Displaying a Popup Window
Today we will learn how to display a Popup window in your android application user interface. A popup window is used to display an arbitrary view that can hold your specified widgets and texts. Android Popup window is a floating container and it appears on top of the current activity. We can populate a Popup window by using android SDK’s PopupWindow class. This class has many useful methods. Those will help us to generate a beautiful Popup window.
Ok, now let’s describe our code snippets. How do we display a Popup window in our android application? First, we create an activity XML file and put a Button widget. That button click event performs the essential task to generate a Popup window on top of the current activity.
We create another XML custom layout file that holds the widgets of the Popup window. Simply put an ImageButton and a TextView widget on it. The image button is used to show a close button to dismiss the Popup window and the text widget display a simple message. In this custom layout file, we used a RelativeLayout to wrap the widget.
Now, let’s describe the actual coding on the Java file. On the button click event, we generate and display the Popup window on top of the current activity. We inflate the custom XML layout file using layout inflater. Then we used this custom layout to generate a new PopupWindow class instance. Next, we set an elevation of the Popup window, so that it will get a material design look.
Third, we define the Popup window close/dismiss button. To do this we choose the custom layout’s image button. On the image button click event, we call the dismiss method to close the Popup window. Finally, we specify the activity location where we want to show our newly created Popup window. Here we display the Popup window at the center position of the current activity. The following code snippets and output images will help you to understand the full sense more efficiently.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/rl"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".MainActivity"
android:background="#f5f1e0"
>
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Popup Window"
/>
</RelativeLayout>
res/layout/custom_layout.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/rl_custom_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="2dp"
android:background="#ab2fc4"
>
<ImageButton
android:id="@+id/ib_close"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_close_white_24dp"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:background="@null"
/>
<TextView
android:id="@+id/tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is a sample popup window."
android:layout_centerInParent="true"
android:padding="25sp"
/>
</RelativeLayout>
MainActivity.java
package com.cfsuman.me.androidcodesnippets;
import android.app.Activity;
import android.content.Context;
import android.os.Build;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.ImageButton;
import android.widget.PopupWindow;
import android.widget.RelativeLayout;
import android.view.ViewGroup.LayoutParams;
public class MainActivity extends AppCompatActivity {
private Context mContext;
private Activity mActivity;
private RelativeLayout mRelativeLayout;
private Button mButton;
private PopupWindow mPopupWindow;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the application context
mContext = getApplicationContext();
// Get the activity
mActivity = MainActivity.this;
// Get the widgets reference from XML layout
mRelativeLayout = (RelativeLayout) findViewById(R.id.rl);
mButton = (Button) findViewById(R.id.btn);
// Set a click listener for the text view
mButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// Initialize a new instance of LayoutInflater service
LayoutInflater inflater = (LayoutInflater) mContext.getSystemService(LAYOUT_INFLATER_SERVICE);
// Inflate the custom layout/view
View customView = inflater.inflate(R.layout.custom_layout,null);
/*
public PopupWindow (View contentView, int width, int height)
Create a new non focusable popup window which can display the contentView.
The dimension of the window must be passed to this constructor.
The popup does not provide any background. This should be handled by
the content view.
Parameters
contentView : the popup's content
width : the popup's width
height : the popup's height
*/
// Initialize a new instance of popup window
mPopupWindow = new PopupWindow(
customView,
LayoutParams.WRAP_CONTENT,
LayoutParams.WRAP_CONTENT
);
// Set an elevation value for popup window
// Call requires API level 21
if(Build.VERSION.SDK_INT>=21){
mPopupWindow.setElevation(5.0f);
}
// Get a reference for the custom view close button
ImageButton closeButton = (ImageButton) customView.findViewById(R.id.ib_close);
// Set a click listener for the popup window close button
closeButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// Dismiss the popup window
mPopupWindow.dismiss();
}
});
/*
public void showAtLocation (View parent, int gravity, int x, int y)
Display the content view in a popup window at the specified location. If the
popup window cannot fit on screen, it will be clipped.
Learn WindowManager.LayoutParams for more information on how gravity and the x
and y parameters are related. Specifying a gravity of NO_GRAVITY is similar
to specifying Gravity.LEFT | Gravity.TOP.
Parameters
parent : a parent view to get the getWindowToken() token from
gravity : the gravity which controls the placement of the popup window
x : the popup's x location offset
y : the popup's y location offset
*/
// Finally, show the popup window at the center location of root relative layout
mPopupWindow.showAtLocation(mRelativeLayout, Gravity.CENTER,0,0);
}
});
}
}
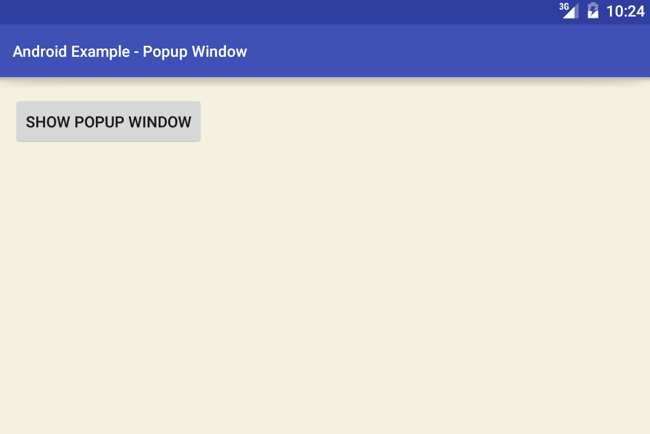
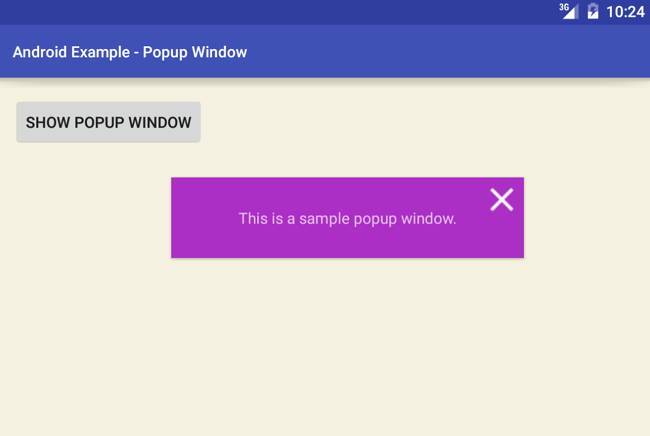