Customizing the Toolbar Back Button Color in Android (Kotlin)
This code demonstrates how to change the color of the back button displayed on the Toolbar in an Android application written in Kotlin. It includes two activities: a MainActivity and a SecondActivity.
The MainActivity serves as the starting point and displays a simple button to navigate to the SecondActivity. The SecondActivity showcases the back button customization.
Breakdown of the Code:
The code consists of several parts:
- Activity Classes (MainActivity.kt & SecondActivity.kt): These files define the functionalities of each activity. They handle setting up the layout, toolbar, and button click listener.
- Layout Resource Files (activity_main.xml & activity_second.xml): These files define the visual structure of each activity using ConstraintLayout and include elements like Toolbar and Button.
- Styles (res/values/styles.xml): This file defines the base theme for the application, including color attributes like
colorPrimary
. - Android Manifest (AndroidManifest.xml): This file specifies important app information and configures the activities, including setting the MainActivity as the launcher activity.
The key logic for customizing the back button color resides in the SecondActivity. Here's what happens:
- Setting Up the Toolbar: The code inflates the layout and sets the toolbar as the support action bar. It also sets the title for the toolbar.
- Enabling Back Button:
setDisplayHomeAsUpEnabled
andsetDisplayShowHomeEnabled
are used to enable the back button on the toolbar and allow it to navigate back to the parent activity (MainActivity). - Changing Back Button Color: The code retrieves the navigation icon (back button) from the toolbar and applies a color filter to it. Depending on the Android version, either
setColorFilter
orBlendModeColorFilter
is used with the desired color (red in this example) and theSRC_IN
blend mode.
This approach allows you to dynamically change the back button color in your application.
MainActivity.kt
package com.example.jetpack
import android.content.Intent
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_main.*
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// set toolbar as support action bar
setSupportActionBar(toolbar).apply {
title = "Kotlin - Toolbar Back Button Color"
}
// navigate to second activity
button.setOnClickListener {
val intent = Intent(this,SecondActivity::class.java)
startActivity(intent)
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#A5B1A6"
tools:context=".MainActivity">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary"
android:minHeight="?attr/actionBarSize"
android:theme="?attr/actionBarTheme"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
android:elevation="4dp"
app:layout_constraintTop_toTopOf="parent" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Go To Second Activity"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/toolbar" />
</androidx.constraintlayout.widget.ConstraintLayout>
SecondActivity.kt
package com.example.jetpack
import android.graphics.*
import android.os.Build
import android.os.Bundle
import androidx.appcompat.app.AppCompatActivity
import kotlinx.android.synthetic.main.activity_second.*
class SecondActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_second)
// set toolbar as support action bar
setSupportActionBar(toolbar)
supportActionBar?.apply {
title = "Toolbar Back Button Color"
// show back button on toolbar
// on back button press, it will navigate to parent activity
setDisplayHomeAsUpEnabled(true)
setDisplayShowHomeEnabled(true)
}
// change the back button color
toolbar.navigationIcon?.apply {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.Q) {
colorFilter = BlendModeColorFilter(Color.RED,BlendMode.SRC_IN)
}else{
setColorFilter(Color.RED,PorterDuff.Mode.SRC_IN)
}
}
}
}
activity_second.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#B0CAB2"
tools:context=".SecondActivity">
<androidx.appcompat.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimary"
android:minHeight="?attr/actionBarSize"
android:theme="?attr/actionBarTheme"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
android:elevation="4dp"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Second Activity"
style="@style/TextAppearance.MaterialComponents.Headline6"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
res/values/styles.xml
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.MaterialComponents.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest
xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.jetpack">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".SecondActivity"
android:parentActivityName=".MainActivity">
</activity>
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
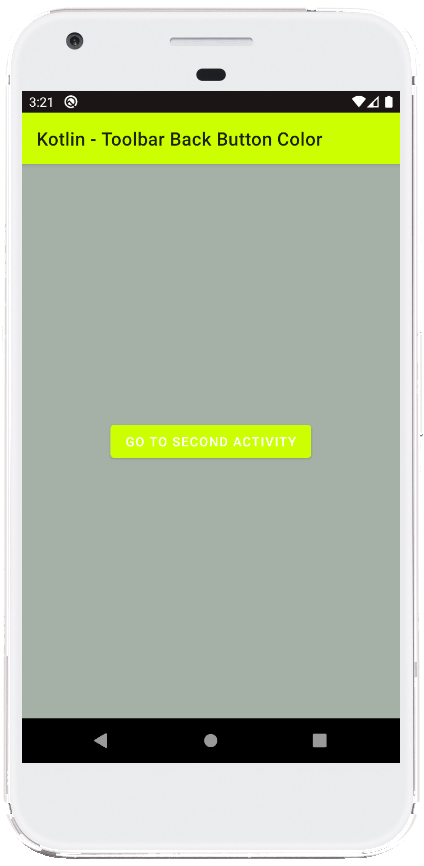
