cfdirectory - create directory programmatically
cfdirectoryCreate.cfm
<!DOCTYPE html">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>ColdFusion cfdirectory tag example: how to create directory programmatically</title>
</head>
<body>
<h2 style="color:DodgerBlue">ColdFusion cfdirectory tag example: Create</h2>
<cfset CurrentDirectory=GetTemplatePath()>
<cfset CurrentDirectory=ListDeleteAt(CurrentDirectory,ListLen(CurrentDirectory,"/\"),"/\")>
<cfset NewDirectory="#CurrentDirectory#\TestFolder">
<cfoutput>
<b>Current Directory:</b> #CurrentDirectory#
<br />
<b>New Directory:</b> #NewDirectory#
</cfoutput>
<cfdirectory action="create" directory="#NewDirectory#">
</body>
</html>
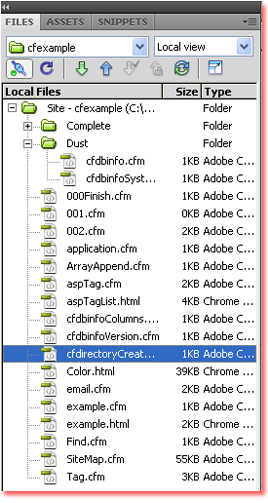
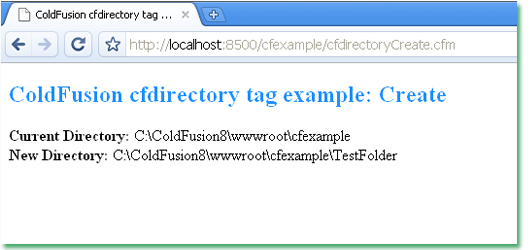
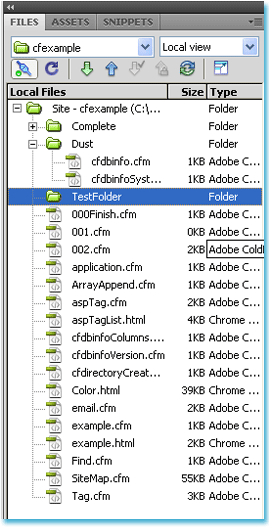