw line
- android kotlin - Canvas draw arc between two points
- android kotlin - Canvas draw path
- android kotlin - Canvas draw text rotate
- android kotlin - Canvas draw text with border
- android kotlin - Canvas draw text in rectangle
- android kotlin - Canvas draw text inside circle
- android kotlin - Canvas draw text wrap
- android kotlin - Canvas draw circle