Introduction
In web development, managing server-side file systems efficiently is an essential task. Adobe ColdFusion offers several useful tags and functions to interact with files and directories, including the ability to programmatically create, modify, and delete directories. In this tutorial, we will explore how to delete a directory using the ColdFusion <cfdirectory>
tag. This functionality is particularly useful for developers needing to automate file system management tasks, such as cleaning up unused directories or managing temporary files generated by web applications.
This example demonstrates how to check if a directory exists on the server and, if it does, delete it using ColdFusion's native tags. We will walk through the structure and logic of the code, providing clear explanations of how it works and how you can apply it to similar scenarios.
Displaying the Current Directory
The code starts by setting the path of the directory we want to work with using the cfset
tag. In this case, the path C:\TestDirectory
is stored in the CurrentDirectory
variable. This path represents the directory we intend to check and potentially delete.
The use of the <cfoutput>
tag helps in dynamically displaying the directory path on the webpage. This step is useful for debugging purposes, as it allows you to see which directory is currently being targeted before any actions are taken. When running the script, it will output a line like "Current Directory: C:\TestDirectory", which gives the user immediate feedback on the directory's location.
Checking if the Directory Exists
Before attempting to delete the directory, it's essential to ensure that it exists. ColdFusion's DirectoryExists()
function is used here to check whether the specified directory is present on the server. This function returns a Boolean value—true
if the directory exists, and false
otherwise.
In the code, the existence of the directory is evaluated inside a <cfif>
conditional block. If the directory is found, the code proceeds to delete it. If it does not exist, a message is output stating that the directory does not exist. This step ensures that unnecessary deletion attempts are avoided, preventing potential errors or performance issues.
Deleting the Directory
The key part of this example is the <cfdirectory>
tag, which is used to perform actions on directories. In this case, the action specified is "delete"
, which instructs ColdFusion to remove the directory at the path specified by the directory
attribute. The directory path is dynamically passed to the tag using #CurrentDirectory#
, ensuring that the directory specified earlier is deleted if it exists.
Once the directory is successfully deleted, a confirmation message ("Directory deleted!") is displayed to the user. This feedback is critical for informing the user that the operation has been completed without any issues.
Handling Non-Existent Directories
In the event that the directory does not exist, the code enters the <cfelse>
block, where it outputs a message stating that the specified directory does not exist. This is a graceful way of handling scenarios where the deletion action is unnecessary, and it prevents errors that could arise from trying to delete something that isn't there.
Conclusion
This tutorial showcases how ColdFusion's <cfdirectory>
tag can be leveraged to manage server-side directories efficiently. By checking if a directory exists before attempting to delete it, we can avoid unnecessary operations and potential errors. This process is simple yet powerful for automating file system tasks in web applications.
With the ability to delete directories programmatically, developers have greater control over the file systems their applications interact with. Whether it’s for routine maintenance, cleanup, or other file management tasks, understanding how to use the <cfdirectory>
tag is an essential skill for ColdFusion developers.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>ColdFusion cfdirectory tag example: how to delete directory programmatically</title>
</head>
<body>
<h2 style="color:DodgerBlue">ColdFusion cfdirectory tag example: Delete</h2>
<cfset CurrentDirectory="C:\TestDirectory">
<cfoutput>
<b>Current Directory:</b> #CurrentDirectory#
<br />
</cfoutput>
<cfif DirectoryExists(CurrentDirectory)>
<cfdirectory action="delete" directory="#CurrentDirectory#">
<i>Directory deleted!</i>
<cfelse>
<cfoutput>
#CurrentDirectory# Directory not exists!
</cfoutput>
</cfif>
</body>
</html>
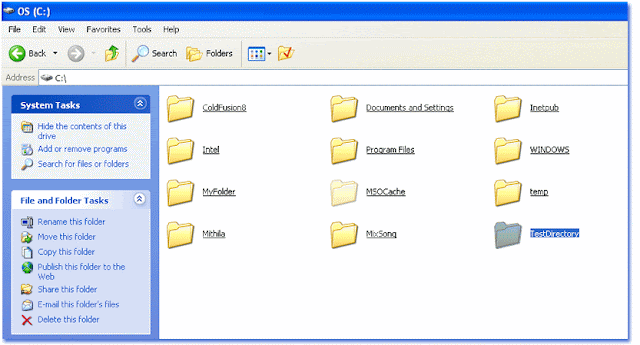
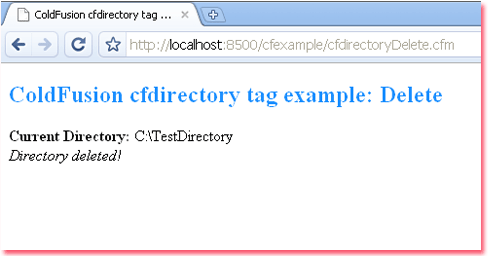
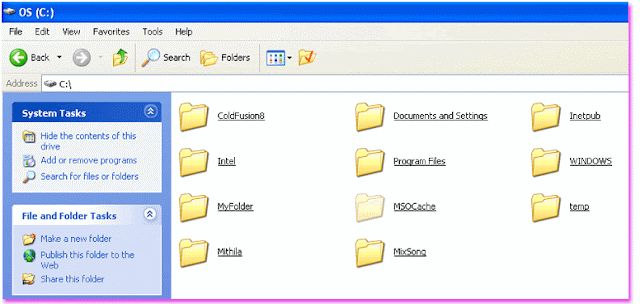